TextGrad: Optimizing AI-Generated Text with Gradient-Based Techniques
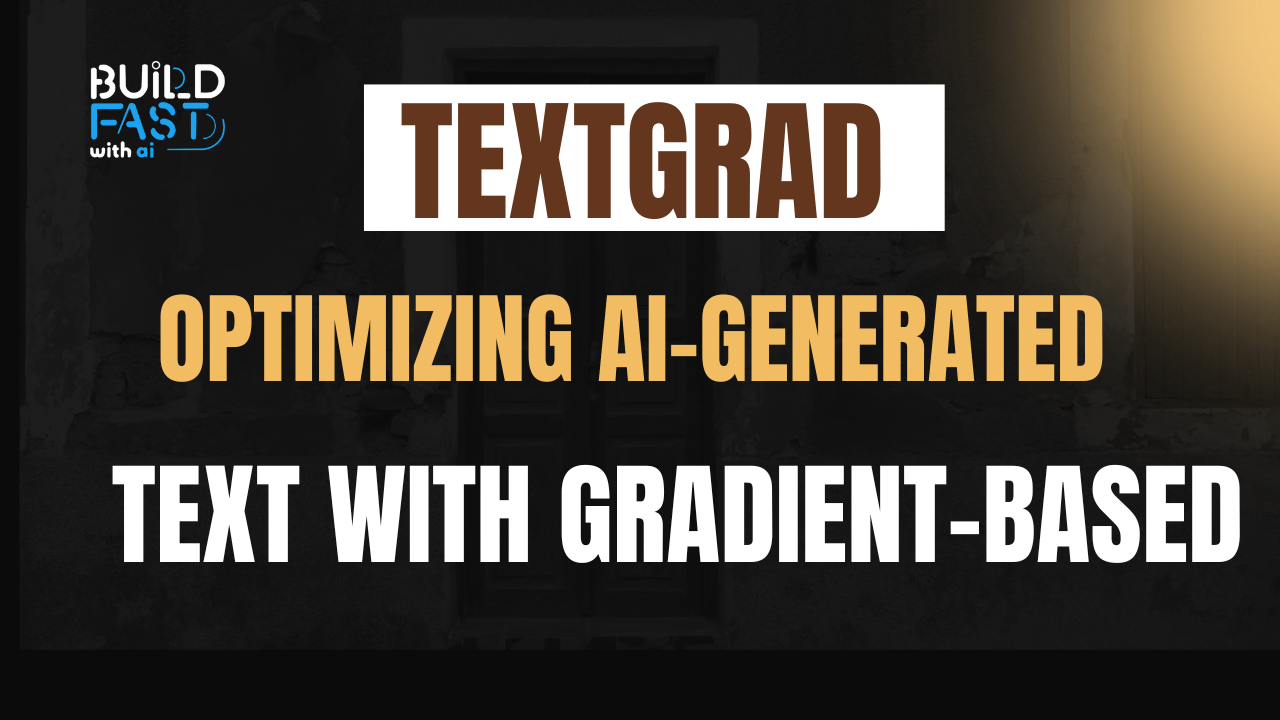
Will you look back and wish you acted, or look forward knowing you did?
Gen AI Launch Pad 2025 is your moment to build what’s next.
Introduction
With the rise of Generative AI, optimizing text output has become a critical challenge. Whether it's improving model robustness, fine-tuning text embeddings, or generating adversarial text examples, gradient-based optimization techniques offer a powerful approach. Enter TextGrad, an open-source library that applies differentiable optimization methods to manipulate text efficiently.
This blog post will provide a detailed walkthrough of TextGrad, explaining key concepts and demonstrating its real-world applications. You will learn:
- How TextGrad optimizes text generation through gradient-based methods.
- How to install and set up the library.
- How to optimize answers generated by GPT-4o.
- How to refine mathematical solutions using gradient descent.
- How to perform multimodal optimization (text & image-based QA).
Getting Started with TextGrad
Installation
To start using TextGrad, install the package using pip:
pip install textgrad
Setting Up API Keys
Since TextGrad relies on GPT-4o, you'll need to set up API keys:
from google.colab import userdata import os os.environ['OPENAI_API_KEY'] = userdata.get('OPENAI_API_KEY')
This ensures secure access to OpenAI's API for text optimization tasks.
Optimizing Text Generation with TextGrad
TextGrad enables users to optimize text outputs by treating text as a differentiable object, which allows for fine-grained text modifications using gradient descent.
Let's see how we can optimize a question-answering task using GPT-4o.
Using GPT-4o for Question Answering
Code Implementation
import textgrad as tg tg.set_backward_engine("gpt-4o", override=True) model = tg.BlackboxLLM("gpt-4o") question_string = "If it takes 1 hour to dry 25 shirts under the sun, how long will it take to dry 30 shirts?" question = tg.Variable(question_string, role_description="question to the LLM", requires_grad=False) answer = model(question)
Expected Output
The model generates an answer to the given question. However, this answer may not always be optimal. That's where TextGrad's optimization capabilities come in.
Optimizing the Answer with Gradient Descent
Defining an Evaluation and Loss Function
To improve the generated answer, we define a loss function that critically evaluates its quality.
Code Implementation
answer.set_role_description("concise and accurate answer to the question") optimizer = tg.TGD(parameters=[answer]) evaluation_instruction = ( f"Here's a question: {question_string}. Evaluate any given answer, be critical, and provide concise feedback." ) loss_fn = tg.TextLoss(evaluation_instruction)
Expected Output
The loss function provides feedback on the generated answer, which helps refine it through gradient descent.
Optimization with Gradient Descent
loss = loss_fn(answer) loss.backward() optimizer.step() answer
Now, the answer should be more precise and well-structured than the original output.
Optimizing Mathematical Solutions with TextGrad
Problem Statement
Let's take an example where we optimize a mathematical solution. The initial solution has errors:
initial_solution = """To solve the equation 3x^2 - 7x + 2 = 0, we use the quadratic formula: x = (-b ± √(b^2 - 4ac)) / 2a a = 3, b = -7, c = 2 x = (7 ± √((-7)^2 - 4 * 3(2))) / 6 x = (7 ± √(7^3) / 6 The solutions are: x1 = (7 + √73) x2 = (7 - √73)"""
Defining a Loss Function for Error Identification
solution = tg.Variable(initial_solution, requires_grad=True, role_description="solution to the math question") loss_fn = tg.TextLoss("Identify errors in this solution. Do not solve it, just critique.")
Optimizing with Gradient Descent
optimizer = tg.TGD(parameters=[solution]) loss = loss_fn(solution) loss.backward() optimizer.step() print(solution.value)
Expected Output
The model refines the mathematical solution by correcting errors and structuring the output properly.
Multimodal Image Question Answering with TextGrad
Processing Images with TextGrad
TextGrad can also be used for image-based question-answering tasks.
Downloading an Image from the Web
import httpx image_url = "https://upload.wikimedia.org/wikipedia/commons/a/a7/Camponotus_flavomarginatus_ant.jpg" image_data = httpx.get(image_url).content
Processing the Image in TextGrad
from PIL import Image import textgrad as tg image_variable = tg.Variable(image_data, role_description="image to answer a question about", requires_grad=False) question_variable = tg.Variable("What do you see in this image?", role_description="question", requires_grad=False)
Generating an Answer
from textgrad.autograd import MultimodalLLMCall response = MultimodalLLMCall("gpt-4o")([image_variable, question_variable])
Expected Output
The response provides a detailed description of the image.
Conclusion
TextGrad offers a powerful gradient-based approach to text optimization. By treating text as a differentiable object, it enables fine-tuned control over:
- Text generation for NLP models.
- Mathematical solution refinement.
- Adversarial text generation.
- Multimodal interactions (text & image QA).
This library is valuable for researchers, engineers, and developers working in NLP, adversarial AI, and generative models.
Resources
- TextGrad GitHub Repository
- OpenAI API Documentation
- Gradient Descent for Text Optimization
- TextGrad Build Fast with AI Experiment Notebook
---------------------------
Stay Updated:- Follow Build Fast with AI pages for all the latest AI updates and resources.
Experts predict 2025 will be the defining year for Gen AI Implementation. Want to be ahead of the curve?
Join Build Fast with AI’s Gen AI Launch Pad 2025 - your accelerated path to mastering AI tools and building revolutionary applications.
---------------------------
Resources and Community
Join our community of 12,000+ AI enthusiasts and learn to build powerful AI applications! Whether you're a beginner or an experienced developer, our resources will help you understand and implement Generative AI in your projects.
- Website: www.buildfastwithai.com
- LinkedIn: linkedin.com/company/build-fast-with-ai/
- Instagram: instagram.com/buildfastwithai/
- Twitter: x.com/satvikps
- Telegram: t.me/BuildFastWithAI