FastAPI for AI Integration: Build and Deploy AI-Powered APIs
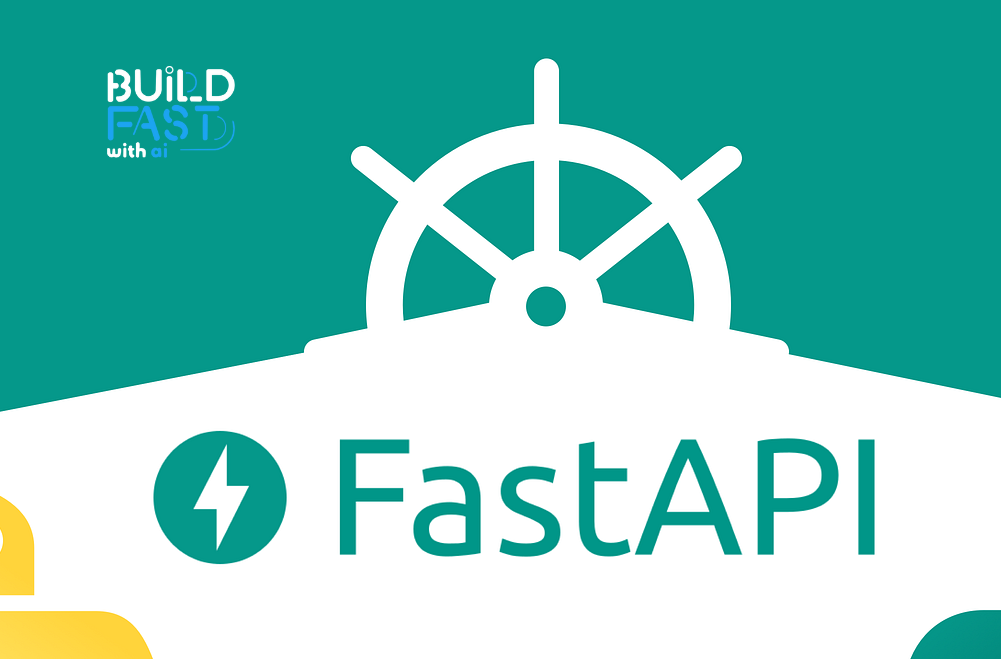
Are you waiting for change, or will you create it?
The future is calling—answer it with Gen AI Launch Pad 2025.
Introduction
FastAPI is a modern, high-performance web framework for building APIs with Python, optimized for asynchronous operations. It is widely used in AI applications, particularly for serving Generative AI models. This blog will guide you through setting up FastAPI for AI integration, covering installation, API authentication, request handling, and serving AI models with Google Generative AI.
By the end of this guide, you will:
- Understand the basics of FastAPI and its advantages.
- Learn how to set up an API server using FastAPI.
- Integrate Google Generative AI to create AI-powered applications.
- Deploy and test the FastAPI application.
1. Installing Dependencies
FastAPI relies on several libraries for web server functionality and AI integration. To begin, install the necessary packages using:
!pip install fastapi uvicorn python-multipart google-generativeai
Explanation of Installed Packages:
- FastAPI: The core framework for building APIs. It provides automatic validation, async support, and built-in documentation.
- Uvicorn: A high-performance ASGI server optimized for FastAPI applications.
- python-multipart: A package for handling form data in HTTP requests.
- google-generativeai: A Python client for interacting with Google’s AI models.
Why FastAPI?
- Performance: Based on Starlette and Pydantic, FastAPI offers better speed than traditional frameworks like Flask.
- Automatic Documentation: OpenAPI and Swagger UI are built-in.
- Asynchronous Support: Handles high loads efficiently with async/await.
2. Setting Up API Keys Securely
To interact with AI models, you need API keys. The safest way to store and access these keys is by using environment variables. If working in Google Colab, you can use:
from google.colab import userdata import os OPENAI_API_KEY = userdata.get('OPENAI_API_KEY') os.environ['OPENAI_API_KEY'] = OPENAI_API_KEY GOOGLE_API_KEY = userdata.get('GOOGLE_API_KEY') os.environ['GOOGLE_API_KEY'] = GOOGLE_API_KEY
Best Practices for API Key Security:
- Never hardcode keys in scripts.
- Use
.env
files for local development. - Use cloud services like AWS Secrets Manager or Google Secret Manager.
3. Creating a Basic FastAPI App
Let’s build a simple FastAPI application:
from fastapi import FastAPI app = FastAPI() @app.get("/") def read_root(): return {"message": "Welcome to FastAPI for AI!"}
To start the server, run:
uvicorn main:app --reload
Understanding the Code:
FastAPI()
initializes the app.@app.get("/")
defines an endpoint at/
.- The function returns a JSON response.
Expected Output:
Visit http://127.0.0.1:8000/
in your browser, and you should see:
{"message": "Welcome to FastAPI for AI!"}
4. Integrating Google Generative AI
To interact with Google AI, configure the API client:
import google.generativeai as genai genai.configure(api_key=os.getenv('GOOGLE_API_KEY')) def generate_text(prompt): response = genai.generate(prompt) return response
When to Use This:
- Chatbots that generate responses based on user input.
- Content generation for blogs, articles, or code snippets.
- Summarization of long texts into concise formats.
5. Creating AI-Powered API Endpoints
Now, let’s create an API endpoint that generates AI responses:
from pydantic import BaseModel class PromptRequest(BaseModel): prompt: str @app.post("/generate") def generate(request: PromptRequest): response = generate_text(request.prompt) return {"response": response}
Explanation:
BaseModel
: Ensures the request contains a validprompt
.@app.post("/generate")
: Defines a POST request.request: PromptRequest
: Parses incoming JSON data.generate_text(request.prompt)
: Calls the AI model.
Testing the API:
Run the following curl
command:
curl -X POST "http://127.0.0.1:8000/generate" -H "Content-Type: application/json" -d '{"prompt":"Tell me a joke"}'
Expected Output:
{"response": "Why did the chicken cross the road? To get to the other side!"}
6. Running and Testing the API
Start the FastAPI application using:
uvicorn main:app --host 0.0.0.0 --port 8000
Visit http://127.0.0.1:8000/docs
to access the Swagger UI, where you can test API endpoints.
Additional Testing Methods:
- Postman: A GUI tool to send API requests and inspect responses.
- Python Requests Module: Send requests programmatically.
import requests response = requests.post("http://127.0.0.1:8000/generate", json={"prompt": "Tell me a fun fact"}) print(response.json())
Conclusion
FastAPI is an excellent choice for AI applications due to its speed, ease of use, and automatic documentation. In this guide, we covered:
- Installing and setting up FastAPI.
- Securing API keys.
- Creating AI-powered endpoints with Google Generative AI.
- Running and testing the API.
Next Steps:
- Deploy your FastAPI app using Docker or Google Cloud Run.
- Integrate authentication using OAuth2 or JWT.
- Optimize performance with Redis caching.
Resources
---------------------------
Stay Updated:- Follow Build Fast with AI pages for all the latest AI updates and resources.
Experts predict 2025 will be the defining year for Gen AI Implementation. Want to be ahead of the curve?
Join Build Fast with AI’s Gen AI Launch Pad 2025 - your accelerated path to mastering AI tools and building revolutionary applications.
---------------------------
Resources and Community
Join our community of 12,000+ AI enthusiasts and learn to build powerful AI applications! Whether you're a beginner or an experienced developer, this tutorial will help you understand and implement AI agents in your projects.
- Website: www.buildfastwithai.com
- LinkedIn: linkedin.com/company/build-fast-with-ai/
- Instagram: instagram.com/buildfastwithai/
- Twitter: x.com/satvikps
- Telegram: t.me/BuildFastWithAI