Mastering Speech AI with NVIDIA NeMo: A Hands-On Guide
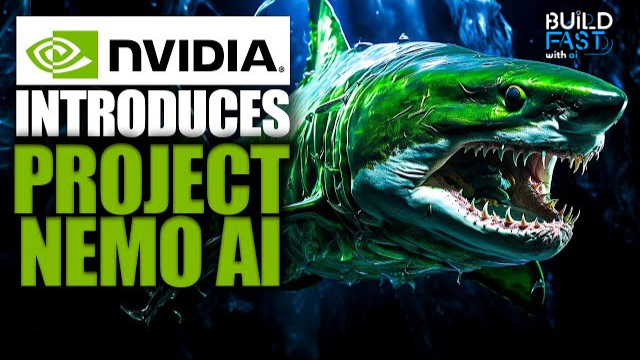
Will you let others shape the future for you, or will you lead the way?
Gen AI Launch Pad 2025 is your moment to shine.
Introduction
Speech AI has seen rapid advancements, and NVIDIA NeMo stands at the forefront of this evolution. NeMo provides a modular and scalable approach to building speech-related AI applications, including automatic speech recognition (ASR), text-to-speech (TTS), and speech classification. This guide will walk you through NeMo’s key features, code implementation, and real-world applications.
Getting Started with NeMo
Before diving into the code, ensure you have NVIDIA NeMo installed. If not, install it using the following command:
pip install nemo_toolkit[all]
Understanding the Code Blocks
1. Importing Required Libraries
To start, we need to import the essential libraries:
import nemo.collections.asr as nemo_asr import torch
Explanation:
nemo.collections.asr
: Provides prebuilt models and tools for automatic speech recognition.torch
: Used for deep learning computations.
2. Loading a Pretrained ASR Model
asr_model = nemo_asr.models.EncDecCTCModelBPE.from_pretrained(model_name="stt_en_conformer_ctc_large")
Explanation:
EncDecCTCModelBPE.from_pretrained
: Loads a pre-trained speech recognition model.stt_en_conformer_ctc_large
: A large English ASR model based on Conformer architecture.
Expected Output: The model will be downloaded and initialized, ready for inference.
3. Transcribing Audio
audio_file = "sample_audio.wav" transcription = asr_model.transcribe([audio_file]) print("Transcription:", transcription)
Explanation:
- The model takes an audio file and transcribes it into text.
- The output will be a list containing the transcribed text.
Expected Output:
Transcription: ['Hello, how are you?']
4. Training a Custom Model
To fine-tune the model, we need to set up training parameters:
import nemo.collections.asr as nemo_asr import pytorch_lightning as pl # Define a model model = nemo_asr.models.EncDecCTCModelBPE(cfg="/path/to/config.yaml") # Define a Trainer trainer = pl.Trainer(max_epochs=5, gpus=1) trainer.fit(model)
Explanation:
cfg
: Configuration file defining the model architecture and training parameters.pl.Trainer
: Handles training with PyTorch Lightning.max_epochs=5
: Runs training for 5 epochs.
5. Generating Speech (Text-to-Speech - TTS)
import nemo.collections.tts as nemo_tts # Load a TTS model tts_model = nemo_tts.models.FastPitchModel.from_pretrained("tts_en_fastpitch") text = "Hello, welcome to NVIDIA NeMo!" audio = tts_model.generate_speech(text)
Explanation:
tts_en_fastpitch
: A pretrained FastPitch TTS model.generate_speech(text)
: Converts text into synthesized speech.
6. Deploying a Model
To deploy a trained model, we can save and export it:
model.save_to("custom_asr_model.nemo")
To load the model later:
loaded_model = nemo_asr.models.EncDecCTCModelBPE.restore_from("custom_asr_model.nemo")
Explanation:
save_to
: Saves the trained model.restore_from
: Loads the model for inference.
Applications of NVIDIA NeMo
- Voice Assistants: Build AI-powered assistants like Siri or Google Assistant.
- Captioning Systems: Automate captioning for videos, improving accessibility.
- Call Center Automation: Enhance customer support through AI-driven call transcription.
- Language Learning: Assist users in pronunciation and language acquisition.
Conclusion
NVIDIA NeMo provides a powerful toolkit for developing Speech AI applications. Whether you’re working on ASR, TTS, or speech classification, NeMo simplifies development with pretrained models and modular design. Try implementing NeMo in your projects today!
Resources
- NVIDIA NeMo Documentation
- PyTorch Lightning
- Speech AI Research Papers
- NeMo Build Fast with AI Notebook
- NVIDIA NeMo GitHub
- Pretrained ASR Models
---------------------------
Stay Updated:- Follow Build Fast with AI pages for all the latest AI updates and resources.
Experts predict 2025 will be the defining year for Gen AI Implementation. Want to be ahead of the curve?
Join Build Fast with AI’s Gen AI Launch Pad 2025 - your accelerated path to mastering AI tools and building revolutionary applications.
---------------------------
Resources and Community
Join our community of 12,000+ AI enthusiasts and learn to build powerful AI applications! Whether you're a beginner or an experienced developer, this tutorial will help you understand and implement AI agents in your projects.
- Website: www.buildfastwithai.com
- LinkedIn: linkedin.com/company/build-fast-with-ai/
- Instagram: instagram.com/buildfastwithai/
- Twitter: x.com/satvikps
- Telegram: t.me/BuildFastWithAI