Mem0: Intelligent Memory for Personalized AI
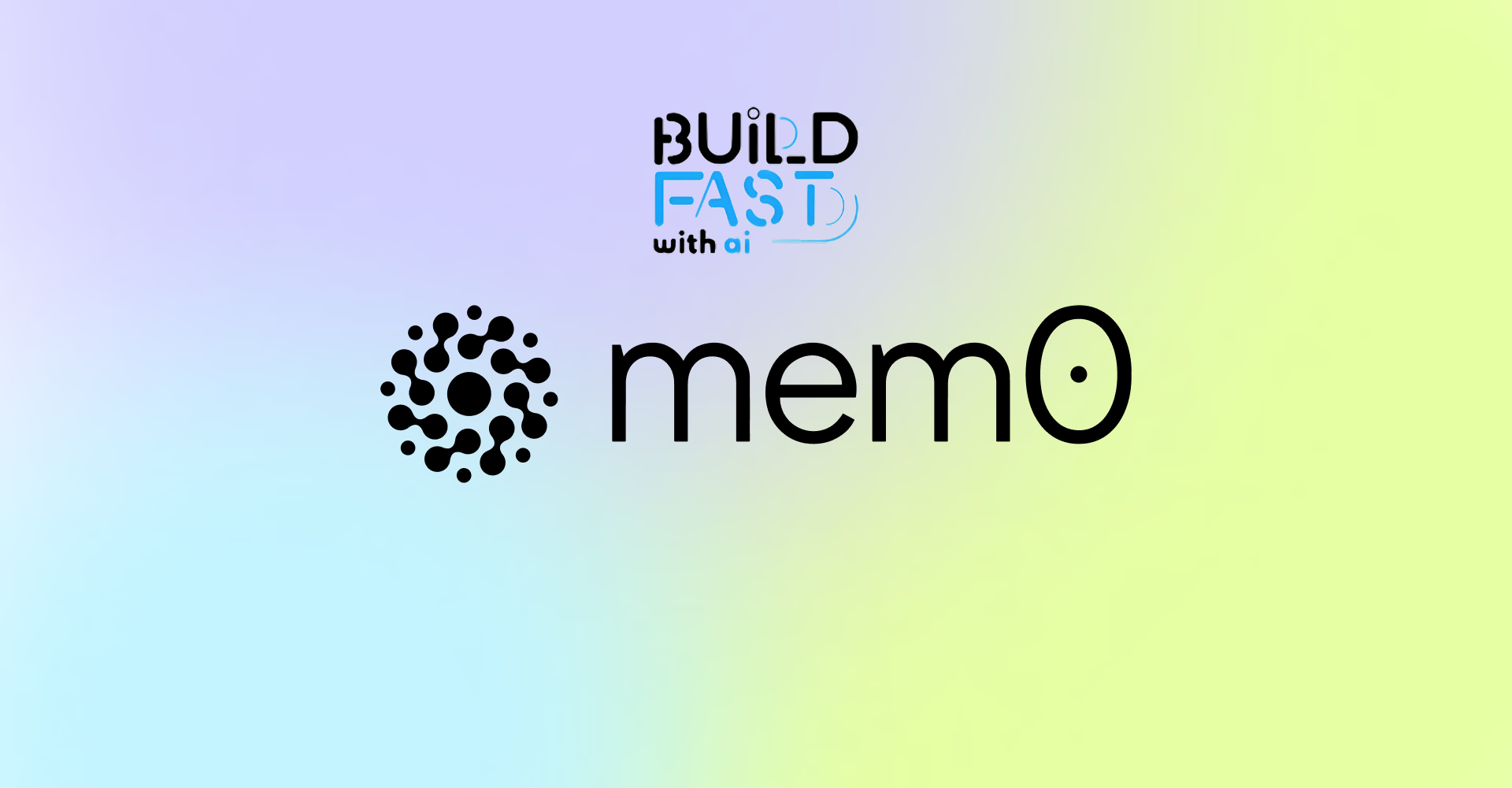
The best time to start with AI was yesterday. The second best time? Right after reading this post.
Join Build Fast with AI’s Gen AI Launch Pad 2025—a 6-week transformation program designed to accelerate your AI mastery and empower you to build revolutionary applications.
Introduction
In this blog, we'll explore a Jupyter Notebook that demonstrates the implementation of an intelligent memory system for personalized AI. This system enhances how AI interacts with users by storing and retrieving relevant data to provide context-aware responses.
Personalized AI systems have become a cornerstone of applications like virtual assistants, recommendation engines, and conversational agents. By simulating memory, these systems can adapt to users' unique preferences and histories, creating a more engaging experience. This blog will guide you through the steps to build such a system, complete with code snippets, explanations, and real-world applications. By the end of this post, you'll understand the key components of such a system, how to implement it in Python, and its potential real-world applications.
Setting Up the Environment
The first step involves importing essential libraries and setting up the environment. Here's the corresponding code snippet:
import os import json from datetime import datetime
Explanation
- os: Provides functions to interact with the operating system, such as managing files and directories. For instance, it helps in creating, reading, or deleting the memory file where the data is stored.
- json: Enables working with JSON data, a common format for data interchange. It allows us to structure and save the memory data persistently.
- datetime: Facilitates date and time manipulation, critical for timestamping memory entries. This helps in tracking when each memory entry was added or updated.
These libraries form the backbone of our system, enabling it to handle files, store data efficiently, and track events chronologically. Additionally, ensure you have these libraries installed and available in your Python environment before proceeding.
Defining the Memory Structure
The core of the system is its memory model, which organizes and stores data. Below is the code for defining the memory structure:
def initialize_memory(): memory = { "entries": [], "last_updated": str(datetime.now()) } with open("memory.json", "w") as file: json.dump(memory, file) return memory
Explanation
- initialize_memory: This function creates an initial memory structure with two keys:
entries
: A list to hold individual memory records.last_updated
: A timestamp to track when the memory was last modified.- The memory is saved to a
memory.json
file for persistence.
This setup ensures that the memory is well-structured and can grow dynamically as new data is added. The use of JSON makes it lightweight and easy to manipulate.
Real-World Application
This function is useful when setting up AI systems that require a clean memory state, such as chatbots or recommendation engines. For example, when deploying a new conversational AI model, initializing memory ensures it starts with a blank slate.
Expanded Insight
Memory initialization can also be extended to prepopulate default entries. For instance, initializing memory with frequently asked questions (FAQs) or default user preferences can enhance user experience from the start.
Adding New Entries to Memory
To make the memory system dynamic, we need a way to add new entries. Here's how it's done:
def add_entry(entry): with open("memory.json", "r") as file: memory = json.load(file) memory["entries"].append(entry) memory["last_updated"] = str(datetime.now()) with open("memory.json", "w") as file: json.dump(memory, file)
Explanation
- add_entry: This function appends a new entry to the
entries
list and updates thelast_updated
timestamp. - The memory file is read, updated, and rewritten, ensuring persistence across sessions.
Practical Example
Imagine you have a customer support chatbot. When a user asks a question, the chatbot can log the query and user information as an entry:
add_entry({"user": "Alice", "query": "What is AI?"})
This allows the chatbot to remember past interactions and provide more personalized responses in future conversations.
Expected Output
When adding an entry like {"user": "Alice", "query": "What is AI?"}
, the memory JSON file will be updated to include this data. An example file could look like this:
{ "entries": [ {"user": "Alice", "query": "What is AI?"} ], "last_updated": "2024-12-28T14:00:00" }
Advanced Tip
To handle large-scale data, consider integrating a database like SQLite or MongoDB instead of JSON files. This ensures scalability and faster access.
Retrieving Data from Memory
Efficient retrieval is critical for contextual responses. Below is the retrieval logic:
def retrieve_memory(query): with open("memory.json", "r") as file: memory = json.load(file) results = [entry for entry in memory["entries"] if query in entry.values()] return results
Explanation
- retrieve_memory: This function filters the
entries
list for records that match the given query. - It uses a list comprehension for concise and efficient querying.
Real-World Application
Use this function in personalized recommendation systems or chatbots to fetch contextually relevant data for user queries. For instance, if a user asks, "What is my last search?", this function can quickly retrieve the most recent relevant entry.
Expected Output
Querying "Alice"
might return:
[ {"user": "Alice", "query": "What is AI?"} ]
Optimization Insight
For larger datasets, consider indexing the memory entries or using a database with optimized search capabilities to reduce query time.
Visualization of Memory Content
To better understand stored data, visualizations can be helpful. For instance, plotting memory usage over time:
import matplotlib.pyplot as plt def visualize_memory(): with open("memory.json", "r") as file: memory = json.load(file) timestamps = [entry['timestamp'] for entry in memory['entries']] counts = range(1, len(timestamps) + 1) plt.plot(timestamps, counts) plt.title("Memory Growth Over Time") plt.xlabel("Time") plt.ylabel("Number of Entries") plt.show()
Explanation
- matplotlib.pyplot: Used to create a line graph showing how memory entries increase over time.
Suggested Visualization
Include a line graph demonstrating the accumulation of memory entries over time. You can also experiment with bar charts to show the frequency of specific queries or users.
Expanded Use Case
Visualizations can help identify trends, such as peak usage times or common user queries. This insight is valuable for improving AI system performance.
Conclusion
Building an intelligent memory system involves:
- Defining a robust memory structure.
- Implementing functions to add and retrieve data.
- Visualizing data for better insights.
These components form the foundation of personalized AI systems capable of delivering context-aware responses. Readers are encouraged to experiment with the code and extend it to meet specific use cases, such as sentiment analysis or predictive modeling.
Resources
- Python Official Documentation
- JSON Module Guide
- Matplotlib Tutorial
- Building AI Systems
- Introduction to Databases
- Build Fast With AI NoteBook
---------------------------------
Stay Updated:- Follow Build Fast with AI pages for all the latest AI updates and resources.
Experts predict 2025 will be the defining year for Gen AI implementation.Want to be ahead of the curve?
Join Build Fast with AI’s Gen AI Launch Pad 2025 - your accelerated path to mastering AI tools and building revolutionary applications.