Mastering NLP with Promptify
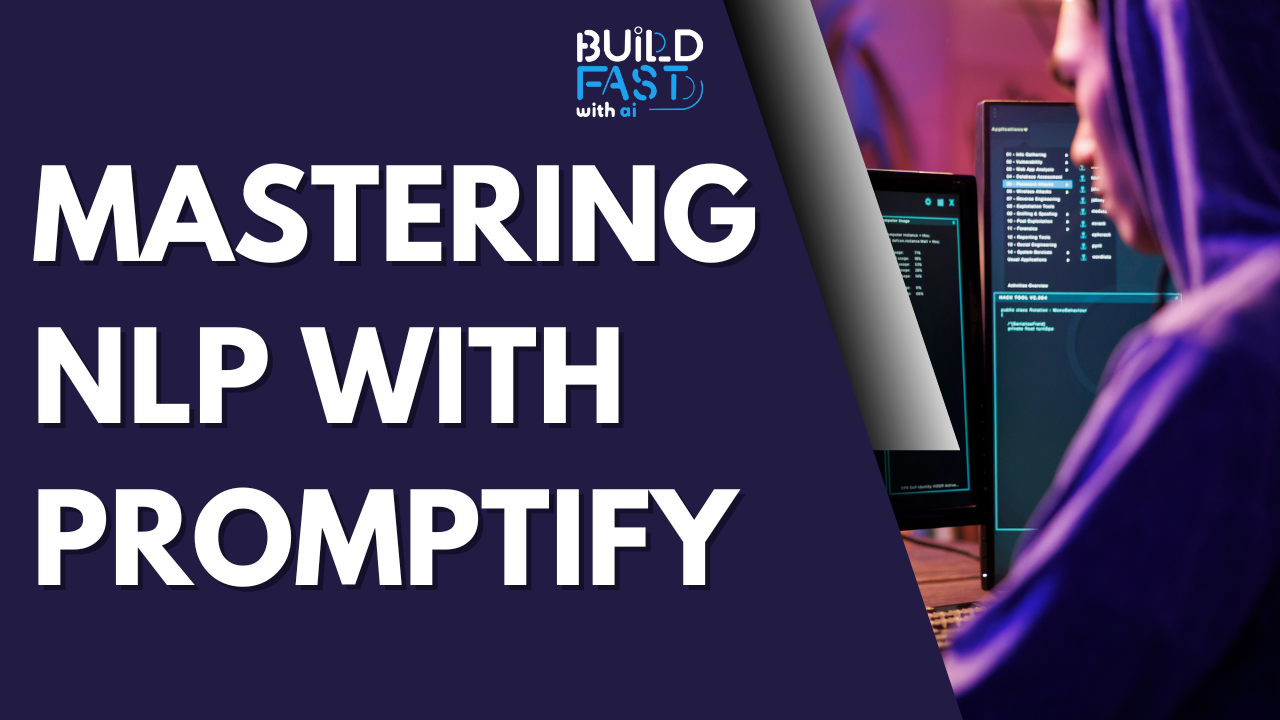
Will you be part of the change or just watch it happen?
Be bold—join Gen AI Launch Pad 2025 today.
Introduction
Prompt engineering is an essential skill for effectively utilizing large language models (LLMs) such as GPT-3.5 and PaLM. Promptify is a powerful tool that simplifies prompt generation for various Natural Language Processing (NLP) tasks, making it easier to extract structured outputs without the need for extensive training data.
In this guide, we’ll walk through how to use Promptify for key NLP tasks like Named Entity Recognition (NER), binary classification, sentiment analysis, and topic extraction. You’ll learn how to set up Promptify, understand its key features, and apply it in real-world scenarios with just a few lines of Python code.
Setting Up Promptify
Installation
To install Promptify, run the following command:
!pip install promptify
Setting Up the API Key
Since Promptify interacts with generative AI models, you’ll need an API key. In Google Colab, retrieve it using:
from google.colab import userdata api_key = userdata.get("OPENAI_API_KEY")
Alternatively, if you're using a local environment, store your key as an environment variable:
import os api_key = os.getenv("OPENAI_API_KEY")
Named Entity Recognition (NER) with Promptify
Named Entity Recognition (NER) extracts structured information from unstructured text. Here’s how Promptify makes NER effortless:
Code Implementation
from promptify import Prompter, OpenAI, Pipeline sentence = """The patient is a 93-year-old female with a medical history of chronic right hip pain, osteoporosis, hypertension, depression, and chronic atrial fibrillation admitted for evaluation and management of severe nausea and vomiting and urinary tract infection.""" model = OpenAI(api_key) prompter = Prompter('ner.jinja') pipe = Pipeline(prompter, model) result = pipe.fit(sentence, domain="medical", labels=None) print(result)
Expected Output
The output is a structured JSON-like list of extracted entities:
[ {"T": "Age", "E": "93-year-old"}, {"T": "Gender", "E": "female"}, {"T": "Medical Condition", "E": "chronic right hip pain"}, {"T": "Medical Condition", "E": "osteoporosis"}, {"T": "Medical Condition", "E": "hypertension"}, {"T": "Medical Condition", "E": "depression"}, {"T": "Medical Condition", "E": "chronic atrial fibrillation"}, {"T": "Reason for Admission", "E": "evaluation and management of severe nausea and vomiting and urinary tract infection"} ]
Use Cases
- Extracting structured data from medical reports.
- Automating entity recognition in legal or business documents.
- Improving search and indexing capabilities in text-heavy applications.
Binary Classification Using Promptify
Binary classification assigns a label (e.g., positive/negative) to input text. Here’s how Promptify enables this task:
Code Implementation
labels = ['positive', 'negative'] data = [ {'text': 'This is great', 'label': 'positive'}, {'text': 'This is awful', 'label': 'negative'} ] sent = "The patient is a 93-year-old female..." model_name = "gpt-3.5-turbo" model = OpenAI(api_key=api_key, model=model_name) template_string = """ You are a highly intelligent Binary Classification system. Classify the passage as either {{ label_0 }} or {{ label_1 }}. Output format: [{'C':Category}] Input: {{ text_input }} Output: """ prompter = Prompter(template=template_string, from_string=True) pipe = Pipeline(prompter, model) result = pipe.fit(sent, label_0=labels[0], label_1=labels[1], examples=data) print(result[0])
Expected Output
{"C": "negative"}
Use Cases
- Sentiment classification in customer reviews.
- Spam detection in email filtering.
- Fraud detection in financial transactions.
Sentiment Analysis with Promptify
Sentiment analysis determines whether text conveys a positive or negative sentiment.
Code Implementation
template = """Classify the sentiment of the following text as positive or negative. Text: {{text_input}} Sentiment:""" prompter = Prompter(template, from_string=True) pipe = Pipeline(prompter, model, structured_output=False) text = "This movie was amazing! I loved it." result = pipe.fit(text) if result: print(f"Sentiment: {result[0]}")
Expected Output
Sentiment: Positive
Use Cases
- Analyzing social media sentiment.
- Monitoring brand reputation.
- Enhancing chatbot responses.
Topic Extraction with Promptify
Topic extraction identifies the main subject of a text.
Code Implementation
template = """Extract the main topic from the following text: Text: {{text_input}} Topic:""" prompter = Prompter(template, from_string=True) pipe = Pipeline(prompter, model, structured_output=False) text = "The quick brown fox jumps over the lazy dog." result = pipe.fit(text) if result: print(f"Topic: {result[0]}")
Expected Output
Topic: English Pangram
Use Cases
- Summarizing news articles.
- Categorizing customer feedback.
- Enhancing content recommendations.
Conclusion
Promptify simplifies NLP tasks like NER, classification, sentiment analysis, and topic extraction with minimal code. By leveraging structured prompts, it ensures accurate and formatted outputs, making it ideal for real-world applications in healthcare, finance, marketing, and more.
To explore further, try experimenting with custom templates and additional Promptify features!
Resources
---------------------------
Stay Updated:- Follow Build Fast with AI pages for all the latest AI updates and resources.
Experts predict 2025 will be the defining year for Gen AI Implementation. Want to be ahead of the curve?
Join Build Fast with AI’s Gen AI Launch Pad 2025 - your accelerated path to mastering AI tools and building revolutionary applications.
---------------------------
Resources and Community
Join our community of 12,000+ AI enthusiasts and learn to build powerful AI applications! Whether you're a beginner or an experienced developer, this tutorial will help you understand and implement AI agents in your projects.
- Website: www.buildfastwithai.com
- LinkedIn: linkedin.com/company/build-fast-with-ai/
- Instagram: instagram.com/buildfastwithai/
- Twitter: x.com/satvikps
- Telegram: t.me/BuildFastWithAI