Camel AI: Mastering Task Automation and Role-Playing
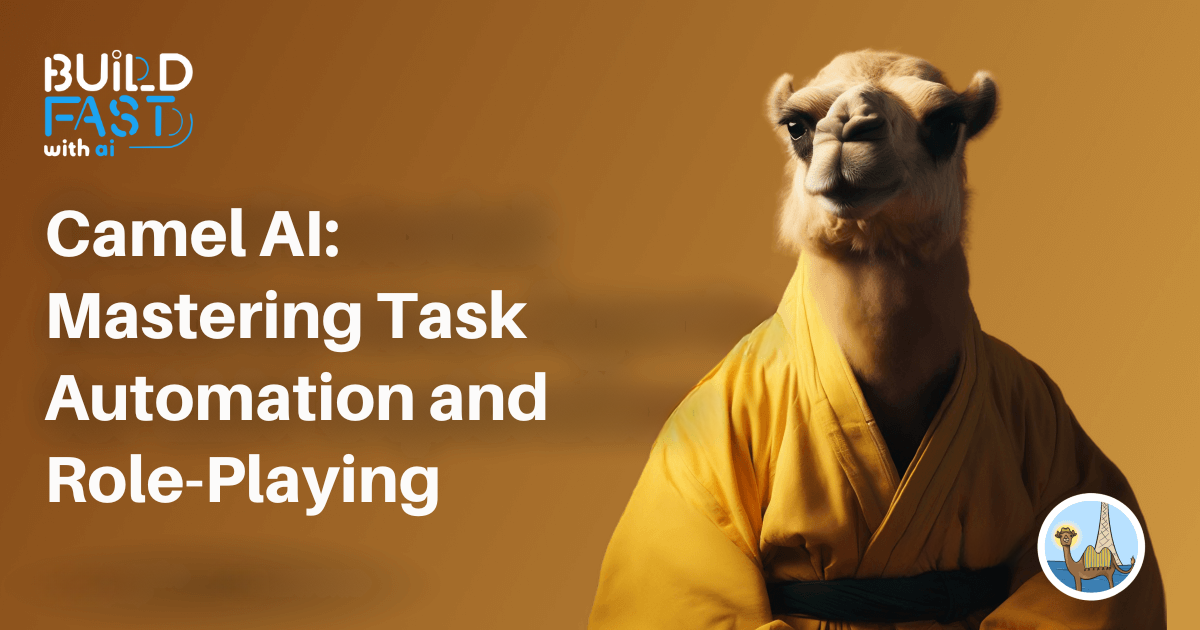
Will you stand by as the future unfolds, or will you seize the opportunity to create it?
Be part of Gen AI Launch Pad 2025 and take control.
Introduction
In this blog post, we’ll explore Camel AI, an open-source platform designed to enhance task automation, role-playing simulations, and collaborative problem-solving using Large Language Models (LLMs). Whether you're a developer, researcher, or AI enthusiast, this notebook provides a hands-on guide to leveraging Camel AI for creative projects, research, and real-world applications.
By the end of this blog, you’ll learn:
- How to set up and use Camel AI for role-playing simulations.
- How to automate complex tasks like vacation planning and trading bot development.
- How to convert ShareGPT conversations into Camel AI messages for seamless integration.
- Practical applications of Camel AI in real-world scenarios.
Let’s dive into the code and explore its capabilities step by step!
Detailed Explanation
1. Setting Up Camel AI
The first step is to install the Camel AI library and configure the environment.
Code Snippet:
!pip install camel-ai import os from google.colab import userdata # Set up OpenAI API key os.environ["OPENAI_API_KEY"] = userdata.get('OPENAI_API_KEY')
Explanation:
!pip install camel-ai
: Installs the Camel AI library.os.environ["OPENAI_API_KEY"]
: Sets the OpenAI API key as an environment variable. This is required for Camel AI to interact with LLMs.userdata.get('OPENAI_API_KEY')
: Retrieves the API key securely from Google Colab’s user data.
Expected Output:
No visible output, but the environment is now ready to use Camel AI.
Real-World Application:- This setup is essential for any project involving Camel AI. It ensures that the platform can access the necessary APIs to generate intelligent responses.
2. Vacation Planning Simulation
Camel AI excels at role-playing simulations. Here, we simulate a conversation between a Travel Planner and a Tourist to plan a 7-day vacation in Paris.
Code Snippet:
from colorama import Fore from camel.societies import RolePlaying from camel.utils import print_text_animated # Define the task prompt task_prompt = "Plan a 7-day vacation to Paris for a tourist" print(Fore.YELLOW + f"Original task prompt:\n{task_prompt}\n") # Initialize the role-playing session role_play_session = RolePlaying("Travel Planner", "Tourist", task_prompt=task_prompt) print(Fore.CYAN + f"Specified task prompt:\n{role_play_session.task_prompt}\n") # Simulate the conversation chat_turn_limit, n = 5, 0 input_msg = role_play_session.init_chat() while n < chat_turn_limit: n += 1 assistant_response, user_response = role_play_session.step(input_msg) print_text_animated(Fore.BLUE + f"Tourist:\n\n{user_response.msg.content}\n") print_text_animated(Fore.GREEN + "Travel Planner:\n\n" f"{assistant_response.msg.content}\n") if "CAMEL_TASK_DONE" in user_response.msg.content: break input_msg = assistant_response.msg
Explanation:
RolePlaying("Travel Planner", "Tourist", task_prompt=task_prompt)
: Initializes a role-playing session between two agents.print_text_animated
: Displays the conversation in an animated format for better readability.chat_turn_limit
: Limits the number of conversation turns to 5.CAMEL_TASK_DONE
: A keyword to indicate the task is complete.
Expected Output:-
The output will show a conversation between the Travel Planner and Tourist, with the Travel Planner providing detailed recommendations for each day of the vacation.
Real-World Application:-
This simulation can be used in travel agencies, customer service bots, or personal trip planning tools to automate itinerary creation.
3. Chat Agent Role Simulation
Camel AI can also simulate diverse roles and occupations. Here, we generate a list of 50 common internet user groups or occupations.
Code Snippet:
from camel.agents import ChatAgent from camel.messages import BaseMessage from camel.prompts import PromptTemplateGenerator from camel.types import TaskType def main(key: str = 'generate_users', num_roles: int = 50, model=None): prompt_template = PromptTemplateGenerator().get_prompt_from_key(TaskType.AI_SOCIETY, key) prompt = prompt_template.format(num_roles=num_roles) print(prompt) assistant_sys_msg = BaseMessage.make_assistant_message(role_name="Assistant", content="You are a helpful assistant.") agent = ChatAgent(assistant_sys_msg, model=model) agent.reset() user_msg = BaseMessage.make_user_message(role_name="User", content=prompt) assistant_response = agent.step(user_msg) print(assistant_response.msg.content) if __name__ == "__main__": main()
Explanation:
PromptTemplateGenerator
: Generates a prompt template for the task.ChatAgent
: Simulates a chat agent that generates the list of roles.BaseMessage.make_assistant_message
: Creates a system message for the assistant.
Expected Output:
A list of 50 roles, such as:
- Accountant
- Artist
- Blogger
- ...
- Volunteer
Real-World Application:- This feature is useful for creating datasets, training models, or simulating diverse user interactions in applications like chatbots or virtual assistants.
4. ShareGPT to CAMEL Message Conversion
Camel AI supports converting ShareGPT conversations into its own message format for seamless integration.
Code Snippet:
from typing import List from camel.messages import BaseMessage, HermesFunctionFormatter from camel.messages.conversion import ShareGPTConversation, ShareGPTMessage from camel.messages.func_message import FunctionCallingMessage def sharegpt_to_camel_messages(conversation: ShareGPTConversation) -> List[BaseMessage]: return [BaseMessage.from_sharegpt(msg, HermesFunctionFormatter()) for msg in conversation] def camel_messages_to_sharegpt(messages: List[BaseMessage]) -> ShareGPTConversation: sharegpt_messages = [msg.to_sharegpt(HermesFunctionFormatter()) for msg in messages] return ShareGPTConversation.model_validate(sharegpt_messages) # Example usage if __name__ == "__main__": # Create a sample ShareGPT conversation sharegpt_conv = ShareGPTConversation.model_validate([ ShareGPTMessage(from_="system", value="You are a helpful assistant."), ShareGPTMessage(from_="human", value="What's Tesla's P/E ratio?"), ShareGPTMessage(from_="gpt", value="Let me check Tesla's stock fundamentals.\n{'name': 'get_stock_fundamentals', 'arguments': {'symbol': 'TSLA'}}\n"), ShareGPTMessage(from_="tool", value='{"name": "get_stock_fundamentals", "content": {"symbol": "TSLA", "company_name": "Tesla, Inc.", "sector": "Consumer Cyclical", "pe_ratio": 49.604652}}'), ShareGPTMessage(from_="gpt", value="Tesla (TSLA) currently has a P/E ratio of 49.60."), ]) # Convert to CAMEL messages camel_messages = sharegpt_to_camel_messages(sharegpt_conv) for msg in camel_messages: print(f"Role: {msg.role_name}") print(f"Content: {msg.content}") if isinstance(msg, FunctionCallingMessage): print(f"Function Name: {msg.func_name}") if msg.args: print(f"Arguments: {msg.args}") if msg.result: print(f"Result: {msg.result}") print() # Convert back to ShareGPT converted_back = camel_messages_to_sharegpt(camel_messages) for msg in converted_back: print(f"From: {msg.from_}") print(f"Value: {msg.value}") print()
Explanation:
sharegpt_to_camel_messages
: Converts ShareGPT messages to Camel AI format.camel_messages_to_sharegpt
: Converts Camel AI messages back to ShareGPT format.FunctionCallingMessage
: Handles function calls within the conversation.
Expected Output:- The output will show the converted messages in both Camel AI and ShareGPT formats.
Real-World Application:- This functionality is useful for integrating Camel AI with existing chatbot frameworks or APIs that use ShareGPT.
Conclusion
Camel AI is a versatile platform for automating tasks, simulating role-playing scenarios, and integrating with other AI frameworks. By following this notebook, you’ve learned how to:
- Set up Camel AI and configure the environment.
- Simulate conversations for vacation planning and role-playing.
- Convert ShareGPT messages to Camel AI format.
These capabilities make Camel AI a powerful tool for developers, researchers, and businesses looking to leverage AI for intelligent automation.
Resources Section
- Camel AI GitHub Repository
- OpenAI API Documentation
- ShareGPT Documentation
- Python’s
yfinance
Library - Getting Started with Camel AI
- Camel AI Build Fast With AI Notebook
---------------------------
Stay Updated:- Follow Build Fast with AI pages for all the latest AI updates and resources.
Experts predict 2025 will be the defining year for Gen AI Implementation. Want to be ahead of the curve?
Join Build Fast with AI’s Gen AI Launch Pad 2025 - your accelerated path to mastering AI tools and building revolutionary applications.
---------------------------
Resources and Community
Join our community of 12,000+ AI enthusiasts and learn to build powerful AI applications! Whether you're a beginner or an experienced developer, this tutorial will help you understand and implement AI agents in your projects.
- Website: www.buildfastwithai.com
- LinkedIn: linkedin.com/company/build-fast-with-ai/
- Instagram: instagram.com/buildfastwithai/
- Twitter: x.com/satvikps
- Telegram: t.me/BuildFastWithAI