OpenLLMetry: Mastering Observability in LLM Applications
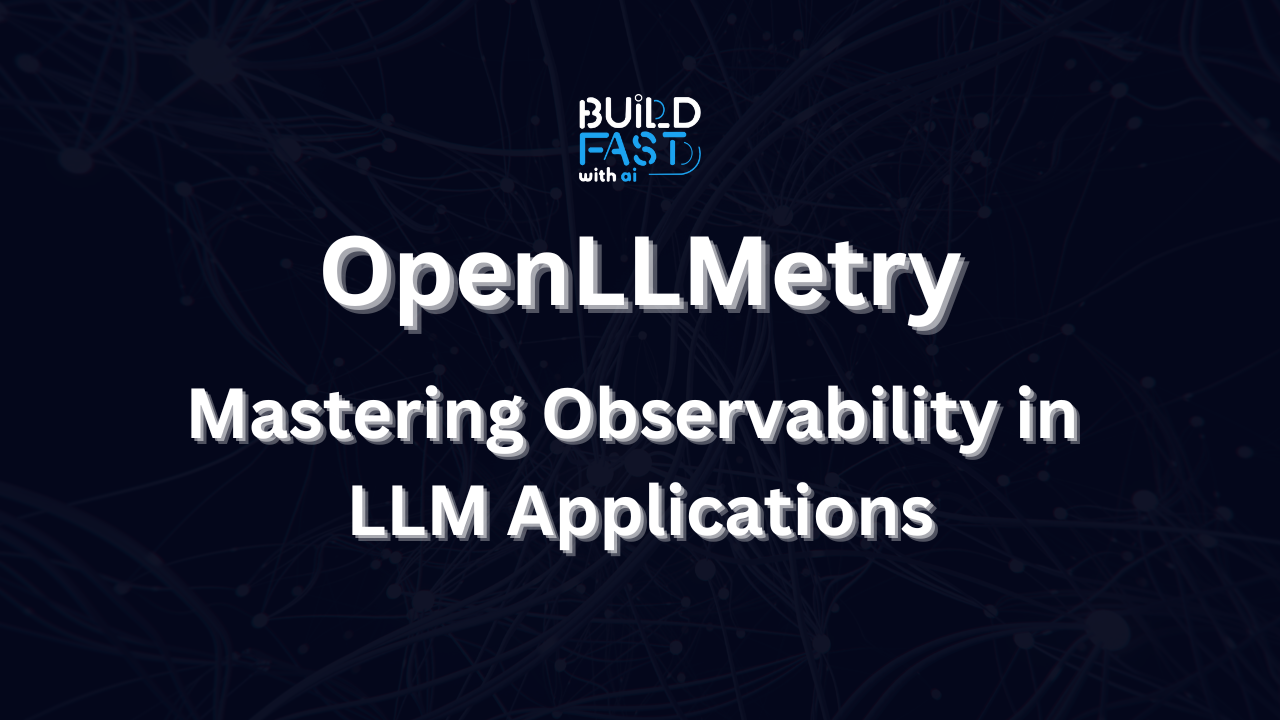
Will you be left behind or step forward into the future?
Gen AI Launch Pad 2025 is your chance to shape tomorrow.
Introduction
As LLM-powered applications become more sophisticated, understanding their internal processes is crucial for debugging, performance optimization, and security. OpenLLMetry (Open Large Language Model Telemetry) provides an observability toolkit tailored for LLM-based applications. It allows developers to track data flow, monitor prompts, collect user feedback, and optimize workflows effortlessly.
In this tutorial, we'll explore how to integrate OpenLLMetry into an LLM application, analyze its key features, and demonstrate its practical use through coding examples.
Setting Up OpenLLMetry
Before diving into implementation, install the necessary dependencies:
pip install traceloop-sdk langchain_community langchain_openai
Next, configure API keys for authentication:
from google.colab import userdata import os os.environ["OPENAI_API_KEY"] = userdata.get('OPENAI_API_KEY') os.environ['TRACELOOP_API_KEY'] = userdata.get('TRACELOOP_API_KEY')
This setup ensures secure communication between OpenLLMetry and your LLM application.
Initializing OpenLLMetry for LLM Observability
Once dependencies are installed, initialize OpenLLMetry within your application:
from traceloop.sdk import Traceloop Traceloop.init(app_name="llm_observability_demo")
With this initialization, OpenLLMetry starts collecting traces, which help visualize and analyze LLM request-response cycles.
Tracking LLM Calls with OpenLLMetry
One of OpenLLMetry’s key features is tracing LLM interactions. Let’s define a function that tracks a simple joke generation request:
from openai import OpenAI from traceloop.sdk.tracing.manual import LLMMessage, track_llm_call import os client = OpenAI(api_key=os.environ["OPENAI_API_KEY"]) with track_llm_call(vendor="openai", type="chat") as span: span.report_request( model="gpt-3.5-turbo", messages=[LLMMessage(role="user", content="Tell me a joke about observability")], ) response = client.chat.completions.create( model="gpt-3.5-turbo", messages=[{"role": "user", "content": "Tell me a joke about observability"}] ) span.report_response(response.model, [msg.message.content for msg in response.choices]) print(response.choices[0].message.content)
How It Works
track_llm_call
monitors the OpenAI API call.report_request
logs the LLM request.report_response
captures the model’s response.- The result is a structured trace, enabling debugging and performance analysis.
Monitoring Agents and Tools
Beyond basic tracking, OpenLLMetry supports agent and tool monitoring, useful for autonomous workflows. Let’s create a joke translation agent:
from traceloop.sdk.decorators import agent, tool @tool(name="history_jokes") def history_jokes_tool(): response = client.chat.completions.create( model="gpt-3.5-turbo", messages=[{"role": "user", "content": "Give me a history-related joke"}] ) return response.choices[0].message.content @agent(name="joke_translation") def translate_joke_to_pirate(): joke = history_jokes_tool() response = client.chat.completions.create( model="gpt-3.5-turbo", messages=[{"role": "user", "content": f"Translate this joke into pirate speak: \n{joke}"}] ) return response.choices[0].message.content print(translate_joke_to_pirate())
How It Works
@tool
decorates a function that retrieves history-related jokes.@agent
monitors the joke translation process.- This enables observability over both tools and agents.
Version Control for Prompts
Tracking prompt versions is crucial for evaluating model performance. OpenLLMetry provides prompt observability:
def tell_joke(subject): Traceloop.set_prompt("Tell me a joke about {subject}", {"subject": subject}, version=1) response = client.chat.completions.create( model="gpt-3.5-turbo", messages=[{"role": "user", "content": f"Tell me a joke about {subject}"}] ) return response.choices[0].message.content Traceloop.init(app_name="joke_app") print(tell_joke("OpenTelemetry"))
Why This Matters
- Helps track changes in prompts over time.
- Evaluates prompt effectiveness.
- Identifies regression issues in model responses.
Conclusion
OpenLLMetry is a powerful observability tool for LLM applications, offering:
- LLM Call Tracing – Monitor request-response cycles.
- Prompt Versioning – Track and analyze prompt performance.
- Agent & Tool Monitoring – Gain insights into autonomous system workflows.
- User Feedback Tracking – Collect and act on feedback for model improvement.
References
---------------------------
Stay Updated:- Follow Build Fast with AI pages for all the latest AI updates and resources.
Experts predict 2025 will be the defining year for Gen AI Implementation. Want to be ahead of the curve?
Join Build Fast with AI’s Gen AI Launch Pad 2025 - your accelerated path to mastering AI tools and building revolutionary applications.
---------------------------
Resources and Community
Join our community of 12,000+ AI enthusiasts and learn to build powerful AI applications! Whether you're a beginner or an experienced developer, our resources will help you understand and implement Generative AI in your projects.
- Website: www.buildfastwithai.com
- LinkedIn: linkedin.com/company/build-fast-with-ai/
- Instagram: instagram.com/buildfastwithai/
- Twitter: x.com/satvikps
- Telegram: t.me/BuildFastWithAI