Langroid: Simplifying LLM-Powered Chatbots
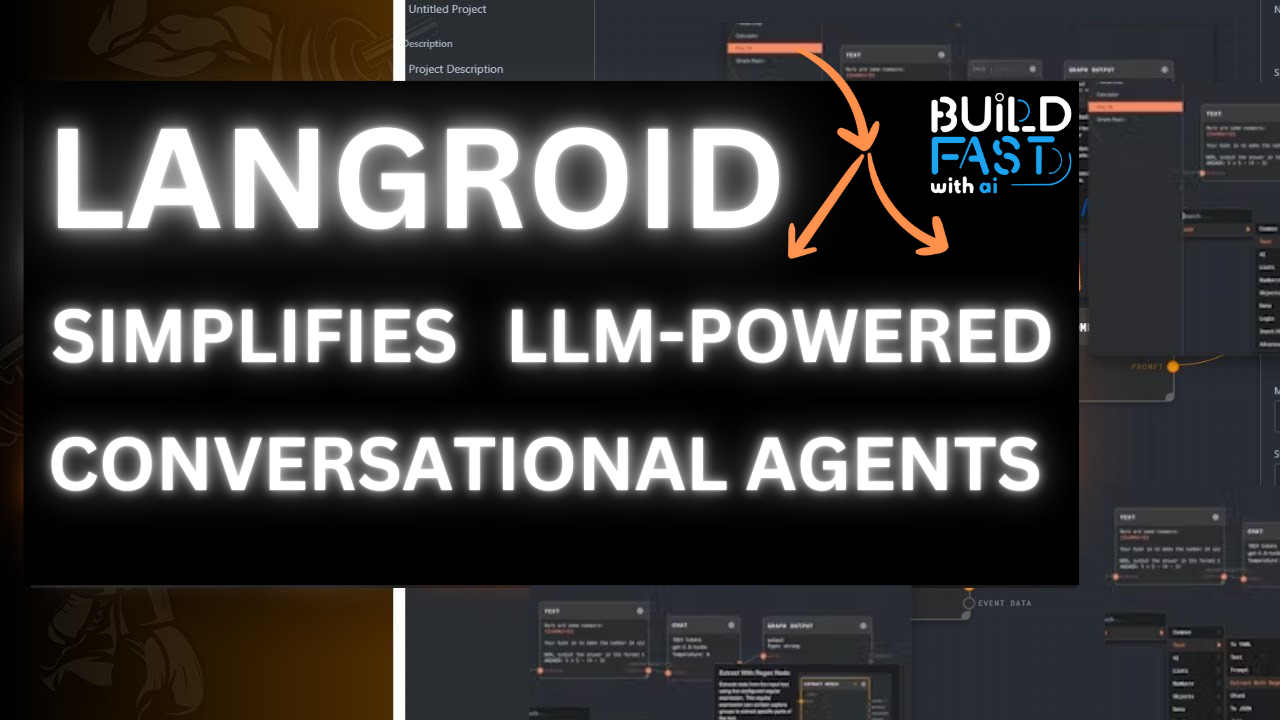
Are you hesitating while the next big breakthrough happens?
Don’t wait—be part of Gen AI Launch Pad 2025 and make history.
Introduction
Language models have transformed how we interact with AI, but building effective conversational agents still requires managing prompts, handling user input, and optimizing model responses. Langroid simplifies this process, making it easier to develop, test, and deploy LLM-powered agents. This blog post will walk through a Jupyter notebook demonstrating Langroid's capabilities, explaining each code snippet, and highlighting key concepts.
Setting Up Langroid
To get started, we need to install Langroid and import necessary libraries:
!pip install langroid import langroid as lg from langroid.agent.chat_agent import ChatAgent, ChatAgentConfig from langroid.utils.logging import setup_colored_logging setup_colored_logging()
This sets up Langroid and its logging system, which provides color-coded logs for better debugging and tracking. The setup_colored_logging() function ensures that different types of messages (e.g., warnings, errors, information logs) are easily distinguishable.
Creating a Simple Chat Agent
We define a configuration for our chat agent, specifying parameters such as verbosity and response behavior.
config = ChatAgentConfig(verbosity=2) agent = ChatAgent(config)
- ChatAgentConfig sets up parameters like verbosity level to control debugging logs.
- ChatAgent initializes an agent using this configuration.
- Verbosity levels range from 0 (silent) to higher values that increase the amount of logging.
Example Interaction
response = agent.chat("What is Langroid?") print(response)
This sends a user query to the chat agent and prints the model’s response. The output would be a detailed explanation of Langroid. The response depends on the underlying LLM being used.
Handling User Input and Responses
Langroid enables structured interactions, allowing us to define custom behavior.
def interactive_chat(): while True: user_input = input("You: ") if user_input.lower() == "exit": break response = agent.chat(user_input) print("Bot:", response) interactive_chat()
Explanation:
- The function runs an infinite loop where users enter messages.
- If the user types "exit," the loop breaks, terminating the conversation.
- Otherwise, the agent processes the input and returns a response.
- This allows for a real-time chatbot experience.
Advanced Features
Adding Memory to Conversations
By default, LLMs process each query independently. Langroid supports conversation memory:
agent.enable_memory(True)
This ensures that previous interactions are considered in subsequent responses, making conversations more context-aware.
Controlling Response Length
config.max_response_tokens = 100
This limits responses to 100 tokens, ensuring concise and efficient replies. Token limits help control cost and prevent excessively long responses that may be redundant.
Streaming Responses
for chunk in agent.chat_stream("Tell me about Langroid."): print(chunk, end="")
This streams responses chunk by chunk, enhancing real-time interactions. This is useful for applications like AI-driven assistants where immediate feedback is necessary.
Visualizing Chat Interactions
Langroid can generate conversational logs and insights. We can use Matplotlib for basic visualizations:
import matplotlib.pyplot as plt log_data = agent.get_log_data() plt.plot(log_data['tokens_used']) plt.xlabel("Query Number") plt.ylabel("Tokens Used") plt.title("Token Usage per Query") plt.show()
Explanation:
- get_log_data() retrieves usage data such as the number of tokens used in each query.
- Matplotlib is used to plot this data, showing trends in token consumption.
- Such analysis helps optimize performance and cost, ensuring efficient LLM usage.
Error Handling and Debugging in Langroid
When working with Langroid, debugging is crucial to handle potential issues like missing responses, high latency, or unexpected behavior. Common strategies include:
try: response = agent.chat("Explain Langroid in simple terms.") print(response) except Exception as e: print("Error occurred:", e)
This ensures that errors are gracefully caught and logged instead of crashing the program.
Applications of Langroid
Langroid is ideal for:
- Customer Support Bots: Automating responses to common queries.
- Education Assistants: Providing personalized tutoring.
- Research Assistants: Summarizing and retrieving information efficiently.
- AI-powered Productivity Tools: Automating workflows, email responses, and knowledge retrieval.
Conclusion
Langroid significantly simplifies LLM-powered agent development, providing a robust framework for building intelligent conversational AI. By using memory, streaming, and response control, developers can enhance chatbot interactions and optimize efficiency.
Langroid’s modularity makes it adaptable for various use cases, from simple Q&A bots to complex enterprise solutions. Developers can experiment with different configurations, integrate external APIs, and analyze chat performance for continual improvement.
Resources
- Langroid Documentation
- OpenAI API
- Matplotlib for Data Visualization
- Python Exception Handling
- Langroid Experiment Notebook
---------------------------
Stay Updated:- Follow Build Fast with AI pages for all the latest AI updates and resources.
Experts predict 2025 will be the defining year for Gen AI Implementation. Want to be ahead of the curve?
Join Build Fast with AI’s Gen AI Launch Pad 2025 - your accelerated path to mastering AI tools and building revolutionary applications.
---------------------------
Resources and Community
Join our community of 12,000+ AI enthusiasts and learn to build powerful AI applications! Whether you're a beginner or an experienced developer, this tutorial will help you understand and implement AI agents in your projects.
- Website: www.buildfastwithai.com
- LinkedIn: linkedin.com/company/build-fast-with-ai/
- Instagram: instagram.com/buildfastwithai/
- Twitter: x.com/satvikps
- Telegram: t.me/BuildFastWithAI