Build Stunning AI Apps in Minutes with Gradio and Google Colab
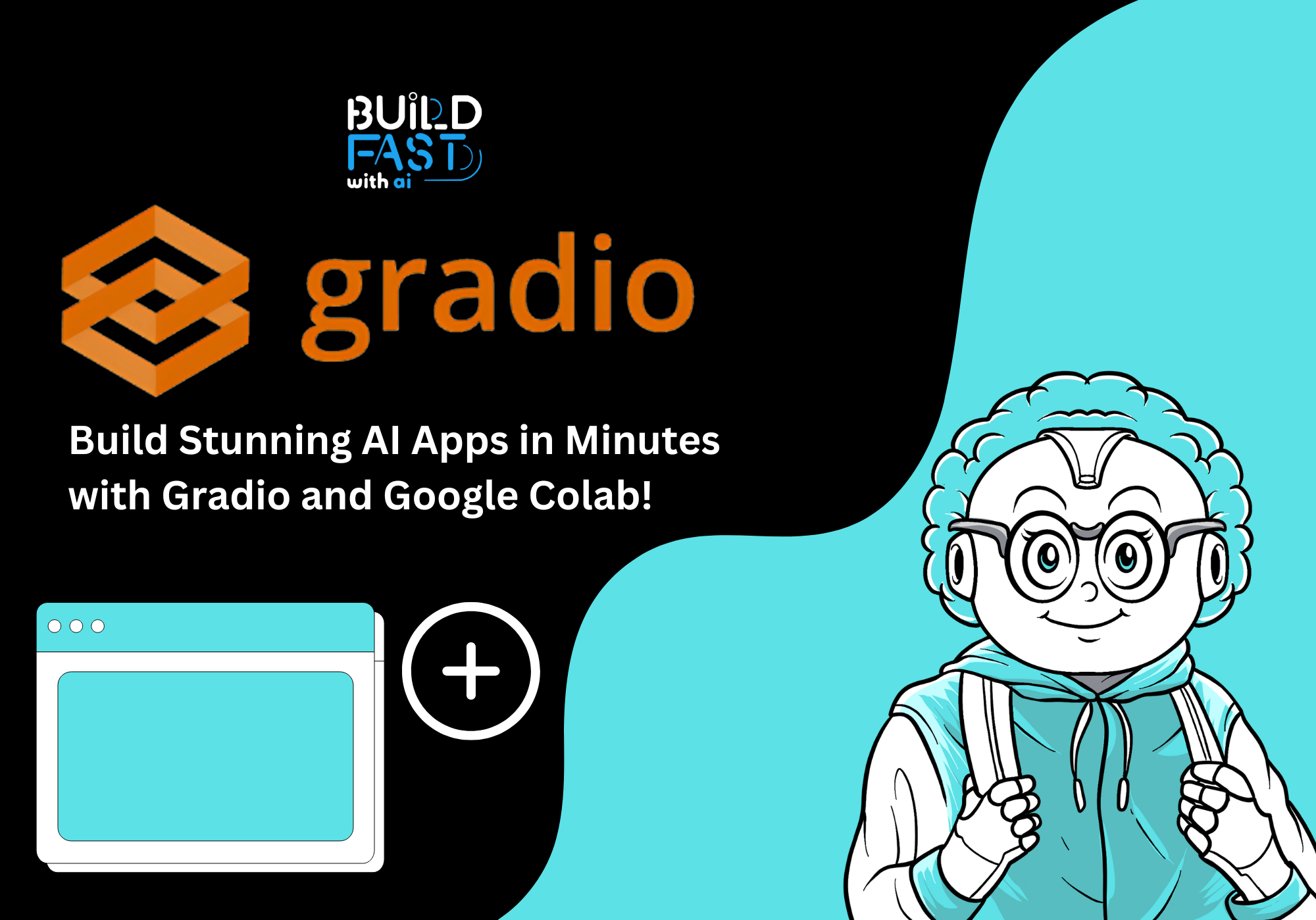
Are you hesitating while the next big breakthrough happens?
Don’t wait—be part of Gen AI Launch Pad 2025 and make history.
Introduction
Gradio is a game-changing open-source Python library that simplifies the creation of intuitive user interfaces for machine learning (ML) models and data science applications. With Gradio, developers can build and share interactive applications in a matter of minutes, directly from their Python code. Whether you want to deploy a real-time transcription tool, create an AI-powered image generator, or build a multi-component interface, Gradio has you covered.
In this comprehensive guide, we will explore:
- How to set up Gradio in Google Colab.
- Building various AI applications using Gradio.
- A detailed explanation of the key components and logic in each example.
- Real-world scenarios where these applications can be applied.
- Useful resources to deepen your knowledge.
By the end of this blog, you’ll have the tools and understanding to create your own Gradio-powered applications.
Setting Up Gradio in Colab
Google Colab provides an excellent environment to experiment with Gradio without the need for complex local setups. To begin, install Gradio and its dependencies using the following command:
!pip install -U langchain-community langchain_openai google-search-results gradio openai_gradio
This command installs Gradio alongside libraries for language model integrations like OpenAI and Hugging Face, as well as utilities for accessing search results.
Once installed, you’re ready to start building interactive applications.
1. Image Generation with Gradio
Overview
This example demonstrates how to create an image generation application using Gradio and tools from Hugging Face. Users can input a description, and the model generates an image matching the prompt.
Step-by-Step Explanation
Code Snippet
import gradio as gr from gradio import ChatMessage from transformers import Tool, ReactCodeAgent from transformers.agents import stream_to_gradio, HfApiEngine from dataclasses import asdict import os # Import tool from Hugging Face Spaces image_generation_tool = Tool.from_space( space_id="black-forest-labs/FLUX.1-schnell", name="image_generator", description="Generates an image following your prompt. Returns a PIL Image.", api_name="/infer", ) # Access token for Hugging Face access_token = os.environ.get("HUGGINGFACE_HUB_TOKEN") if access_token: llm_engine = HfApiEngine("Qwen/Qwen2.5-Coder-32B-Instruct", token=access_token) else: llm_engine = HfApiEngine("Qwen/Qwen2.5-Coder-32B-Instruct") # Initialize the agent with tools and engine agent = ReactCodeAgent(tools=[image_generation_tool], llm_engine=llm_engine) def interact_with_agent(prompt, history): messages = [] yield messages for msg in stream_to_gradio(agent, prompt): messages.append(asdict(msg)) yield messages yield messages # Build the Gradio interface demo = gr.ChatInterface( interact_with_agent, chatbot=gr.Chatbot( label="Agent", type="messages", avatar_images=( None, "https://em-content.zobj.net/source/twitter/53/robot-face_1f916.png", ), ), examples=[ ["Generate an image of an astronaut riding an alligator"], ["I am writing a children's book for my daughter. Can you help me with some illustrations?"], ], type="messages", ) if __name__ == "__main__": demo.launch()
Key Components Explained
Tool.from_space
: This function imports a pre-trained image generation tool hosted on Hugging Face Spaces. Thespace_id
identifies the specific tool.ReactCodeAgent
: The ReactCodeAgent is initialized with the image generation tool and a language model engine (HfApiEngine). It serves as the backend for processing user prompts.gr.ChatInterface
: This creates a chat-based interface with an input field for user prompts and a chatbot that displays responses.- Example Prompts: Users can try predefined examples such as “Generate an image of an astronaut riding an alligator” to see how the tool works.
Expected Output
- A user-friendly chat interface with input and output fields.
- Responses include generated images based on user prompts.
Real-World Applications
- Creative Industries: Generate illustrations for children’s books, marketing campaigns, or social media content.
- Education: Help students visualize complex concepts or historical events.
- Design Prototyping: Create concept art or draft designs for products.
2. Real-Time Speech Recognition
Overview
In this example, we use Gradio to build a live transcription tool. The application uses Hugging Face’s Whisper model to transcribe speech in real time.
Step-by-Step Explanation
Code Snippet
import gradio as gr from transformers import pipeline import numpy as np transcriber = pipeline("automatic-speech-recognition", model="openai/whisper-base.en") def transcribe(stream, new_chunk): sr, y = new_chunk # Convert to mono if stereo if y.ndim > 1: y = y.mean(axis=1) y = y.astype(np.float32) y /= np.max(np.abs(y)) if stream is not None: stream = np.concatenate([stream, y]) else: stream = y return stream, transcriber({"sampling_rate": sr, "raw": stream})["text"] demo = gr.Interface( transcribe, ["state", gr.Audio(sources=["microphone"], streaming=True)], ["state", "text"], live=True, ) demo.launch()
Key Components Explained
pipeline
: Initializes the Whisper model for automatic speech recognition.- Audio Preprocessing: The function converts stereo audio to mono and normalizes it for consistent input.
- Live Streaming: Gradio’s
gr.Audio
supports live audio input, allowing users to provide real-time speech data.
Expected Output
- Live text transcription appears on the interface as you speak into the microphone.
Real-World Applications
- Accessibility: Provide subtitles for live events to assist people with hearing impairments.
- Note-Taking: Automatically transcribe meetings or lectures for later reference.
- Voice Interfaces: Enable voice-driven commands for smart home systems or customer support tools.
Conclusion
Gradio unlocks the potential to create engaging and intuitive AI-powered applications with minimal coding. By combining Gradio with libraries like Hugging Face Transformers, you can prototype, test, and share applications effortlessly. From generating creative images to enabling real-time speech transcription, the possibilities are endless.
Key Takeaways
- Gradio’s flexibility and ease of use make it an excellent choice for AI developers.
- Applications can range from creative tools to accessibility solutions.
- Integration with platforms like Hugging Face ensures access to state-of-the-art models.
Next Steps
- Explore additional Gradio components like Blocks for multi-component layouts.
- Experiment with other pre-trained models on Hugging Face.
- Share your applications via Colab or host them on Hugging Face Spaces for wider accessibility.
Resources
---------------------------
Stay Updated:- Follow Build Fast with AI pages for all the latest AI updates and resources.
Experts predict 2025 will be the defining year for Gen AI implementation.Want to be ahead of the curve?
Join Build Fast with AI’s Gen AI Launch Pad 2025 - your accelerated path to mastering AI tools and building revolutionary applications.
---------------------------
Resources and Community
Join our community of 12,000+ AI enthusiasts and learn to build powerful AI applications! Whether you're a beginner or an experienced developer, this tutorial will help you understand and implement AI agents in your projects.
- Website: www.buildfastwithai.com
- LinkedIn: linkedin.com/company/build-fast-with-ai/
- Instagram: instagram.com/buildfastwithai/
- Twitter: x.com/satvikps
- Telegram: t.me/BuildFastWithAI