Tiktoken: High-Performance Tokenizer for OpenAI Models
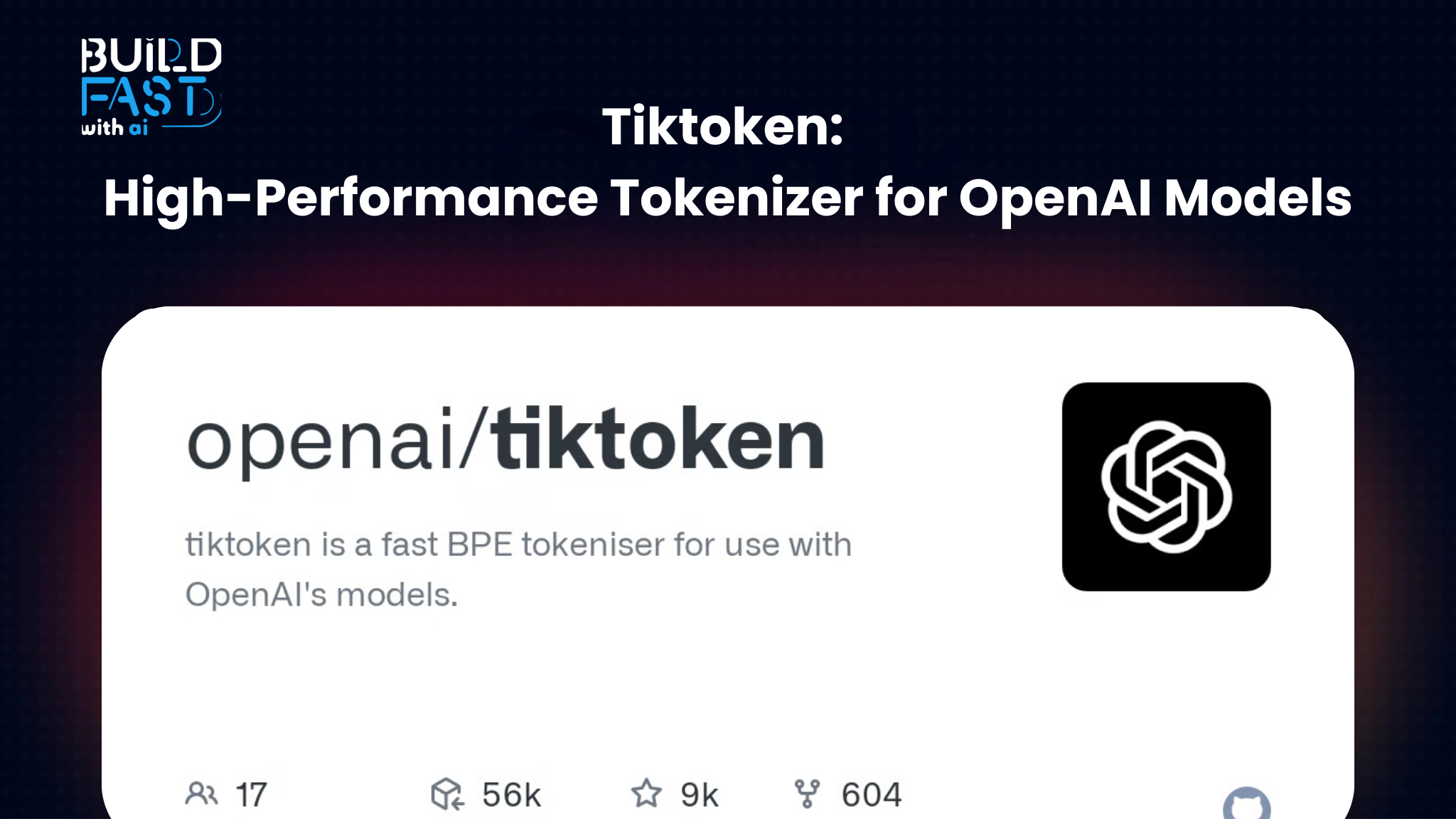
Are you going to let the future happen to you, or will you happen to the future?
Gen AI Launch Pad 2025 is your moment.
Introduction
When it comes to working with advanced natural language models like OpenAI's GPT series, one of the most critical processes is tokenization. Tokenization involves breaking down text into smaller, manageable pieces (tokens) that the model can understand and process. The efficiency and effectiveness of tokenization directly affect model performance and the costs associated with using these models.
In this blog post, we'll dive into Tiktoken, an open-source tokenizer developed by OpenAI that significantly improves the speed and accuracy of tokenization. Whether you’re a data scientist, software engineer, or AI enthusiast, understanding how to use Tiktoken will empower you to optimize text processing in your projects and unlock the full potential of OpenAI models.
We'll explore how Tiktoken works, walk through code examples, and explain how you can use it in real-world applications. Let’s get started!
What is Tokenization?
Tokenization is the process of breaking down text into units, known as tokens, that a model can process. These tokens can represent words, characters, or even parts of words. Tokenization is essential because large models like GPT-3 and GPT-4 work with these tokens instead of raw text. Models are trained to predict the next token in a sequence, making tokenization a key step in natural language understanding.
For example, the sentence "Tiktoken is amazing!" might be broken down into tokens like:
- "Tiktoken"
- "is"
- "amazing"
- "!"
But the way these tokens are represented internally (as numbers or byte sequences) can vary depending on the tokenizer being used.
Tiktoken is a high-performance library designed to efficiently tokenize text for OpenAI models. It’s optimized for speed and resource efficiency, which is crucial for large-scale applications or real-time processing.
Setting Up Tiktoken: Installation and Initial Setup
The first step to using Tiktoken in your projects is to install it. If you’re using Google Colab or a similar environment, you can simply install Tiktoken using pip.
Code:
pip install tiktoken from google.colab import userdata OPENAI_API_KEY = userdata.get('OPENAI_API_KEY') import tiktoken enc = tiktoken.get_encoding("o200k_base") assert enc.decode(enc.encode("hello world")) == "hello world"
Explanation:
- pip install tiktoken: This command installs the Tiktoken library from PyPI (Python Package Index), so you can use it in your project.
- from google.colab import userdata: This line is specific to Google Colab users and is used to retrieve your OpenAI API key securely.
- tiktoken.get_encoding("o200k_base"): This loads the encoding configuration for a specific model. The encoding configuration defines how text is tokenized. In this case, we're using
o200k_base
, which is optimized for a certain model type. - assert enc.decode(enc.encode("hello world")) == "hello world": This ensures that encoding and decoding are working correctly. The string "hello world" is first encoded into tokens, and then decoded back to the original string to verify correctness.
Expected Output:
After running the setup, the code should execute without any errors, confirming that Tiktoken is properly installed and functioning. If there were any issues, an error message would appear.
Real-World Application:
This setup is fundamental for using Tiktoken in real-world applications, such as preparing text for GPT-3 or GPT-4 model API calls. It's especially useful in scenarios where large datasets need to be preprocessed before being fed into the model, ensuring that tokenization is efficient and error-free.
Encoding and Decoding Text with Tiktoken
Once you've installed Tiktoken, the next step is understanding how to encode and decode text. In this section, we’ll explore the core functionality of Tiktoken: tokenizing and detokenizing text.
Code:
encoding = tiktoken.get_encoding("cl100k_base") encoding.encode("tiktoken is great!") # Expected output: [83, 8251, 2488, 382, 2212, 0] encoding.decode([83, 8251, 2488, 382, 2212, 0]) # Expected output: 'tiktoken is great!' [encoding.decode_single_token_bytes(token) for token in [83, 8251, 2488, 382, 2212, 0]] # Expected output: [b't', b'ikt', b'oken', b' is', b' great', b'!']
Explanation:
- encoding.encode("tiktoken is great!"): This method takes a string and converts it into a list of token integers. The string "tiktoken is great!" is transformed into tokens represented by integer values that the model can process.
- encoding.decode([83, 8251, 2488, 382, 2212, 0]): This method decodes the list of token integers back into the original string. The list of token integers corresponds to the text "tiktoken is great!".
- encoding.decode_single_token_bytes: This function decodes individual tokens into byte representations. This allows us to see how Tiktoken splits a string into its smallest components.
Expected Output:
- Encoding:
[83, 8251, 2488, 382, 2212, 0]
– This represents the tokenized form of "tiktoken is great!".
- Decoding:
'tiktoken is great!'
– This is the original string after decoding the tokens.
- Byte-Level Decoding:
[b't', b'ikt', b'oken', b' is', b' great', b'!']
– These byte sequences represent the breakdown of the original text into smaller, byte-level components.
Real-World Application:
Understanding the encoding and decoding process is essential for applications that involve generating or analyzing text with large language models. For example, if you're building a chat application or a content generation tool, understanding tokenization helps you better manage token limits, optimize API usage, and ensure the output matches your expectations.
Comparing Different Encodings
Tiktoken supports several different encoding schemes, each optimized for different model types. In this section, we’ll compare how different encodings handle the same text, helping you understand their behavior and choose the right one for your application.
Code:
def compare_encodings(example_string: str) -> None: """Prints a comparison of four string encodings.""" print(f'\nExample string: "{example_string}"') for encoding_name in ["r50k_base", "p50k_base", "cl100k_base", "o200k_base"]: encoding = tiktoken.get_encoding(encoding_name) token_integers = encoding.encode(example_string) num_tokens = len(token_integers) token_bytes = [encoding.decode_single_token_bytes(token) for token in token_integers] print() print(f"{encoding_name}: {num_tokens} tokens") print(f"token integers: {token_integers}") print(f"token bytes: {token_bytes}") compare_encodings("antidisestablishmentarianism")
Explanation:
In this function, we compare four different encodings using the string "antidisestablishmentarianism":
r50k_base
p50k_base
cl100k_base
o200k_base
For each encoding, the function:
- Encodes the string into tokens.
- Counts the number of tokens.
- Prints the list of token integers.
- Displays the byte representation of each token.
Expected Output:
The output will vary depending on the encoding used. Here's a sample output for this string:
Example string: "antidisestablishmentarianism" r50k_base: 5 tokens token integers: [415, 29207, 44390, 3699, 1042] token bytes: [b'ant', b'idis', b'establishment', b'arian', b'ism'] p50k_base: 5 tokens token integers: [415, 29207, 44390, 3699, 1042] token bytes: [b'ant', b'idis', b'establishment', b'arian', b'ism'] cl100k_base: 6 tokens token integers: [519, 85342, 34500, 479, 8997, 2191] token bytes: [b'ant', b'idis', b'establish', b'ment', b'arian', b'ism'] o200k_base: 6 tokens token integers: [493, 129901, 376, 160388, 21203, 2367] token bytes: [b'ant', b'idis', b'est', b'ablishment', b'arian', b'ism']
Real-World Application:
Choosing the right encoding depends on the model you're using and the specific needs of your application. For example, if you're processing short texts like user messages in a chatbot, r50k_base
might be sufficient. For longer, more complex texts, you might prefer a more detailed encoding like cl100k_base
or o200k_base
.
Counting Tokens in Chat Completions
Finally, we’ll explore how to count the tokens used in a set of messages. This is essential for managing API usage, as models like GPT-4 have token limits that determine how much text can be sent in a single request.
Code:
def num_tokens_from_messages(messages, model="gpt-4o-mini-2024-07-18"): """Returns the number of tokens used by a list of messages.""" # logic to count tokens... return num_tokens num_tokens_from_messages(example_messages, "gpt-4o-mini")
Explanation:
This function takes a list of messages and returns the total number of tokens used. It’s useful when interacting with the OpenAI API, where token usage is billed. Knowing how many tokens you’ve used helps you stay within API limits and manage costs effectively.
Expected Output:
The function will return the number of tokens used by the provided set of messages.
Real-World Application:
Understanding token usage is crucial for managing costs when using large models. If you're building a chatbot or virtual assistant, you can optimize interactions by keeping track of token usage and adjusting the conversation flow accordingly.
Conclusion
In this post, we’ve explored how Tiktoken streamlines the tokenization process for OpenAI models. From setting up the library and encoding/decoding text, to comparing different encodings and counting tokens for chat completions, Tiktoken provides a powerful toolkit for working with text in AI applications.
Key Takeaways:
- Tiktoken is optimized for speed and efficiency, making it a great choice for large-scale AI applications.
- Tokenization is a crucial part of working with language models, and understanding how different encodings work can help you optimize text processing.
- By tracking token usage, you can manage costs and stay within API limits.
Next Steps:
- Experiment with Tiktoken in your own projects, such as chatbots or content generation tools.
- Dive deeper into the Tiktoken documentation to understand more advanced features and use cases.
Resources Section
- Tiktoken GitHub Repository
- OpenAI Documentation
- Byte Pair Encoding Explanation
- OpenAI GPT Models Overview
- Tiktoken Experiment Notebook
---------------------------
Stay Updated:- Follow Build Fast with AI pages for all the latest AI updates and resources.
Experts predict 2025 will be the defining year for Gen AI implementation.Want to be ahead of the curve?
Join Build Fast with AI’s Gen AI Launch Pad 2025 - your accelerated path to mastering AI tools and building revolutionary applications.
---------------------------
Resources and Community
Join our community of 12,000+ AI enthusiasts and learn to build powerful AI applications! Whether you're a beginner or an experienced developer, this tutorial will help you understand and implement AI agents in your projects.
- Website: www.buildfastwithai.com
- LinkedIn: linkedin.com/company/build-fast-with-ai/
- Instagram: instagram.com/buildfastwithai/
- Twitter: x.com/satvikps
- Telegram: t.me/BuildFastWithAI