How Gensim Makes Topic Modeling Easy for Any Dataset
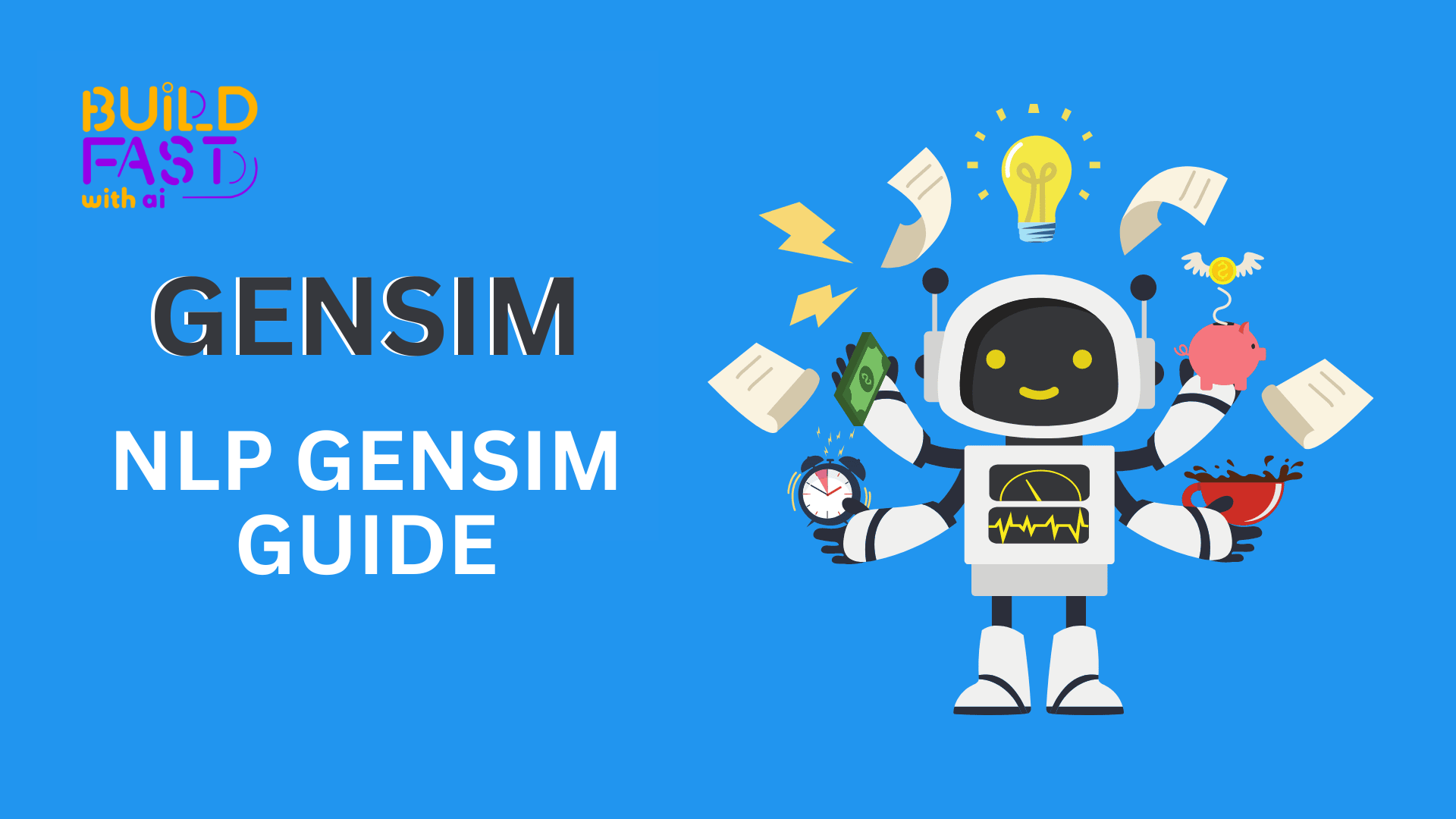
Are you letting today’s opportunities pass you by?
Join Gen AI Launch Pad 2025 and create the future you envision.
Introduction
Natural Language Processing (NLP) has become an essential field in data science, empowering applications such as sentiment analysis, text classification, and search engines. A key aspect of NLP is understanding and deriving meaning from large corpora of text. This is where Gensim, an open-source Python library, shines. Gensim is tailored for unsupervised topic modeling and document similarity analysis, enabling developers to work with massive datasets efficiently.
In this blog, we will explore how to use Gensim for:
- Topic modeling with algorithms like Latent Dirichlet Allocation (LDA) and Latent Semantic Indexing (LSI).
- Calculating document similarity.
- Preprocessing textual data for NLP tasks.
By the end of this guide, you’ll have a clear understanding of Gensim’s features, how to implement them, and their real-world applications.
What is Gensim?
Gensim is a Python library that specializes in unsupervised learning for textual data. It provides efficient algorithms for:
- Topic Modeling: Discovering hidden themes in large text datasets.
- Document Similarity: Measuring how similar two pieces of text are.
- Semantic Analysis: Extracting meaningful relationships between words and concepts.
Key features of Gensim include:
- Scalability for large text corpora.
- Integration with NLP pipelines.
- Support for out-of-core processing (streaming data that doesn’t fit in memory).
Let’s dive into the practical implementation of these features.
1. Setting Up Gensim
Before we start coding, let’s set up the environment. Install Gensim using pip:
pip install gensim
Additionally, we’ll use Python’s logging
module to monitor Gensim’s processes.
import logging logging.basicConfig(format='%(asctime)s : %(levelname)s : %(message)s', level=logging.INFO)
This setup ensures that you receive real-time updates on the progress of operations such as training models.
2. Preparing the Text Corpus
A text corpus is the foundation for any NLP task. We’ll use a small example dataset to demonstrate preprocessing steps.
Code: Creating the Corpus
from collections import defaultdict from gensim import corpora # Example dataset documents = [ "Human machine interface for lab abc computer applications", "A survey of user opinion of computer system response time", "The EPS user interface management system", "System and human system engineering testing of EPS", "Relation of user perceived response time to error measurement", "The generation of random binary unordered trees", "The intersection graph of paths in trees", "Graph minors IV Widths of trees and well quasi ordering", "Graph minors A survey", ] # Removing stop words stoplist = set('for a of the and to in'.split()) texts = [ [word for word in document.lower().split() if word not in stoplist] for document in documents ] # Removing infrequent words frequency = defaultdict(int) for text in texts: for token in text: frequency[token] += 1 texts = [ [token for token in text if frequency[token] > 1] for text in texts ] # Creating a dictionary dictionary = corpora.Dictionary(texts) corpus = [dictionary.doc2bow(text) for text in texts]
Explanation
- Stop Words: Common words like “for,” “and,” and “in” are removed to focus on meaningful words.
- Infrequent Words: Words that appear only once are filtered out to reduce noise.
- Dictionary: Maps unique tokens (words) to integer IDs.
- Bag-of-Words (BoW): Represents each document as a vector of token counts.
Expected Output
After preprocessing, the dictionary may look like this:
Dictionary<12 unique tokens: ['computer', 'human', 'interface', 'response', 'survey']...>
3. Topic Modeling with LSI
Latent Semantic Indexing (LSI) is a technique to identify patterns in the relationships between terms and concepts in text.
Code: Creating an LSI Model
from gensim import models # Creating an LSI model lsi = models.LsiModel(corpus, id2word=dictionary, num_topics=2) # Testing the model doc = "Human computer interaction" vec_bow = dictionary.doc2bow(doc.lower().split()) vec_lsi = lsi[vec_bow] print(vec_lsi)
Explanation
- LSI Model: Maps the corpus into a lower-dimensional space with
num_topics
dimensions. - Querying: Converts a new document (“Human computer interaction”) into the LSI space.
Expected Output
[(0, 0.46182100453271535), (1, -0.07002766527900064)]
This output shows the contribution of the query document to each topic.
4. Document Similarity
One of Gensim’s strengths is calculating how similar documents are to each other.
Code: Measuring Document Similarity
from gensim import similarities # Creating a similarity index index = similarities.MatrixSimilarity(lsi[corpus]) # Querying the similarity index sims = index[vec_lsi] # Sorting and displaying results sims = sorted(enumerate(sims), key=lambda item: -item[1]) for doc_position, doc_score in sims: print(doc_score, documents[doc_position])
Explanation
- MatrixSimilarity: Converts the LSI space into a structure for similarity comparisons.
- Query: Computes the similarity of the query document to all documents in the corpus.
Expected Output
0.9984453 The EPS user interface management system 0.998093 Human machine interface for lab abc computer applications 0.9865886 System and human system engineering testing of EPS ...
5. Saving and Loading Models
Preserving your models and indices for reuse is essential for production applications.
Code
# Saving and loading the similarity index index.save('/tmp/deerwester.index') index = similarities.MatrixSimilarity.load('/tmp/deerwester.index')
Explanation
- Save models and indices to disk for later use, eliminating the need to recreate them.
Conclusion
In this blog, we explored Gensim’s capabilities for:
- Preprocessing text corpora.
- Topic modeling using LSI.
- Calculating document similarity.
Gensim’s scalability and support for unsupervised learning make it a go-to library for text analysis. By understanding these techniques, you can build applications for search engines, recommendation systems, and content clustering.
Resources
---------------------------
Stay Updated:- Follow Build Fast with AI pages for all the latest AI updates and resources.
Experts predict 2025 will be the defining year for Gen AI Implementation. Want to be ahead of the curve?
Join Build Fast with AI’s Gen AI Launch Pad 2025 - your accelerated path to mastering AI tools and building revolutionary applications.
---------------------------
Resources and Community
Join our community of 12,000+ AI enthusiasts and learn to build powerful AI applications! Whether you're a beginner or an experienced developer, this tutorial will help you understand and implement AI agents in your projects.
- Website: www.buildfastwithai.com
- LinkedIn: linkedin.com/company/build-fast-with-ai/
- Instagram: instagram.com/buildfastwithai/
- Twitter: x.com/satvikps
- Telegram: t.me/BuildFastWithAI