DeepPavlov: Advanced Conversational AI Framework
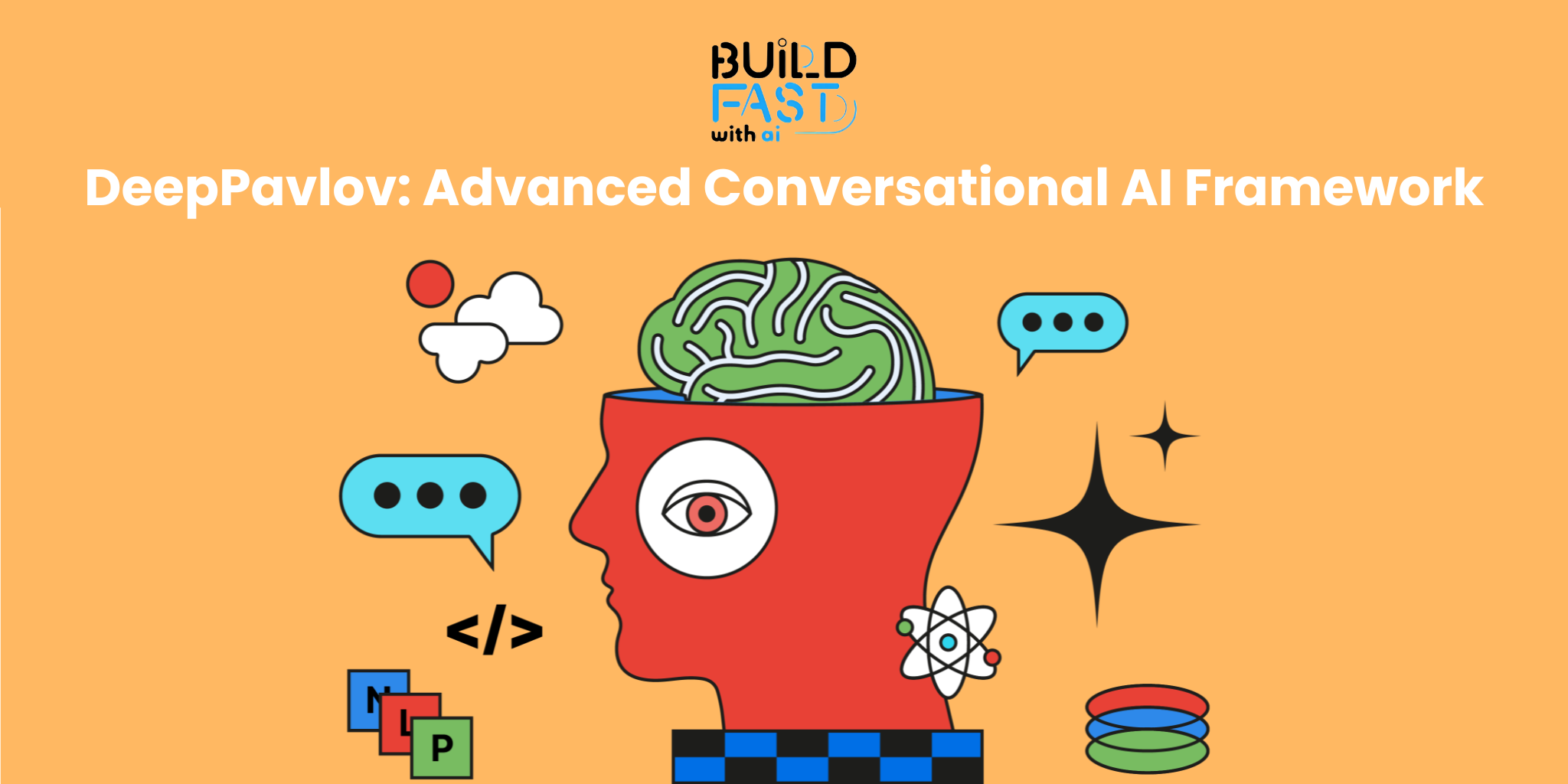
Will you hesitate and miss the chance of a lifetime?
Don’t wait—join Gen AI Launch Pad 2025 and lead the change.
Introduction:
In this comprehensive blog post, we'll delve deep into DeepPavlov, an open-source framework designed to empower developers to build sophisticated conversational AI systems. With a focus on NLP (Natural Language Processing) tasks, DeepPavlov offers a wide range of pre-trained models and tools that can be customized to fit the specific needs of various applications. Whether you’re building a chatbot, a question-answering system, or any other NLP-based application, DeepPavlov has you covered.
By the end of this article, you will have an in-depth understanding of how to use DeepPavlov for different NLP tasks, such as open-domain question answering, knowledge-based question answering, text classification, spelling correction, and more. We’ll go step by step through several code blocks that demonstrate these capabilities, providing both theoretical explanations and practical examples.
Detailed Explanation:
Let’s walk through each block of code, explaining its function, expected output, and real-world applications.
1. Installing DeepPavlov
Before we dive into the code, it’s essential to install the DeepPavlov framework in your Python environment. DeepPavlov is a powerful framework with multiple tools, so we will first ensure everything is set up properly.
pip install deeppavlov
Explanation:
pip install deeppavlov
: This command installs the entire DeepPavlov package, along with its dependencies, into your Python environment. It’s as simple as that!
Once the installation is complete, you're ready to start building your conversational AI applications.
2. Open-Domain Question Answering
The first task we’ll cover is Open-Domain Question Answering. This functionality allows a system to answer questions that are not limited to a specific domain, such as trivia questions, general knowledge queries, or facts drawn from resources like Wikipedia.
Here’s how you can use the Open-Domain Question Answering model:
from deeppavlov import build_model from deeppavlov import configs import nltk nltk.download('punkt_tab') model = build_model('en_odqa_infer_wiki', download=True, install=True) questions = ["What is the name of Darth Vader's son?", 'Who was the first president of France?'] answer, answer_score, answer_place = model(questions) print(answer)
Explanation:
build_model()
: This function loads a pre-trained model from the configuration files provided by DeepPavlov. Here, the model'en_odqa_infer_wiki'
is used, which is trained on open-domain question answering tasks.nltk.download('punkt_tab')
: The NLTK library is used here to download the necessary tokenization resources for sentence segmentation.model(questions)
: We pass in a list of questions, and the model answers them based on the knowledge it has learned from Wikipedia.
Expected Output:
['Luke Skywalker', 'Louis-Napoleon Bonaparte']
Real-World Application:
- This code can be applied in systems such as virtual assistants (like Siri or Google Assistant), customer support bots, and search engines that provide users with accurate and relevant answers from a broad array of topics.
3. Knowledge Base Question Answering
Next, we move to Knowledge Base Question Answering, where the system answers questions based on a structured knowledge base. This is different from the previous model because it retrieves information from a more structured data set rather than general text.
model = build_model('kbqa_cq_en', download=True, install=True) questions = ['What is the currency of Sweden?', 'When did the Korean War end?'] answers, answer_ids, query = model(questions) print(answers)
Explanation:
'kbqa_cq_en'
: This is a pre-trained model that uses a knowledge base (not general text) to answer questions.model(questions)
: Like in the previous example, we input a set of questions and the model returns answers based on the knowledge base.
Expected Output:
['Swedish krona', '27 July 1953']
Real-World Application:
- This type of model is useful in scenarios where you need highly reliable, fact-based answers that don’t require broad context understanding, such as in FAQ systems, knowledge management systems, and chatbots for specific industries like banking or healthcare.
4. Text Classification (Sentiment Analysis, Insult Detection, etc.)
DeepPavlov also offers Text Classification models that allow you to analyze text and categorize it into predefined classes. This functionality can be used for tasks such as sentiment analysis, insult detection, and more.
model = build_model('insults_kaggle_bert', download=True, install=True) phrases = ['You are kind of stupid', 'You are a wonderful person!'] labels = model(phrases) print(labels)
Explanation:
insults_kaggle_bert
: This is a pre-trained BERT model designed to detect insults in text. It classifies each input phrase as either an insult or not.model(phrases)
: We input two phrases, and the model returns a classification label for each.
Expected Output:
['Insult', 'Not Insult']
Real-World Application:
- This functionality is highly useful in moderation systems that automatically detect offensive content in user-generated posts (e.g., on social media or forums). It can also be applied in customer feedback analysis to identify negative comments.
5. Spelling Correction
A common use case in NLP is Spelling Correction, where the system automatically detects and corrects misspelled words. This is crucial for improving the quality of user input and ensuring proper communication.
model = build_model('brillmoore_wikitypos_en', download=True, install=True) phrases_w_typos = ['I think this is the begining of a beautifull frendship.', "I'll be bak"] correct_phrases = model(phrases_w_typos) print(correct_phrases)
Explanation:
brillmoore_wikitypos_en
: A pre-trained model that detects spelling mistakes using a dataset of common English errors.model(phrases_w_typos)
: We pass in a list of phrases containing typos, and the model returns the corrected versions.
Expected Output:
['i think this is the beginning of a beautiful friendship.', "i'll be back"]
Real-World Application:
- Spell checkers in word processors, search engines, and chatbots benefit from such models, ensuring that users' input is more accurate and aligned with the system’s expectations.
6. Text Question Answering
Here, we look at Text Question Answering, where the system answers questions based on a provided context, extracting information directly from the text.
model = build_model('squad_bert', download=True, install=True) contexts = ['DeepPavlov is a library for NLP and dialog systems.', 'All work and no play makes Jack a dull boy'] questions = ['What is DeepPavlov?', 'What makes Jack a dull boy?'] answer, answers_start_idx, score = model(contexts, questions) print(answer)
Explanation:
squad_bert
: A model trained on the Stanford Question Answering Dataset (SQuAD), which excels at answering questions based on a given context.model(contexts, questions)
: The model uses the provided contexts to extract answers to the corresponding questions.
Expected Output:
['a library for NLP and dialog systems', 'All work and no play']
Real-World Application:
- This approach is perfect for document-based question answering systems, such as those used in legal or academic research where users need quick answers from large bodies of text.
7. Entity Extraction
Entity extraction involves identifying key entities (e.g., people, places, organizations) in a given text. This is a foundational task in many NLP applications.
from deeppavlov import build_model import nltk nltk.download('punkt_tab') model = build_model('entity_extraction_en', download=True, install=True) phrases = ['Forrest Gump is a comedy-drama film directed by Robert Zemeckis and written by Eric Roth.'] entity_substr, tags, entity_offsets, entity_ids, entity_conf, entity_pages, entity_labels = model(phrases) print(entity_substr, tags, entity_ids, entity_labels, sep='\n')
Explanation:
entity_extraction_en
: This model extracts named entities (e.g., people, organizations, locations) from a text.model(phrases)
: The model returns various outputs such as entity substrings (the entities found), their tags (e.g., PERSON, ORGANIZATION), and IDs (e.g., from Wikidata).
Expected Output:
[['forrest gump', 'robert zemeckis', 'eric roth']] [['WORK_OF_ART', 'PERSON', 'PERSON']] [[['Q134773', 'Q552213', 'Q12016774'], ['Q187364', 'Q36951156'], ['Q942932', 'Q89320386', 'Q89909683']]] [[['Forrest Gump', 'Forrest Gump', 'Forrest Gump'], ['Robert Zemeckis', 'Welcome to Marwen'], ['Eric Roth', 'Eric Roth', 'Eric W Roth']]]
Real-World Application:
- Named Entity Recognition (NER) is essential for building systems that need to analyze and organize content by entities, such as in news aggregation platforms, knowledge graphs, or legal document analysis.
8. Named Entity Recognition (NER)
NER, or Named Entity Recognition, is used to classify entities (such as names of people, organizations, or places) in text.
from deeppavlov import build_model model = build_model('ner_ontonotes_bert', download=True, install=True) phrases = ['Bob Ross lived in Florida', 'Elon Musk founded Tesla'] tokens, tags = model(phrases) print(tokens, tags, sep='\n')
Explanation:
ner_ontonotes_bert
: A BERT-based model trained on the OntoNotes dataset that recognizes named entities.model(phrases)
: The model tokenizes the input phrases and assigns tags to each token, indicating whether it represents a person, location, organization, or other entity.
Expected Output:
[['Bob', 'Ross', 'lived', 'in', 'Florida'], ['Elon', 'Musk', 'founded', 'Tesla']] [['B-PERSON', 'I-PERSON', 'O', 'O', 'B-GPE'], ['B-PERSON', 'I-PERSON', 'O', 'B-ORG']]
Real-World Application:
- NER is key for information extraction from documents, enhancing the capability of search engines, data extraction pipelines, and content categorization systems.
Conclusion:
DeepPavlov is an incredibly versatile framework that can help you build advanced conversational AI systems for a wide range of NLP tasks. From question answering to entity extraction and sentiment analysis, DeepPavlov offers pre-trained models that can be fine-tuned or directly used in real-world applications. The framework’s ease of use and flexibility make it an invaluable tool for developers working in AI, chatbots, customer service automation, and content analysis.
As you continue to explore DeepPavlov, experiment with the various models and fine-tune them for your specific needs. By doing so, you’ll be able to create robust AI systems capable of handling a variety of real-world challenges.
Resources Section:
---------------------------
Stay Updated:- Follow Build Fast with AI pages for all the latest AI updates and resources.
Experts predict 2025 will be the defining year for Gen AI implementation.Want to be ahead of the curve?
Join Build Fast with AI’s Gen AI Launch Pad 2025 - your accelerated path to mastering AI tools and building revolutionary applications.
---------------------------
Resources and Community
Join our community of 12,000+ AI enthusiasts and learn to build powerful AI applications! Whether you're a beginner or an experienced developer, this tutorial will help you understand and implement AI agents in your projects.
- Website: www.buildfastwithai.com
- LinkedIn: linkedin.com/company/build-fast-with-ai/
- Instagram: instagram.com/buildfastwithai/
- Twitter: x.com/satvikps
- Telegram: t.me/BuildFastWithAI