Mastering Hugging Face Transformers🚀
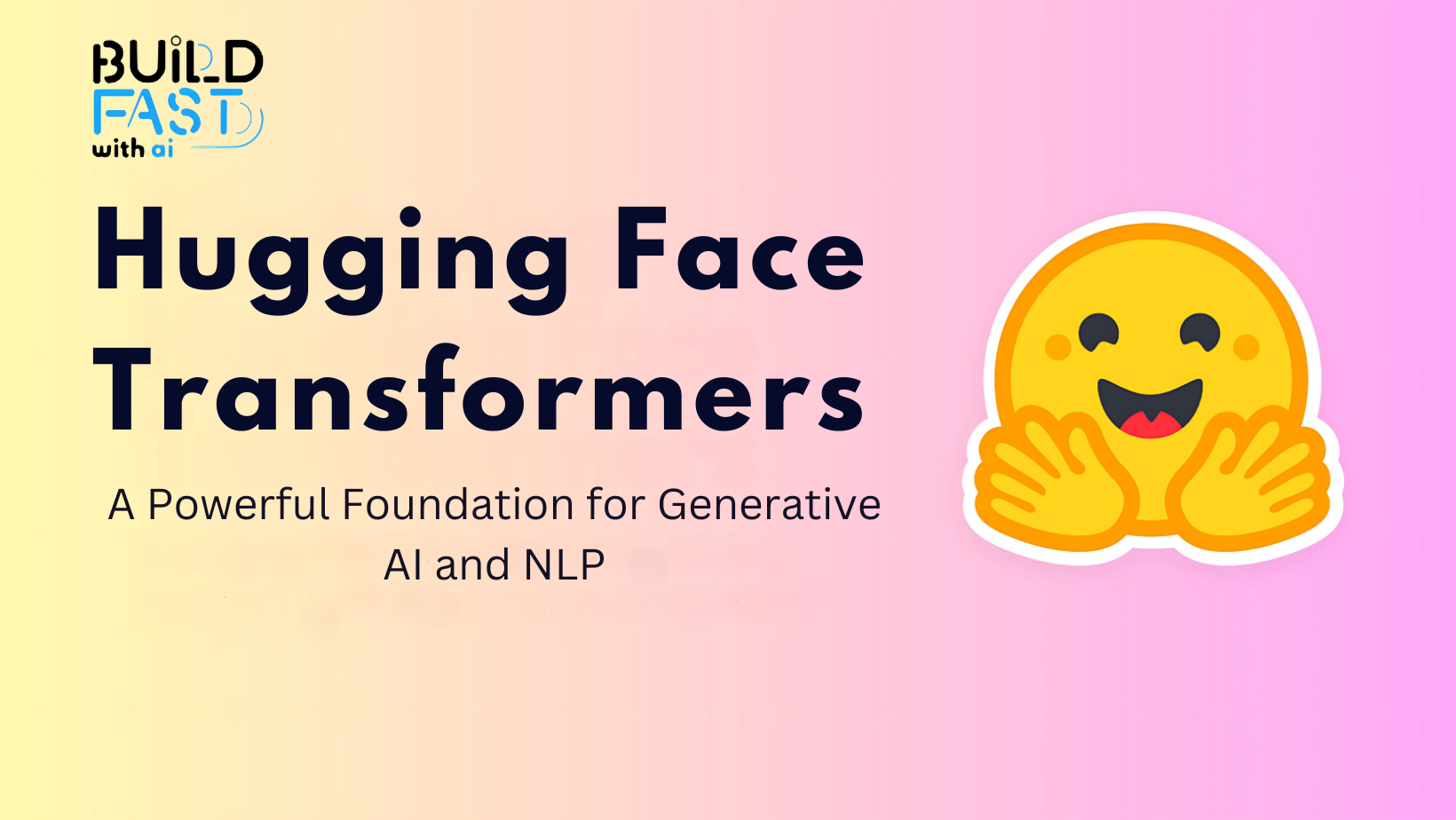
Will you stand by as the future unfolds, or will you seize the opportunity to create it?
Be part of Gen AI Launch Pad 2025 and take control.
Introduction
Hugging Face Transformers is an open-source library that has revolutionized Natural Language Processing (NLP) and Generative AI. By providing easy access to state-of-the-art transformer models, it enables developers and researchers to build applications ranging from text classification to machine translation and fine-tuning custom models.
This blog post is an in-depth guide to understanding Hugging Face Transformers, showcasing its capabilities with practical code examples, explanations, expected outputs, and real-world applications.
Key Features of Hugging Face Transformers
1. Pre-Trained Models
Hugging Face provides a vast collection of pre-trained models, including:
- GPT, BART, and T5 for text generation
- BERT and RoBERTa for NLP tasks like classification, NER, and QA
- Vision Transformer (ViT) for image classification
2. Core NLP and GenAI Tasks
Hugging Face simplifies complex tasks, including:
- Text generation (GPT-2, GPT-3, T5)
- Text classification (DistilBERT, BERT, RoBERTa)
- Named Entity Recognition (NER)
- Machine Translation
- Question Answering (QA)
- Fine-tuning and Custom Models
3. Tools for Managing Large Language Models (LLMs)
Hugging Face provides robust tools to train, optimize, and deploy large-scale transformer models efficiently.
Setting Up Hugging Face Transformers
To get started, install the required packages:
!pip install transformers datasets
This command installs both the transformers
library and the datasets
library for working with NLP datasets.
Text Classification with Hugging Face
Text classification helps determine the sentiment or category of a given text. Here’s how you can use a pre-trained model to classify text:
Code Snippet:
from transformers import pipeline classifier = pipeline("text-classification", model="distilbert-base-uncased-finetuned-sst-2-english") result = classifier("This movie was absolutely fantastic!") print(result)
Expected Output:
[{'label': 'POSITIVE', 'score': 0.999874472618103}]
Explanation:
pipeline
: Simplifies access to various models.text-classification
: Specifies that we want to classify text.- Pre-trained Model:
distilbert-base-uncased-finetuned-sst-2-english
is fine-tuned for sentiment analysis. - Application: Can be used for product reviews, social media analysis, and more.
Text Generation using GPT-2
GPT-2 can generate human-like text. Here’s how:
Code Snippet:
from transformers import AutoTokenizer, AutoModelForCausalLM tokenizer = AutoTokenizer.from_pretrained("gpt2") model = AutoModelForCausalLM.from_pretrained("gpt2") input_text = "In a shocking turn of events, scientists discovered" inputs = tokenizer(input_text, return_tensors="pt") outputs = model.generate(**inputs, max_length=100, temperature=0.7) print(tokenizer.decode(outputs[0]))
Expected Output:
GPT-2 generates text based on the given prompt. Example output:
In a shocking turn of events, scientists discovered that...
Explanation:
- GPT-2 Model: Generates coherent and context-aware text.
temperature=0.7
: Controls creativity of output (lower values make responses more predictable).- Application: Used in content generation, chatbots, and creative writing.
Named Entity Recognition (NER)
NER extracts entities like names, organizations, and locations from text.
Code Snippet:
ner = pipeline("ner", model="dslim/bert-base-NER") result = ner("Hugging Face is a company based in New York City.") print(result)
Expected Output:
[{'entity': 'B-ORG', 'word': 'Hugging Face'}, {'entity': 'B-LOC', 'word': 'New York City'}]
Explanation:
- NER Model: Extracts entities from the text.
- Application: Useful in information retrieval, document processing, and AI-driven assistants.
Machine Translation
Translate English to German using a pre-trained transformer model.
Code Snippet:
translator = pipeline("translation", model="Helsinki-NLP/opus-mt-en-de") result = translator("Hello world!", max_length=40) print(result[0]['translation_text'])
Expected Output:
Hallo Welt!
Application:
- Used in multilingual applications and real-time translation services.
Fine-Tuning a Model
Fine-tuning allows models to be customized for specific use cases, such as classifying movie reviews.
Code Snippet:
from transformers import AutoModelForSequenceClassification, AutoTokenizer, Trainer, TrainingArguments from datasets import load_dataset model = AutoModelForSequenceClassification.from_pretrained("bert-base-uncased", num_labels=2) tokenizer = AutoTokenizer.from_pretrained("bert-base-uncased") dataset = load_dataset("imdb") dataset = dataset.map(lambda x: tokenizer(x["text"], padding=True, truncation=True), batched=True) training_args = TrainingArguments(output_dir="./results", num_train_epochs=1, per_device_train_batch_size=16) trainer = Trainer(model=model, args=training_args, train_dataset=dataset["train"]) trainer.train()
Explanation:
- Fine-tunes BERT for binary sentiment classification.
- Uses the IMDb movie review dataset.
- Essential for domain-specific NLP applications.
Conclusion
Hugging Face Transformers offers a robust ecosystem for building cutting-edge NLP and GenAI applications. From pre-trained models to fine-tuning and deployment, this library empowers developers and researchers alike.
💡 Next Steps
- Explore Hugging Face Hub for new models.
- Try fine-tuning on custom datasets.
- Implement transformers in real-world applications.
Resources
---------------------------
Stay Updated:- Follow Build Fast with AI pages for all the latest AI updates and resources.
Experts predict 2025 will be the defining year for Gen AI Implementation. Want to be ahead of the curve?
Join Build Fast with AI’s Gen AI Launch Pad 2025 - your accelerated path to mastering AI tools and building revolutionary applications.
---------------------------
Resources and Community
Join our community of 12,000+ AI enthusiasts and learn to build powerful AI applications! Whether you're a beginner or an experienced developer, this tutorial will help you understand and implement AI agents in your projects.
- Website: www.buildfastwithai.com
- LinkedIn: linkedin.com/company/build-fast-with-ai/
- Instagram: instagram.com/buildfastwithai/
- Twitter: x.com/satvikps
- Telegram: t.me/BuildFastWithAI