Mastering AI Orchestration with Semantic Kernel: A Hands-On Guide
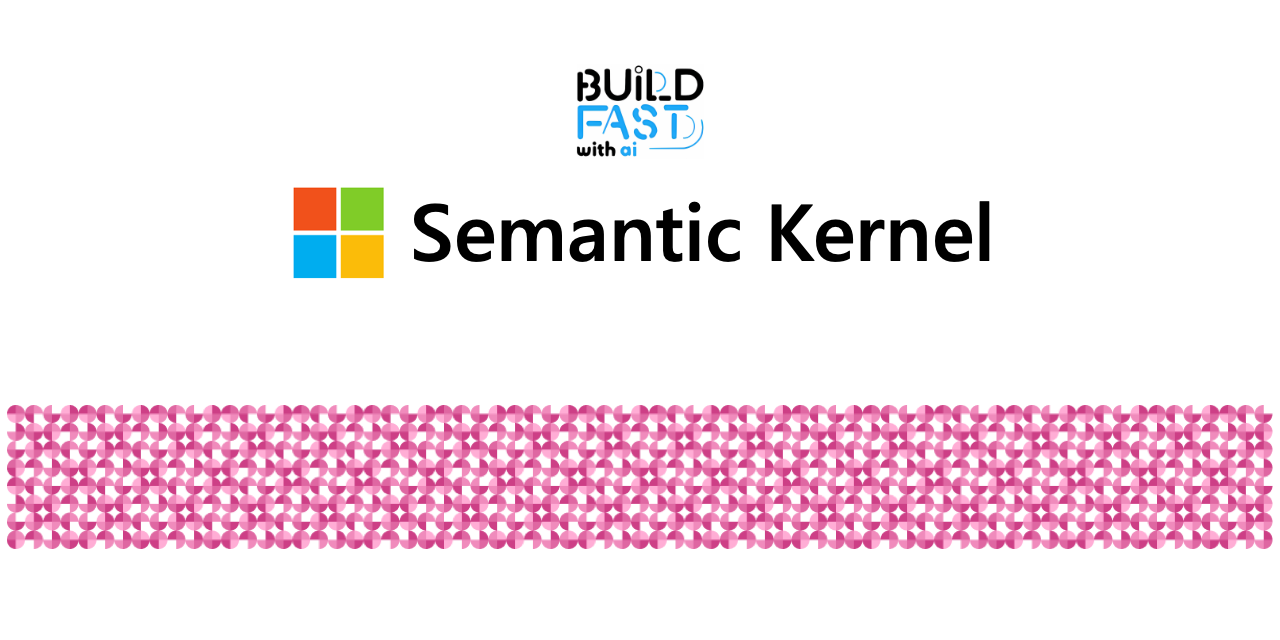
Will you hesitate and miss the chance of a lifetime?
Don’t wait—join Gen AI Launch Pad 2025 and lead the change.
Introduction
Semantic Kernel is an open-source framework designed to seamlessly integrate large language models (LLMs) into traditional applications. Whether you’re looking to automate tasks, create intelligent assistants, or build scalable AI-driven systems, Semantic Kernel offers the tools and modularity to simplify the process. In this blog, we’ll walk you through a hands-on notebook that showcases its features, capabilities, and practical applications.
By the end of this post, you’ll learn:
- How to set up Semantic Kernel.
- Key functionalities such as file handling, task orchestration, and dynamic interaction.
- Real-world use cases for these functionalities.
- How to dive deeper into this powerful framework.
Detailed Explanation
1. Installing and Configuring Semantic Kernel
Code Block:
!pip install semantic-kernel from semantic_kernel import __version__ print(f"Semantic Kernel version: {__version__}")
What It Does:
This block installs the Semantic Kernel library and verifies the installed version. This is your first step in setting up the environment to leverage Semantic Kernel’s capabilities.
Expected Output:
Semantic Kernel version: <installed_version>
Key Insights:
- Library Installation: Use
!pip install
to fetch the latest version from PyPI. - Version Verification: Ensures compatibility with your existing code or dependencies.
Real-World Application:
This step ensures that you have the required dependencies before building AI-powered applications.
2. Initial Notebook Configuration
Code Block:
import os import sys notebook_dir = os.path.abspath("") parent_dir = os.path.dirname(notebook_dir) grandparent_dir = os.path.dirname(parent_dir) sys.path.append(grandparent_dir)
What It Does:
Sets up the environment for seamless execution by managing system paths. This ensures the notebook can locate required modules and dependencies.
Key Functions:
os.path.abspath("")
: Gets the absolute path of the current directory.sys.path.append
: Adds paths to the Python interpreter’s search path.
Real-World Application:
This step is crucial when your project relies on custom modules stored outside the current working directory.
3. Configuring the Kernel
Code Block:
from semantic_kernel import Kernel kernel = Kernel()
What It Does:
Initializes the Semantic Kernel object, which acts as the central orchestrator for all subsequent operations.
Key Insights:
- The
Kernel
class is the entry point for creating tasks, managing agents, and leveraging LLMs. - Acts as the foundation for all interactions within the Semantic Kernel framework.
Real-World Application:
Essential for applications that require AI task orchestration, such as chat assistants or automated workflows.
4. Using OpenAI Configuration
Code Block:
from google.colab import userdata import os OPENAI_API_KEY= userdata.get('OPENAI_API_KEY') OPENAI_CHAT_MODEL_ID='gpt-4o' os.environ['GLOBAL_LLM_SERVICE'] = 'OpenAI' os.environ['OPENAI_API_KEY'] = userdata.get('OPENAI_API_KEY') os.environ['OPENAI_CHAT_MODEL_ID'] = 'gpt-4o' os.environ['OPENAI_TEXT_MODEL_ID'] = 'gpt-4'
What It Does:
Configures Semantic Kernel to use OpenAI’s GPT models by setting environment variables for API keys and model IDs.
Key Functions:
- Environment Variables: Store sensitive information like API keys.
userdata.get
: Fetches user-specific credentials securely.
Real-World Application:
Allows developers to switch between AI providers (OpenAI, Azure, etc.) effortlessly by reconfiguring environment variables.
5. Creating an OpenAI Assistant
Code Block:
from semantic_kernel.agents.open_ai import OpenAIAssistantAgent assistant_agent = await OpenAIAssistantAgent.create( service_id="AIAssistant", description="An AI assistant that helps with everyday tasks.", instructions="Help the user with their task.", enable_code_interpreter=True, enable_file_search=True, api_key=OPENAI_API_KEY, model_id=OPENAI_CHAT_MODEL_ID, )
What It Does:
Creates an AI assistant agent capable of interpreting code, handling file searches, and interacting dynamically with users.
Key Insights:
- Service ID: Uniquely identifies the agent.
- Features: Enable advanced capabilities like code interpretation and file search.
Expected Output:
A functional assistant ready to accept user inputs and perform tasks.
Real-World Application:
Ideal for developing intelligent chatbots, interactive tools, or task managers.
6. Handling File Uploads
Code Block:
def parse_upload_command(user_input: str): match = re.search(r"upload (\w+) (\S+)", user_input) if match: return match.group(1), match.group(2) return None, None
What It Does:
Parses user input to identify file upload commands and extract relevant details (purpose and file path).
Key Functions:
- Regex Matching: Extracts command components like purpose and file path.
Real-World Application:
Used in conversational agents to interpret user-uploaded files for analysis or processing.
7. Enabling Code Interpretation
Code Block:
async def enable_code_interpreter(assistant_agent, file_id): assistant_agent.code_interpreter_file_ids.append(file_id) tools = [{"type": "file_search"}, {"type": "code_interpreter"}] tool_resources = {"code_interpreter": {"file_ids": assistant_agent.code_interpreter_file_ids}} await assistant_agent.modify_assistant( assistant_id=assistant_agent.assistant.id, tools=tools, tool_resources=tool_resources ) print("File enabled for code interpreter.")
What It Does:
Adds uploaded files to the assistant’s code interpreter tool, enabling analysis and interaction with the file’s contents.
Expected Output:
File enabled for code interpreter.
Conclusion
Semantic Kernel is a versatile framework for integrating LLMs into real-world applications. From task orchestration to building intelligent assistants, its modularity and scalability make it an invaluable tool for developers.
Key Takeaways:
- Simplifies AI orchestration through modular architecture.
- Supports advanced features like dynamic task planning and code interpretation.
- Seamlessly integrates with OpenAI, Azure, and Hugging Face.
Resources
- Semantic Kernel GitHub Repository
- OpenAI Documentation
- Hugging Face
- Python Asyncio Documentation
- Semantic Kernel Experiment Notebook
---------------------------
Stay Updated:- Follow Build Fast with AI pages for all the latest AI updates and resources.
Experts predict 2025 will be the defining year for Gen AI implementation.Want to be ahead of the curve?
Join Build Fast with AI’s Gen AI Launch Pad 2025 - your accelerated path to mastering AI tools and building revolutionary applications.
---------------------------
Resources and Community
Join our community of 12,000+ AI enthusiasts and learn to build powerful AI applications! Whether you're a beginner or an experienced developer, this tutorial will help you understand and implement AI agents in your projects.
- Website: www.buildfastwithai.com
- LinkedIn: linkedin.com/company/build-fast-with-ai/
- Instagram: instagram.com/buildfastwithai/
- Twitter: x.com/satvikps
- Telegram: t.me/BuildFastWithAI