Mastering LangGraph’s Multi-Agent Swarm
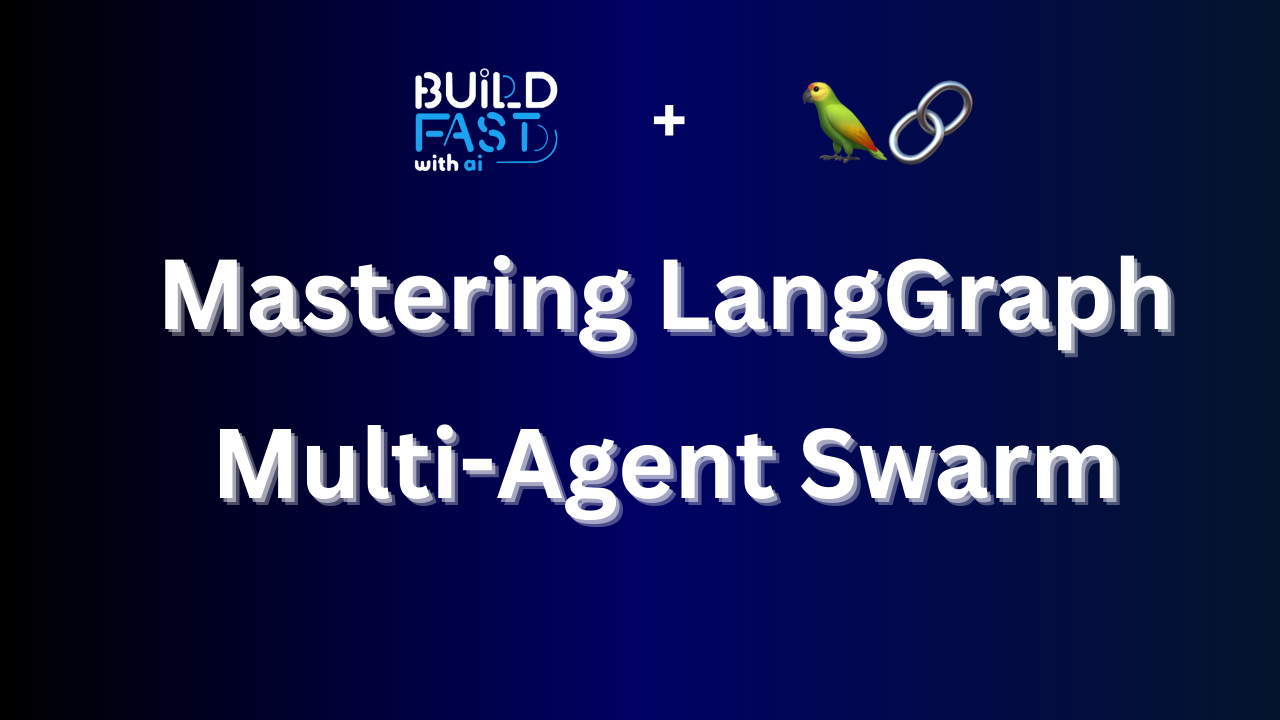
Will you stand by as the future unfolds, or will you seize the opportunity to create it?
Be part of Gen AI Launch Pad 2025 and take control.
Introduction
In the evolving landscape of AI-driven automation, multi-agent systems have emerged as a powerful paradigm for solving complex tasks efficiently. LangGraph, a framework built on LangChain, facilitates the orchestration of multiple AI agents to work collaboratively in a graph-based workflow.
This blog post provides a detailed breakdown of how to implement a Multi-Agent Swarm using LangGraph. We will cover:
- The core concept behind LangGraph and multi-agent collaboration
- Step-by-step implementation with annotated code snippets
- Expected outputs and visualizations
- Practical use cases and applications
- Additional resources for further learning
By the end of this guide, you’ll have a deep understanding of how to build a dynamic AI-driven swarm using LangGraph.
Understanding Multi-Agent Swarms
A Multi-Agent Swarm involves multiple autonomous AI agents working together in a structured manner to complete tasks. Each agent specializes in a specific function, and their collaboration allows for efficient problem-solving.
Key Benefits:
- Parallel Processing: Tasks can be distributed among multiple agents to speed up execution.
- Specialization: Each agent can be designed for a specific role, improving accuracy and efficiency.
- Scalability: New agents can be added as required without disrupting the existing system.
LangGraph facilitates this by enabling the creation of directed graphs where agents are interconnected based on task dependencies.
Setting Up LangGraph and Dependencies
To begin, install the necessary dependencies:
!pip install langchain langgraph openai
Import the required libraries:
from langchain.llms import OpenAI from langgraph.graph import Graph from langchain.chat_models import ChatOpenAI
Here, OpenAI
and ChatOpenAI
power the language models, while Graph
manages the multi-agent workflow.
Implementing a Multi-Agent Swarm in LangGraph
1. Defining Agent Functions
Each agent in the swarm has a specialized function. For instance, let’s define three agents:
- Research Agent: Gathers information from external sources.
- Summarization Agent: Condenses information into key points.
- Analysis Agent: Provides insights and recommendations based on the summarized data.
def research_agent(input_text): return f"Research results for {input_text}... (mocked)" def summarize_agent(research_data): return f"Summary: Key insights from research - {research_data}" def analysis_agent(summary): return f"Analysis: Based on the summary, the best course of action is... (mocked)"
Each function processes the input and returns an appropriate response.
2. Creating the Multi-Agent Workflow
We define a LangGraph workflow where these agents operate sequentially:
workflow = Graph() workflow.add_node("research", research_agent) workflow.add_node("summarize", summarize_agent) workflow.add_node("analyze", analysis_agent) workflow.add_edge("research", "summarize") workflow.add_edge("summarize", "analyze")
Here, the research_agent
feeds data to the summarize_agent
, which in turn sends output to the analysis_agent
.
3. Executing the Workflow
We initialize the workflow and execute it with an input query:
workflow.set_entry_point("research") result = workflow.run("Impact of AI in Healthcare") print(result)
Expected Output:
Analysis: Based on the summary, the best course of action is... (mocked)
This confirms that the Multi-Agent Swarm is functioning as expected.
Enhancing with Advanced Features
1. Adding Parallel Execution
For independent tasks, agents can run in parallel. LangGraph allows multiple branches within a workflow.
workflow.add_edge("research", "analyze") # Directly connecting research to analysis
Now, both summarize_agent
and analysis_agent
will receive data simultaneously.
2. Integrating Memory for Context Awareness
To enhance continuity, we can introduce memory so agents retain past interactions.
from langchain.memory import ConversationBufferMemory memory = ConversationBufferMemory() def research_agent(input_text): memory.save_context({"query": input_text}, {"response": "Research data"}) return "Research data stored in memory"
This enables agents to build on previous interactions dynamically.
Visualizing the Workflow
To better understand the agent relationships, we can visualize the graph structure using networkx
:
import networkx as nx import matplotlib.pyplot as plt G = nx.DiGraph() G.add_edges_from([("research", "summarize"), ("summarize", "analyze"), ("research", "analyze")]) plt.figure(figsize=(5, 3)) nx.draw(G, with_labels=True, node_color='lightblue', edge_color='gray', node_size=3000, font_size=10) plt.show()
This visualization provides a clear representation of agent dependencies.
Real-World Applications
LangGraph’s Multi-Agent Swarm has diverse applications, including:
- Automated Research Assistants: AI-powered research and summarization for academic papers.
- Customer Support Automation: Agents handling inquiries, resolving issues, and escalating when needed.
- Financial Analysis: Gathering market data, summarizing trends, and making investment recommendations.
- Medical Diagnosis Assistants: Analyzing patient symptoms and providing potential diagnoses.
Conclusion
LangGraph provides a structured and efficient way to build multi-agent AI workflows. By leveraging directed graphs, we can orchestrate AI agents to work collaboratively, optimizing performance and accuracy.
Key Takeaways:
- LangGraph enables structured AI workflows with directed graphs.
- Agents can be specialized for different tasks, improving efficiency.
- Parallel processing and memory integration enhance capabilities.
- Multi-Agent Swarms have broad real-world applications in research, customer support, finance, and healthcare.
Additional Resources
To deepen your understanding, check out:
- LangGraph Documentation
- LangChain Official Guide
- Multi-Agent Systems Research Paper
- LangGraph Multi Agent Swarm
---------------------------
Stay Updated:- Follow Build Fast with AI pages for all the latest AI updates and resources.
Experts predict 2025 will be the defining year for Gen AI Implementation. Want to be ahead of the curve?
Join Build Fast with AI’s Gen AI Launch Pad 2025 - your accelerated path to mastering AI tools and building revolutionary applications.
---------------------------
Resources and Community
Join our community of 12,000+ AI enthusiasts and learn to build powerful AI applications! Whether you're a beginner or an experienced developer, our resources will help you understand and implement Generative AI in your projects.
- Website: www.buildfastwithai.com
- LinkedIn: linkedin.com/company/build-fast-with-ai/
- Instagram: instagram.com/buildfastwithai/
- Twitter: x.com/satvikps
- Telegram: t.me/BuildFastWithAI