FireCrawl: Advanced Web Scraping and Data Extraction for AI Applications
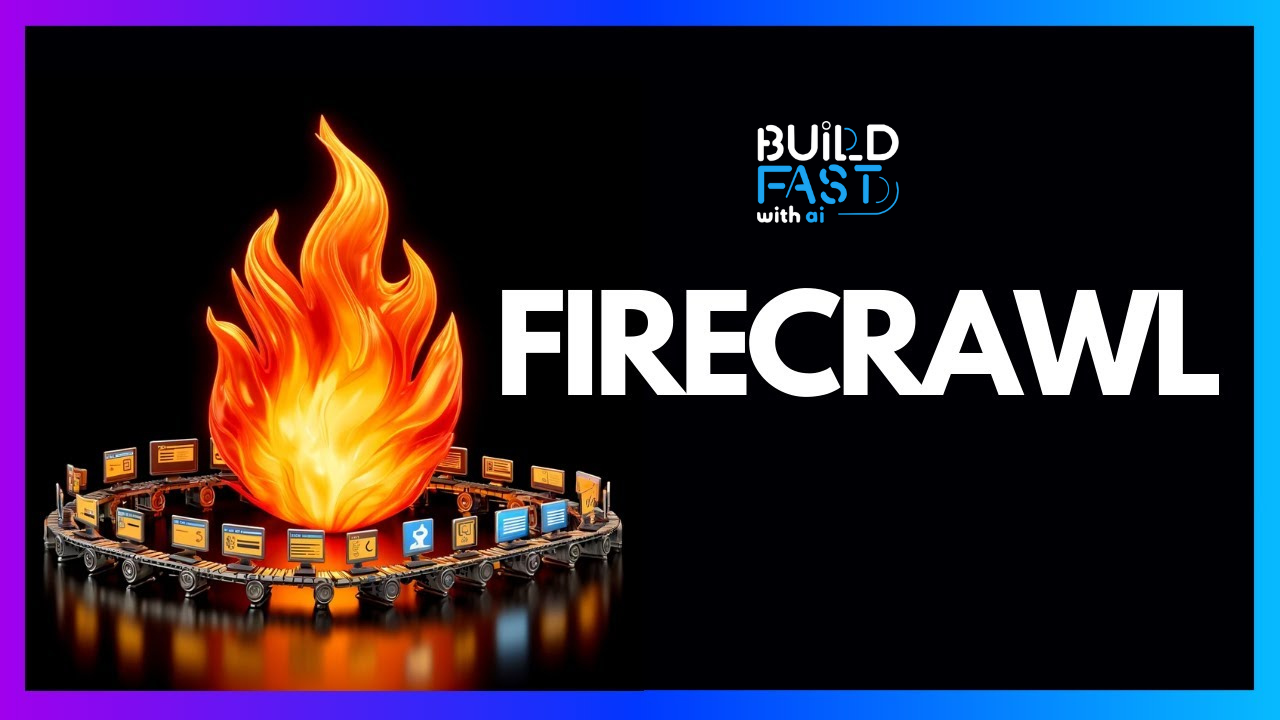
What’s the limit of AI’s potential?
At Gen AI Launch Pad 2024, redefine what’s possible. Step up and be the pioneer shaping the limitless future of AI.
Introduction
The explosion of artificial intelligence has created an insatiable demand for clean, well-structured, and actionable data. Web scraping, when done efficiently, can power AI models with real-time data, automate mundane tasks, and open new horizons for data-driven applications.
FireCrawl is a cutting-edge Python library designed specifically to tackle the challenges of modern web scraping. From handling dynamic pages to extracting structured formats like Markdown or HTML, FireCrawl empowers developers to focus on building innovative AI applications rather than struggling with data collection.
In this blog, you’ll learn:
- How to set up and install FireCrawl.
- Examples of basic and advanced web scraping tasks.
- Detailed code walkthroughs with expected outputs.
- Real-world use cases where FireCrawl shines.
- Resources for further learning.
Setup and Installation
To begin, install FireCrawl using pip
. Here’s how to get started:
Code Snippet
pip install firecrawl-py
Explanation
This command installs the firecrawl-py
library. It’s lightweight and designed to integrate seamlessly with AI and data workflows.
Configuring the API Key
FireCrawl uses an API key to authenticate your requests. Follow these steps to configure it securely in Google Colab:
Code Snippet
from google.colab import userdata import os # Fetch API key securely os.environ['FIRECRAWL_API_KEY'] = userdata.get('FIRECRAWL_API_KEY') # Assign the key to a variable firecrawl_api_key = os.getenv("FIRECRAWL_API_KEY")
Explanation
- The
userdata.get
method retrieves the API key directly from Colab's secure storage. - The API key is then stored in an environment variable to ensure it’s not exposed in your code.
Expected Output
This block doesn't generate visible output but ensures that your API key is ready for subsequent operations.
Visual Aid Suggestion
Include a screenshot of the Colab setup showing the API key retrieval process.
Scraping a Website
Here’s how you can scrape a website with FireCrawl and retrieve data in multiple formats:
Code Snippet
from firecrawl.firecrawl import FirecrawlApp # Initialize FireCrawl with the API key app = FirecrawlApp(api_key=firecrawl_api_key) # Scrape a website scrape_status = app.scrape_url( 'https://www.buildfastwithai.com/', params={'formats': ['markdown', 'html']} ) # Print the scraping status print(scrape_status)
Explanation
- Initialization: The
FirecrawlApp
class initializes the library with your API key. - Scrape Website: The
scrape_url
method fetches data from the given URL.
- The
params
dictionary specifies the desired output formats (markdown
andhtml
).
- Status Check: The output of
scrape_url
provides feedback on whether the scraping was successful.
Expected Output
{ "status": "success", "data": { "markdown": "# Welcome to BuildFastWithAI\n...", "html": "<html><body><h1>Welcome...</h1></body></html>" } }
This JSON-like response includes:
- A status indicating success or failure.
- The extracted data in the requested formats.
Real-World Use Case
- Use this data to power AI models that rely on up-to-date information from a particular domain.
- Automate the process of extracting structured content for blogs, research, or analytics.
Advanced Features of FireCrawl
- Handling Dynamic Content
- FireCrawl can interact with JavaScript-heavy websites by leveraging browser automation.
- Code Snippet
scrape_status = app.scrape_url( 'https://example.com/dynamic-page', params={'formats': ['json']}, render=True # Enables JavaScript rendering ) print(scrape_status)
- Explanation
- The
render=True
parameter activates a headless browser to render JavaScript content before scraping.
- Expected Output
{ "status": "success", "data": { "json": {"key1": "value1", "key2": "value2"} } }
- Real-World Use Case
- Extract product listings, reviews, or user-generated content from e-commerce platforms.
- Crawling Multiple Pages
- FireCrawl supports crawling through multiple pages, gathering data from all linked pages.
- Code Snippet
crawl_status = app.crawl_website( 'https://example.com', depth=2, params={'formats': ['html']} ) print(crawl_status)
- Explanation
- The
crawl_website
method explores the given URL up to the specified depth, scraping data from all reachable pages.
- Expected Output
{ "status": "success", "pages_scraped": 25, "data": { "html": ["<html>...</html>", "<html>...</html>", ...] } }
Visual Aids
- Flowcharts to explain the crawling process.
- Bar charts showing scraped data volume across pages.
Data Transformation and Storage
Once data is scraped, FireCrawl provides options to clean and store it for downstream AI applications:
Code Snippet
cleaned_data = app.clean_data(scrape_status['data']['html']) # Save cleaned data to a file with open('cleaned_data.html', 'w') as file: file.write(cleaned_data)
Explanation
- The
clean_data
method removes unnecessary elements like ads or tracking scripts. - Saves the cleaned data to a local file for further processing.
Expected Output
A cleaned HTML file ready for integration with machine learning workflows.
Conclusion
FireCrawl bridges the gap between raw web content and actionable AI data. Its powerful scraping, crawling, and cleaning capabilities make it indispensable for developers aiming to automate data collection for AI applications.
Key Takeaways:
- FireCrawl simplifies complex scraping tasks, including dynamic content rendering and multi-page crawling.
- It outputs data in flexible formats like HTML, JSON, or Markdown, tailored to AI workflows.
- Integration with tools like Google Colab ensures secure and scalable usage.
Resources
---------------------------------
Stay Updated:- Follow Build Fast with AI pages for all the latest AI updates and resources.
Experts predict 2025 will be the defining year for Gen AI implementation.Want to be ahead of the curve?
Join Build Fast with AI’s Gen AI Launch Pad 2025 - your accelerated path to mastering AI tools and building revolutionary applications.