MongoDB for AI: A Complete Guide to Vector Search and RAG Implementation
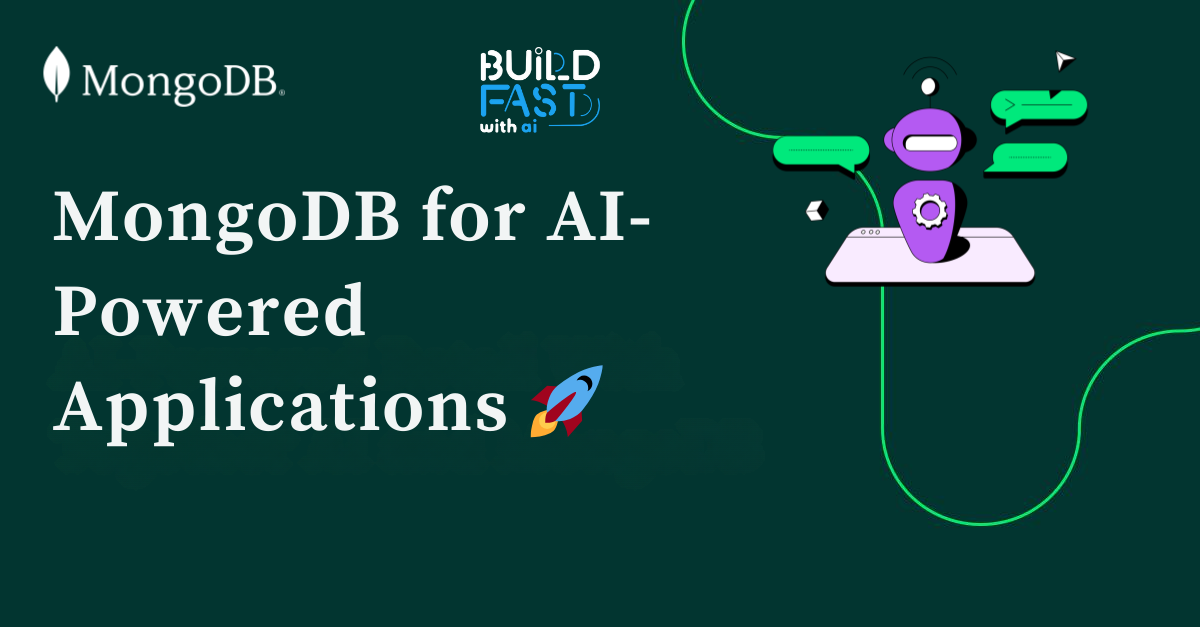
Will you stand by as the future unfolds, or will you seize the opportunity to create it?
Be part of Gen AI Launch Pad 2025 and take control.
Introduction
In the age of artificial intelligence (AI) and large-scale data applications, having a flexible, high-performance database is essential. MongoDB, a NoSQL database, provides an ideal solution with its document-oriented storage, powerful querying capabilities, and seamless integration with AI frameworks. In this guide, we will walk through how MongoDB can be leveraged for AI applications, particularly for Retrieval-Augmented Generation (RAG). By the end of this post, you'll learn how to:
- Set up MongoDB and establish a connection
- Store and retrieve structured and unstructured data
- Perform CRUD (Create, Read, Update, Delete) operations
- Use MongoDB's vector search for AI-driven applications
- Implement RAG with MongoDB and OpenAI embeddings
Setting Up MongoDB
Before diving into AI-powered applications, let’s install and set up MongoDB.
Installing Required Packages
To interact with MongoDB, we need to install the required Python libraries:
!pip install pymongo langchain tiktoken faiss-cpu pypdf
These libraries help us connect to MongoDB, process text data, generate embeddings, and perform similarity searches.
Setting Up API Keys
To access MongoDB Atlas and OpenAI APIs, we configure the API keys securely:
import os from google.colab import userdata os.environ["OPENAI_API_KEY"] = userdata.get('OPENAI_API_KEY') MONGODB_URI = userdata.get('MONGODB_URI')
This ensures that sensitive credentials are not hardcoded into the script.
Connecting to MongoDB
Once the environment is set up, we establish a connection with MongoDB Atlas.
from pymongo import MongoClient connection_string = MONGODB_URI client = MongoClient(connection_string) client.admin.command('ping') print("✅ Connected to MongoDB Atlas successfully!")
Expected Output:
✅ Connected to MongoDB Atlas successfully!
This confirms that we have successfully connected to our database.
Working with Databases and Collections
MongoDB stores data in databases and collections. Let's create a database and a collection.
db = client["my_database"] collection = db["users"]
Now, we can insert and retrieve data.
CRUD Operations in MongoDB
Insert a Single Document
user = {"name": "John Doe", "age": 30, "city": "New York"} collection.insert_one(user) print("✅ One document inserted!")
Expected Output:
✅ One document inserted!
Insert Multiple Documents
users = [ {"name": "Alice", "age": 25, "city": "Los Angeles"}, {"name": "Bob", "age": 28, "city": "Chicago"}, ] collection.insert_many(users) print("✅ Multiple documents inserted!")
Expected Output:
✅ Multiple documents inserted!
Retrieve Data
Find a single document:
user = collection.find_one({"name": "John Doe"}) print("👤 Found user:", user)
Expected Output:
👤 Found user: {'_id': ObjectId('xxx'), 'name': 'John Doe', 'age': 30, 'city': 'New York'}
Find all documents:
for user in collection.find(): print(user)
Find documents with a condition:
for user in collection.find({"city": "New York"}): print(user)
Update a Document
collection.update_one({"name": "John Doe"}, {"$set": {"age": 31}}) print("✅ User updated!")
Delete a Document
collection.delete_one({"name": "Alice"}) print("✅ One document deleted!")
Drop a Collection
collection.drop() print("🗑️ Collection deleted!")
Implementing Retrieval-Augmented Generation (RAG) with MongoDB
Retrieval-Augmented Generation (RAG) enhances AI models by retrieving relevant data before generating a response. MongoDB’s vector search capabilities make this possible.
Generate and Store Embeddings
from langchain.embeddings.openai import OpenAIEmbeddings embeddings = OpenAIEmbeddings() def get_embedding(text: str): return embeddings.embed_query(text)
Load and Split Text Data
from langchain.document_loaders import PyPDFLoader from langchain.text_splitter import RecursiveCharacterTextSplitter pdf_url = "https://investors.mongodb.com/node/12236/pdf" loader = PyPDFLoader(pdf_url) data = loader.load() text_splitter = RecursiveCharacterTextSplitter(chunk_size=400, chunk_overlap=20) documents = text_splitter.split_documents(data)
Expected Output:
Loaded and split 88 documents from PDF.
Store Embeddings in MongoDB
docs_to_insert = [] for doc in documents: embedding = get_embedding(doc.page_content) docs_to_insert.append({"text": doc.page_content, "embedding": embedding}) collection.insert_many(docs_to_insert) print(f"Inserted {len(docs_to_insert)} documents into MongoDB")
Performing Vector Search in MongoDB
Create Vector Search Index
from pymongo.operations import SearchIndexModel index_name = "vector_index" search_index_model = SearchIndexModel( definition={"fields": [{"type": "vector", "numDimensions": 1536, "path": "embedding", "similarity": "cosine"}]}, name=index_name, type="vectorSearch" ) collection.create_search_index(model=search_index_model) print(f"Created vector search index '{index_name}' on MongoDB collection.")
Run Vector Search Query
def get_query_results(query: str, limit: int = 5): query_embedding = get_embedding(query) pipeline = [{"$vectorSearch": {"index": index_name, "queryVector": query_embedding, "path": "embedding", "numCandidates": 10, "limit": limit}}, {"$project": {"_id": 0, "text": 1}}] return list(collection.aggregate(pipeline))
Conclusion
In this guide, we explored MongoDB's capabilities in AI applications, from basic CRUD operations to advanced vector search for RAG. MongoDB's flexibility, scalability, and AI-friendly integrations make it an excellent choice for modern AI-driven systems.
Resources
---------------------------
Stay Updated:- Follow Build Fast with AI pages for all the latest AI updates and resources.
Experts predict 2025 will be the defining year for Gen AI Implementation. Want to be ahead of the curve?
Join Build Fast with AI’s Gen AI Launch Pad 2025 - your accelerated path to mastering AI tools and building revolutionary applications.
---------------------------
Resources and Community
Join our community of 12,000+ AI enthusiasts and learn to build powerful AI applications! Whether you're a beginner or an experienced developer, this tutorial will help you understand and implement AI agents in your projects.
- Website: www.buildfastwithai.com
- LinkedIn: linkedin.com/company/build-fast-with-ai/
- Instagram: instagram.com/buildfastwithai/
- Twitter: x.com/satvikps
- Telegram: t.me/BuildFastWithAI