Serverless PostgreSQL & AI: NeonDB with pgvector
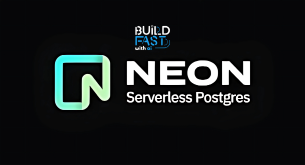
Do you want to be remembered as someone who waited or someone who created?
Gen AI Launch Pad 2025 is your platform to innovate.
Introduction
In the world of AI-driven applications, database performance and scalability are crucial. Neon is an open-source, serverless PostgreSQL database that introduces cutting-edge features like autoscaling, branching, and vector support (pgvector). This makes it an ideal choice for AI workloads, real-time analytics, and scalable cloud applications.
In this blog, we’ll explore how to leverage Neon’s features to create scalable applications. We’ll walk through:
- Setting up a serverless PostgreSQL database.
- Running vector similarity searches with pgvector.
- Creating an interactive to-do list application.
- Implementing an AI chatbot with OpenAI and NeonDB.
Getting Started with Neon PostgreSQL
Before diving into code, let's take a quick look at Neon’s key features:
- Open Source: Transparent and free to use.
- Serverless: No infrastructure management required.
- Autoscaling: Automatically adjusts resources based on demand.
- Branching: Clone databases instantly for testing and development.
- Vector Search (pgvector): Optimized for AI and embedding-based searches.
- Fully PostgreSQL-Compatible: Works with all PostgreSQL tools and extensions.
Step 1: Connect to Neon PostgreSQL
First, let’s connect to a Neon database using Python and psycopg2.
import os import psycopg2 from google.colab import userdata connection_string = userdata.get('NEON_URL') connection = psycopg2.connect(connection_string) cursor = connection.cursor()
Explanation:
- We retrieve the database URL from Google Colab’s
userdata
. - Establish a connection to the database.
- Create a cursor to execute SQL queries.
Step 2: Test the Database Connection
Before proceeding, let’s ensure our database connection is successful:
cursor.execute("SELECT 1;") result = cursor.fetchone() if result == (1,): print("Your database connection was successful!") else: print("Your connection failed.")
Explanation:
- Executes a simple
SELECT 1;
query to test the connection. - If the query returns
(1,)
, the connection is successful.
Expected Output:
Your database connection was successful!
Implementing Vector Search with pgvector
Step 3: Install and Set Up pgvector
To perform vector similarity searches, we first need to install the pgvector
extension in Neon.
cursor.execute("CREATE EXTENSION IF NOT EXISTS vector;")
Explanation:
- Ensures that the pgvector extension is installed and available in the database.
Step 4: Create a Table and Insert Vector Data
Let’s create a table that stores vector embeddings:
create_table_sql = ''' CREATE TABLE items ( id BIGSERIAL PRIMARY KEY, embedding VECTOR(3) ); ''' insert_data_sql = ''' INSERT INTO items (embedding) VALUES ('[1,2,3]'), ('[4,5,6]'), ('[7,8,9]'); ''' cursor.execute(create_table_sql) cursor.execute(insert_data_sql) connection.commit()
Explanation:
- Creates a table
items
with aBIGSERIAL
primary key and a 3-dimensional vector column. - Inserts vector data to be used for similarity searches.
Step 5: Perform a Vector Similarity Search
cursor.execute("SELECT * FROM items ORDER BY embedding <-> '[3,1,2]' LIMIT 3;") all_data = cursor.fetchall() print(all_data)
Explanation:
- Uses the <-> operator to compute similarity between vectors.
- Retrieves the top 3 nearest vectors to
[3,1,2]
.
Expected Output:
[(1, '[1,2,3]'), (2, '[4,5,6]'), (3, '[7,8,9]')]
Building an Interactive To-Do List App
Step 6: Create the To-Do List Table
cursor.execute(""" CREATE TABLE IF NOT EXISTS tasks ( id SERIAL PRIMARY KEY, task TEXT NOT NULL, completed BOOLEAN DEFAULT FALSE ) """) conn.commit()
Explanation:
- Creates a
tasks
table with anid
,task description
, and acompleted
status.
Step 7: Add a New Task
def add_task(task): cursor.execute("INSERT INTO tasks (task) VALUES (%s)", (task,)) conn.commit() print("✅ Task added!")
Explanation:
- Inserts a new task into the
tasks
table. - Commits the transaction to save the task.
Step 8: View All Tasks
def view_tasks(): cursor.execute("SELECT id, task, completed FROM tasks") tasks = cursor.fetchall() print("\n📋 To-Do List:") for task in tasks: status = "✅" if task[2] else "❌" print(f"{task[0]}. {task[1]} {status}")
Explanation:
- Retrieves all tasks from the
tasks
table. - Displays each task along with its completion status.
Step 9: Delete a Task
def delete_task(task_id): cursor.execute("DELETE FROM tasks WHERE id = %s", (task_id,)) conn.commit() print("🗑️ Task deleted!")
Explanation:
- Deletes a task by its
id
from the database. - Commits the deletion to update the database.
OpenAI Chatbot with NeonDB
Step 10: Store Chat History in NeonDB
cursor.execute(""" CREATE TABLE IF NOT EXISTS chat_history ( id SERIAL PRIMARY KEY, user_message TEXT NOT NULL, bot_response TEXT NOT NULL, created_at TIMESTAMP DEFAULT CURRENT_TIMESTAMP ) """)
Explanation:
- Creates a
chat_history
table to store user queries and chatbot responses.
Conclusion
NeonDB provides a powerful, scalable, and serverless PostgreSQL experience, making it perfect for AI applications. Whether you’re implementing vector search, task management, or AI chatbots, Neon simplifies the process.
Resources
---------------------------
Stay Updated:- Follow Build Fast with AI pages for all the latest AI updates and resources.
Experts predict 2025 will be the defining year for Gen AI Implementation. Want to be ahead of the curve?
Join Build Fast with AI’s Gen AI Launch Pad 2025 - your accelerated path to mastering AI tools and building revolutionary applications.
---------------------------
Resources and Community
Join our community of 12,000+ AI enthusiasts and learn to build powerful AI applications! Whether you're a beginner or an experienced developer, this tutorial will help you understand and implement AI agents in your projects.
- Website: www.buildfastwithai.com
- LinkedIn: linkedin.com/company/build-fast-with-ai/
- Instagram: instagram.com/buildfastwithai/
- Twitter: x.com/satvikps
- Telegram: t.me/BuildFastWithAI