Open Interpreter: Local Code Execution with LLMs
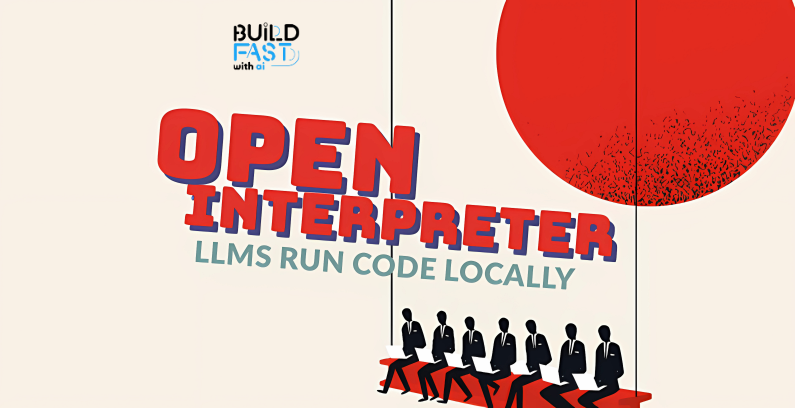
How do you become an AI innovator? Start with the right guide, and this is your first step.
Join Build Fast with AI’s Gen AI Launch Pad 2025—a 6-week program designed to empower you with the tools and skills to lead in AI innovation.
Introduction
In recent years, Large Language Models (LLMs) like OpenAI GPT have evolved beyond generating text to performing dynamic tasks such as executing code locally. This transformative capability enables developers to streamline workflows, automate coding tasks, and validate outputs in real time. In this blog, we explore and demonstrates the power of LLMs in local code execution. You will learn how to:
- Set up and interact with an LLM to execute code locally.
- Generate and refine code snippets dynamically.
- Validate the correctness of model-generated code through local execution.
- Implement error handling and debugging strategies effectively.
- Utilize LLMs for managing files, creating documents, and editing content programmatically.
By the end of this guide, you will understand how to leverage LLMs for enhanced productivity in coding tasks, along with practical examples and actionable insights.
Setup and Installation
Code Snippet
!pip install open-interpreter
Explanation
The first step is to install the open-interpreter
library, an open-source framework that integrates with LLMs to provide local code execution capabilities. This installation ensures that the necessary tools and dependencies are available for subsequent interactions.
Expected Output
Upon successful execution, you should see output confirming the installation of the library and its dependencies. For example:
Collecting open-interpreter ... Successfully installed open-interpreter
Real-World Application
- Streamlined Setup: Quickly integrate local code execution tools into your workflow.
- Exploratory Programming: Use the installed framework to test new ideas or learn programming concepts interactively.
Setting Up the API Key
Code Snippet
from interpreter import interpreter from google.colab import userdata import os os.environ['OPENAI_API_KEY'] = userdata.get('OPENAI_API_KEY') OPENAI_API = os.getenv('OPENAI_API_KEY') # Paste your OpenAI API key below. interpreter.llm.api_key = OPENAI_API
Explanation
This block initializes the environment and securely sets the API key for interacting with OpenAI's LLMs. Here’s what it does:
- Import Libraries: Imports the
interpreter
module and Colab’suserdata
functionality for accessing secure data. - Environment Variables: Uses
os
to set and retrieve the OpenAI API key from environment variables, ensuring security. - API Key Assignment: Configures the interpreter with the API key to enable communication with OpenAI's servers.
Expected Output
There is no direct output from this block. However, successful execution ensures that the API key is correctly configured.
Real-World Application
- Secure API Usage: Ensures sensitive credentials are not exposed in code.
- Foundation for Interaction: Sets up the necessary environment for seamless integration with LLMs.
Printing 'Hello, World!'
Code Snippet
interpreter.chat("Please print hello world.")
Explanation
This command demonstrates the interpreter’s capability to execute conversational commands. By providing a natural language prompt, the LLM interprets and executes the corresponding Python command.
Expected Output
Hello, World!
Key Takeaways
- Natural Language Processing: Allows users to interact with programming environments conversationally.
- Simple Tasks: Demonstrates the ease of performing basic tasks through LLMs.
Real-World Application
- Education: Introducing beginners to programming concepts interactively.
- Automation: Handling routine tasks via natural language commands.
Creating and Editing Documents
Code Snippet
interpreter.messages = [] # Reset the chat interpreter.chat("""Can you make a folder called documents and put five .docx files in it and write a sentence about machine learning in each of them?""")
Explanation
This block showcases the interpreter’s ability to manage files and content programmatically. Here’s what it does:
- Reset Memory: Clears previous chat history to ensure a fresh context.
- Create Folder and Files: Uses a natural language prompt to create a folder (
documents
) and populate it with.docx
files containing sentences about machine learning.
Expected Output
- A folder named
documents
containing five.docx
files. - Each file includes a sentence about machine learning, such as:
Machine learning is a subset of artificial intelligence.
Real-World Application
- Content Automation: Generate bulk content efficiently.
- File Organization: Automate folder and file creation for structured data storage.
Editing Documents Programmatically
Code Snippet
interpreter.messages = [] interpreter.chat("""Go through all the .docx files in my 'documents' folder and replace every occurrence of 'Machine Learning' with 'AI'.""")
Explanation
This block modifies the content of the previously created .docx
files. By providing a conversational command, the LLM executes the required text replacement.
Expected Output
Updated .docx
files where every occurrence of "Machine Learning" is replaced with "AI." For example:
AI is a subset of artificial intelligence.
Real-World Application
- Editing at Scale: Modify large batches of documents programmatically.
- Consistency Checks: Ensure uniform terminology across multiple files.
Resetting Memory
Code Snippet
interpreter.messages = []
Explanation
This command clears the interpreter’s memory, resetting the context for subsequent interactions. It ensures that previous commands or data do not interfere with new tasks.
Expected Output
No visible output. Successful execution ensures a clean slate for the next operation.
Real-World Application
- Session Management: Start fresh without residual data from prior tasks.
- Context-Specific Workflows: Maintain clarity when switching between unrelated projects.
Conclusion
We explored the integration of LLMs for local code execution, showcasing their ability to:
- Generate high-quality, functional code from natural language prompts.
- Validate and refine code snippets through local execution.
- Handle errors and edge cases effectively using robust debugging techniques.
- Manage files and automate content creation programmatically.
These techniques empower developers to work more efficiently, educators to deliver interactive lessons, and researchers to prototype ideas rapidly. By incorporating LLMs into your workflow, you can unlock new levels of productivity and innovation.
As the capabilities of LLMs continue to evolve, their applications in coding and software development are poised to grow, opening new possibilities for automation, creativity, and learning.
Resources
For further reading and exploration, consider the following resources:
- Open Interpreter GitHub Repository
- LangChain Documentation
- OpenAI API Reference
- Jupyter Notebook Documentation
- Open Interpreter Build Fast With AI NoteBook
---------------------------------
Stay Updated:- Follow Build Fast with AI pages for all the latest AI updates and resources.
Experts predict 2025 will be the defining year for Gen AI implementation.Want to be ahead of the curve?
Join Build Fast with AI’s Gen AI Launch Pad 2025 - your accelerated path to mastering AI tools and building revolutionary applications.