Supabase for Generative AI: How to Store, Search & Manage AI Content with pgvector
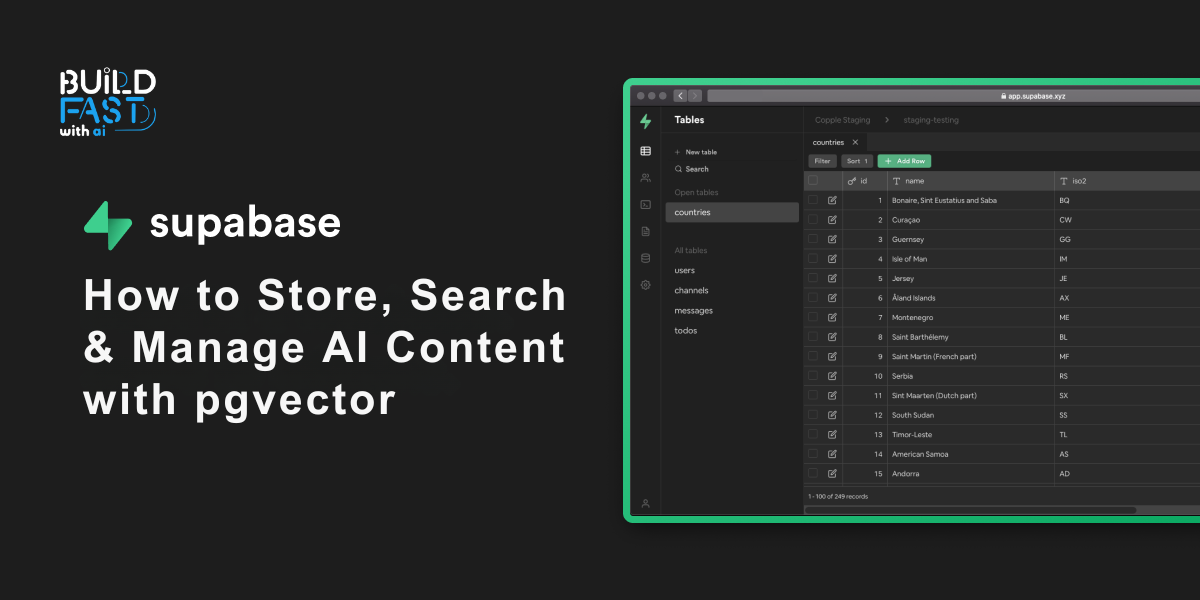
Are you content watching others shape the future, or will you take charge?
Be part of Gen AI Launch Pad 2025 and make your mark today.
Introduction
In the era of artificial intelligence, efficient data storage, retrieval, and management are crucial for building powerful GenAI applications. Supabase, an open-source backend-as-a-service (BaaS), provides scalable real-time databases, authentication, and edge functions, making it an excellent choice for GenAI developers.
In this guide, we’ll explore how to leverage Supabase for tasks like embedding storage, similarity search, and retrieval-augmented generation (RAG). You’ll learn how to:
- Set up Supabase for AI projects
- Store AI-generated content efficiently
- Perform vector similarity searches with
pgvector
- Manage user authentication and real-time streaming
By the end, you'll have a solid understanding of integrating Supabase with OpenAI and LangChain to build intelligent applications.
Setting Up Supabase and OpenAI Credentials
Install Required Libraries
To begin, install the necessary Python libraries for interacting with Supabase and handling AI embeddings.
!pip install supabase python-dotenv openai langchain sentence-transformers tiktoken langchain-community
Import Required Libraries
import os from supabase import create_client, Client from openai import OpenAI from langchain.embeddings import OpenAIEmbeddings
Configure Supabase and OpenAI API Keys
from google.colab import userdata import numpy as np SUPABASE_URL = userdata.get('SUPABASE_URL') SUPABASE_KEY = userdata.get('SUPABASE_KEY') OPENAI_API_KEY = userdata.get('OPENAI_API_KEY') os.environ['OPENAI_API_KEY'] = OPENAI_API_KEY supabase: Client = create_client(SUPABASE_URL, SUPABASE_KEY) embeddings_model = OpenAIEmbeddings(openai_api_key=OPENAI_API_KEY)
📌 What’s Happening Here?
- The script installs and imports key libraries for database interactions and AI processing.
- Supabase API keys are retrieved securely from Colab’s
userdata
. - OpenAI’s embedding model is initialized for vector storage and similarity search.
Creating an AI Content Table in Supabase
Step 1: Create the ai_content
Table
Open the SQL Editor in Supabase and run the following:
CREATE TABLE ai_content ( id SERIAL PRIMARY KEY, created_at TIMESTAMPTZ DEFAULT NOW(), content TEXT NOT NULL );
Step 2: Enable Vector Extension & Add Embeddings Column
CREATE EXTENSION IF NOT EXISTS vector; ALTER TABLE ai_content ADD COLUMN embedding vector(1536);
Step 3: Add User-Specific Content Storage
ALTER TABLE ai_content ADD COLUMN user_id UUID REFERENCES auth.users(id);
📌 Why This Matters?
- The
ai_content
table stores AI-generated text. - The
embedding
column holds vector representations for similarity searches. - User IDs allow personalized AI content storage.
Storing AI-Generated Content with Embeddings
def store_with_embedding(text, user_id=None): """Generate embeddings for the given text and store them in Supabase""" embedding = embeddings_model.embed_query(text) # Generate embedding embedding_array = np.array(embedding).tolist() # Convert to list for storage data = {"content": text, "embedding": embedding_array} if user_id: data["user_id"] = user_id # Store user-specific content if provided response = supabase.table("ai_content").insert(data).execute() return response
📌 Breaking It Down:
- Converts input text into an embedding vector using OpenAI’s model.
- Inserts the content and its embedding into Supabase.
- Optionally stores the user ID for personalized content management.
Example Usage:
store_with_embedding("The quick brown fox jumps over the lazy dog.") store_with_embedding("Artificial Intelligence is transforming the world.") store_with_embedding("Machine learning is a subset of AI.")
Searching for Similar Content
def search_similar_content(query, top_k=5): """Search for similar content using the match_ai_content function""" query_embedding = embeddings_model.embed_query(query) response = supabase.rpc("match_ai_content", { "query_embedding": query_embedding, "match_count": top_k }).execute() return response
📌 How It Works?
- Converts the search query into an embedding vector.
- Calls the Supabase Remote Procedure Call (RPC) function
match_ai_content
. - Returns the top
k
most similar content items.
Example Search Query:
results = search_similar_content("Tell me about AI") print(results)
Expected Output:
[ {"content": "Artificial Intelligence is transforming the world.", "similarity": 0.88}, {"content": "Machine learning is a subset of AI.", "similarity": 0.85}, {"content": "The quick brown fox jumps over the lazy dog.", "similarity": 0.73} ]
Fetching & Managing AI Content
Fetch All Stored Content
response = supabase.table("ai_content").select("*").execute() print(response.data)
Insert a New Document
new_doc = {"content": "The AI revolution is transforming industries worldwide."} response = supabase.table("ai_content").insert(new_doc).execute() print(response)
Update an Existing Document
updated_doc = {"content": "Updated content about Artificial Intelligence and its applications."} response = supabase.table("ai_content").update(updated_doc).eq("id", 1).execute() print(response)
Delete a Document
response = supabase.table("ai_content").delete().eq("id", 1).execute() print(response)
📌 Why These Operations Matter?
- Fetching allows viewing stored AI content.
- Inserting enables adding new AI-generated insights.
- Updating ensures content relevance.
- Deleting removes unnecessary data.
Conclusion
In this guide, we covered how to:
- Set up Supabase for AI applications.
- Store AI-generated content with embeddings.
- Search for similar content using vector search.
- Manage data operations (insert, update, delete).
By integrating Supabase with OpenAI & LangChain, you can build robust retrieval-augmented generation (RAG) systems, AI-powered knowledge bases, and more!
Further Resources
- Supabase Documentation
- pgvector for PostgreSQL
- OpenAI Embeddings Guide
- LangChain Documentation
- Supabase Build Fast with AI Notebook
---------------------------
Stay Updated:- Follow Build Fast with AI pages for all the latest AI updates and resources.
Experts predict 2025 will be the defining year for Gen AI Implementation. Want to be ahead of the curve?
Join Build Fast with AI’s Gen AI Launch Pad 2025 - your accelerated path to mastering AI tools and building revolutionary applications.
---------------------------
Resources and Community
Join our community of 12,000+ AI enthusiasts and learn to build powerful AI applications! Whether you're a beginner or an experienced developer, this tutorial will help you understand and implement AI agents in your projects.
- Website: www.buildfastwithai.com
- LinkedIn: linkedin.com/company/build-fast-with-ai/
- Instagram: instagram.com/buildfastwithai/
- Twitter: x.com/satvikps
- Telegram: t.me/BuildFastWithAI