ChatArena: Build and Simulate Multi-Agent AI Environments
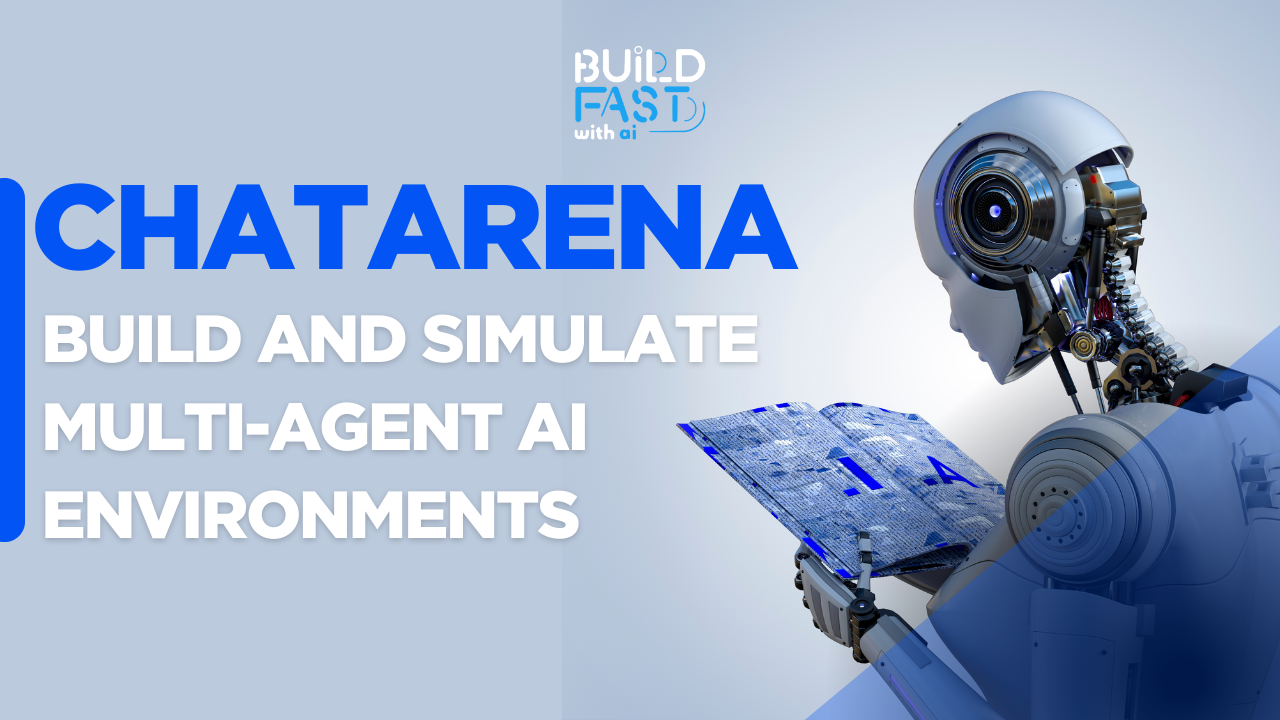
Will you regret not taking action, or be proud of what you’ve built?
Gen AI Launch Pad 2025 awaits your vision.
Introduction
In the ever-evolving world of artificial intelligence, the ability of language models to engage in multi-agent interactions is a crucial area of research. ChatArena is a powerful framework designed for multi-agent language game environments, enabling researchers and developers to simulate, benchmark, and improve the behavior of autonomous LLM agents in social interactions.
This blog post will explore ChatArena’s key features, walk through the provided code, explain each component, and demonstrate how to use it to create an AI-powered bargaining game. By the end, you'll have a solid grasp of how ChatArena structures interactions using Markov Decision Processes (MDP) and how you can apply it to various LLM-based research and applications.
What is ChatArena?
ChatArena provides a structured framework for modeling agents, environments, and interactions. It offers:
- Abstraction: A flexible way to define agents and their decision-making processes.
- Pre-built Language Game Environments: Ready-to-use environments for training, benchmarking, and evaluating LLM-based agents.
- User-friendly Interfaces: Supports both Web UI and CLI, making it easier to interact with the environment and fine-tune prompts.
Now, let’s break down the provided code and understand how to set up and simulate a bargaining game.
Installing Dependencies
To begin, install the required dependencies:
!pip install chatarena[all] !pip install openai==0.27.2
These commands install ChatArena along with all necessary dependencies and specify a compatible version of the OpenAI library.
Setting Up the OpenAI API Key
To use OpenAI’s models for agent interactions, set up the API key:
from google.colab import userdata import os os.environ['OPENAI_API_KEY'] = userdata.get('OPENAI_API_KEY')
Key Explanation:
- Uses
google.colab.userdata
to securely fetch the API key. - Stores the API key in an environment variable for authentication.
Creating a Buyer Agent
In our bargaining game, we need a buyer agent that negotiates for a lower price.
from chatarena.agent import Player from chatarena.backends import OpenAIChat buyer_role_description = """ You are a buyer in a Bargaining game. Your goal is to negotiate the price down while ensuring an agreement. Your negotiation strategy should: 1. Push the price lower for higher rewards. 2. Avoid exceeding your upper limit, or you’ll receive a negative reward. """ format_specification = """ Your response should be in JSON format: { "price": <your proposed price>, "arguments": "<your negotiation arguments>" } """ buyer = Player(name="buyer", role_desc=buyer_role_description+format_specification, backend=OpenAIChat(model="gpt-3.5-turbo"))
Explanation:
- Defines a buyer agent with a role description.
- The agent must negotiate within an upper price limit.
- Uses OpenAI’s GPT-3.5 Turbo model for responses.
- Returns a JSON-formatted response with a proposed price and justification.
Expected Output:
When prompted, the buyer might respond:
{ "price": 50, "arguments": "I believe a price of $50 is fair based on market value." }
Creating a Seller Agent
The seller agent follows a similar setup but aims to push the price higher.
seller_role_description = """ You are a seller in a Bargaining game. Your goal is to negotiate the price higher while ensuring an agreement. Your negotiation strategy should: 1. Push the price up for higher rewards. 2. Avoid going below your lower limit. """ seller = Player(name="seller", role_desc=seller_role_description+format_specification, backend=OpenAIChat(model="gpt-3.5-turbo"))
Key Differences:
- The seller agent aims to maximize the price.
- It has a lower price limit, below which it incurs a loss.
- Uses GPT-3.5 Turbo as its backend model.
Implementing the Bargaining Game Environment
The Bargaining Environment simulates the negotiation process between the buyer and seller.
from chatarena.environments.base import TimeStep, Environment from chatarena.message import Message, MessagePool from chatarena.utils import extract_jsons class Bargaining(Environment): def __init__(self, item_name, upper_limit, lower_limit, max_turn, unit="$"): super().__init__(player_names=["buyer", "seller"]) self.item_name = item_name self.upper_limit = upper_limit self.lower_limit = lower_limit self.max_turn = max_turn self.unit = unit self.turn = 0 self.message_pool = MessagePool()
Explanation:
Bargaining
Class: Defines the environment for negotiation.- Tracks:
- Item being negotiated.
- Upper and lower price limits.
- Number of turns allowed.
- Messages exchanged between players.
The environment updates messages, validates player actions, and determines when an agreement is reached.
Running the Bargaining Game
To launch the game:
from chatarena.arena import Arena env = Bargaining(item_name="diamond", upper_limit=500, lower_limit=100, max_turn=4) arena = Arena([buyer, seller], env) arena.launch_cli(interactive=False)
Key Features:
- Creates an Arena where the agents interact.
- Runs the negotiation game for 4 turns.
- Determines if an agreement is reached based on price proposals.
Upgrading to GPT-4
For improved negotiation, switch to GPT-4:
gpt4_buyer = Player(name="buyer", role_desc=buyer_role_description+format_specification, backend=OpenAIChat(model="gpt-4-0613")) gpt4_seller = Player(name="seller", role_desc=seller_role_description+format_specification, backend=OpenAIChat(model="gpt-4-0613")) env = Bargaining(item_name="diamond", upper_limit=500, lower_limit=100, max_turn=4) arena = Arena([gpt4_buyer, gpt4_seller], env) arena.launch_cli(interactive=False)
Why GPT-4?
- More advanced negotiation capabilities.
- Generates more coherent arguments.
- Simulates real-world bargaining scenarios more effectively.
Conclusion
ChatArena is a powerful tool for simulating multi-agent LLM environments. In this guide, we explored how to:
- Set up agents for a bargaining game.
- Define a game environment with price constraints.
- Simulate negotiations and analyze outcomes.
- Upgrade to GPT-4 for better performance.
Resources
- ChatArena GitHub Repo
- OpenAI API Documentation
- Markov Decision Process in AI
- ChatArena Experiment Notebook
---------------------------
Stay Updated:- Follow Build Fast with AI pages for all the latest AI updates and resources.
Experts predict 2025 will be the defining year for Gen AI Implementation. Want to be ahead of the curve?
Join Build Fast with AI’s Gen AI Launch Pad 2025 - your accelerated path to mastering AI tools and building revolutionary applications.
---------------------------
Resources and Community
Join our community of 12,000+ AI enthusiasts and learn to build powerful AI applications! Whether you're a beginner or an experienced developer, this tutorial will help you understand and implement AI agents in your projects.
- Website: www.buildfastwithai.com
- LinkedIn: linkedin.com/company/build-fast-with-ai/
- Instagram: instagram.com/buildfastwithai/
- Twitter: x.com/satvikps
- Telegram: t.me/BuildFastWithAI