Redis for Generative AI: Fast Data & Vector Search
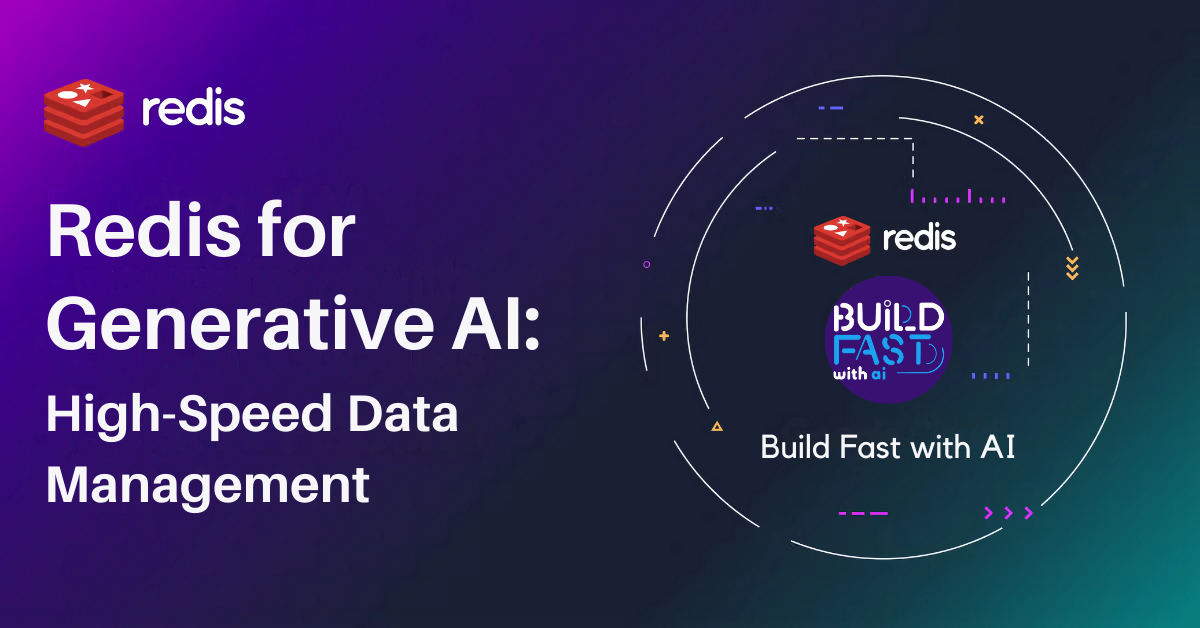
Are you willing to risk being left behind, or will you take action?
Join Gen AI Launch Pad 2025 and be the future.
Introduction
Generative AI models require efficient, high-speed data handling to optimize performance in real-time applications. Redis (Remote Dictionary Server), an in-memory key-value store, provides ultra-fast data storage and retrieval, making it ideal for AI-driven applications such as chatbots, recommendation systems, and real-time inference.
This blog will explore how Redis enhances Generative AI, covering fundamental operations, vector search, session management, and practical AI-driven use cases. Readers will learn how to install Redis, store and retrieve AI-related data, set up vector indexes for similarity search, and integrate Redis with AI applications. By the end of this guide, you will understand how Redis facilitates AI-powered workflows and improves application efficiency.
Setting Up Redis
Installing Redis
To begin, install the Redis library in a Jupyter Notebook or a Python environment using:
!pip install redis
This command installs the Redis client for Python, which allows interaction with a Redis server.
Connecting to Redis
from google.colab import userdata import redis Redis_host = userdata.get('Redis_host') Redis_password = userdata.get('Redis_password') redis_client = redis.Redis(host=Redis_host, port=17158, password=Redis_password, decode_responses=True) redis_client.ping() print("Connected to Redis successfully!")
Explanation:
redis.Redis()
: Initializes a Redis client to interact with the server.decode_responses=True
: Ensures data is returned as Python strings instead of byte objects.ping()
: Verifies that the connection to Redis is active.
Expected Output:
Connected to Redis successfully!
Why Use Redis for AI?
- High-Speed Access: Redis is an in-memory database, ensuring fast read/write operations.
- Scalability: Redis supports distributed architectures, handling high query loads efficiently.
- Persistence: While Redis is primarily in-memory, it provides options for persistence via snapshots or append-only files (AOF).
- AI-Friendly: Ideal for storing chat histories, embeddings, and vector search indexes for AI applications.
Data Storage and Retrieval in Redis
Storing and Retrieving User Context
if redis_client: key = "user_context:123" value = {"name": "Alice", "last_message": "Hello"} redis_client.hset(key, mapping=value) print(f"Stored data: {value} with key: {key}") retrieved_data = redis_client.hgetall(key) print(f"Retrieved data: {retrieved_data}") else: print("Redis client not initialized, skipping data operations.")
Explanation:
hset(key, mapping=value)
: Stores a hash (dictionary) in Redis.hgetall(key)
: Retrieves all key-value pairs from the hash.
Expected Output:
Stored data: {'name': 'Alice', 'last_message': 'Hello'} with key: user_context:123 Retrieved data: {'name': 'Alice', 'last_message': 'Hello'}
Use Case:
This is useful in AI chatbots where maintaining user session history improves response relevance.
Vector Indexing and Search in Redis
Creating a Vector Index
from redis.commands.search.field import TagField, VectorField from redis.commands.search.indexDefinition import IndexDefinition, IndexType INDEX_NAME = "vector_index" try: redis_client.ft(INDEX_NAME).dropindex(delete_documents=True) except: pass schema = [ TagField("id"), VectorField("vector", "HNSW", { "TYPE": "FLOAT32", "DIM": 128, "DISTANCE_METRIC": "COSINE" }) ] redis_client.ft(INDEX_NAME).create_index(fields=schema, definition=IndexDefinition(prefix=["vec:"], index_type=IndexType.HASH)) print("Vector index created successfully!")
Explanation:
- VectorField: Defines a searchable vector field for embeddings.
- HNSW (Hierarchical Navigable Small World): An efficient nearest-neighbor search algorithm.
- Cosine distance: Measures similarity between vectors.
Expected Output:
Vector index created successfully!
Use Case:
Used in Generative AI-powered recommendation systems and semantic search.
Performing KNN Search in Redis
from redis.commands.search.query import Query import numpy as np query_vector = np.random.rand(128).astype(np.float32) query = Query("*=>[KNN 5 @vector $vec AS score]").return_fields("id", "score").paging(0, 5).dialect(2) try: res = redis_client.ft(INDEX_NAME).search(query, query_params={"vec": query_vector.tobytes()}) if res.total == 0: print("No results found! Try inserting more vectors.") else: for doc in res.docs: print(f"ID: {doc.id}, Score: {doc.score}") except Exception as e: print("Search failed:", e)
Explanation:
- KNN (K-Nearest Neighbors) Search: Finds the most similar vectors.
ft(INDEX_NAME).search()
: Executes the nearest neighbor search query.
Expected Output:
ID: vec:item_4, Score: 0.210143089294 ID: vec:item_40, Score: 0.221729815006 ID: vec:item_56, Score: 0.210548818111 ID: vec:item_81, Score: 0.224302589893 ID: vec:item_90, Score: 0.217894077301
Use Case:
Essential for Generative AI applications like chatbots, recommendation engines, and similarity searches.
Conclusion
Redis provides a powerful, low-latency data store that significantly enhances Generative AI applications. From caching user contexts to executing fast similarity searches using vector indexes, Redis enables real-time AI applications to perform efficiently at scale.
Key Takeaways:
- Redis optimizes AI models by storing and retrieving data in sub-millisecond time.
- Vector search with Redis enables efficient similarity retrieval for Generative AI tasks.
- Storing chat histories and embeddings improves AI-driven personalization.
Next Steps:
- Explore Redis official documentation: Redis Documentation
- Learn about Redis Vector Search: Redis Search Guide
- Experiment with real-world AI applications using Redis:- Notebook with Code
---------------------------
Stay Updated:- Follow Build Fast with AI pages for all the latest AI updates and resources.
Experts predict 2025 will be the defining year for Gen AI Implementation. Want to be ahead of the curve?
Join Build Fast with AI’s Gen AI Launch Pad 2025 - your accelerated path to mastering AI tools and building revolutionary applications.
---------------------------
Resources and Community
Join our community of 12,000+ AI enthusiasts and learn to build powerful AI applications! Whether you're a beginner or an experienced developer, this tutorial will help you understand and implement AI agents in your projects.
- Website: www.buildfastwithai.com
- LinkedIn: linkedin.com/company/build-fast-with-ai/
- Instagram: instagram.com/buildfastwithai/
- Twitter: x.com/satvikps
- Telegram: t.me/BuildFastWithAI