Ell: The Language Model Programming Library
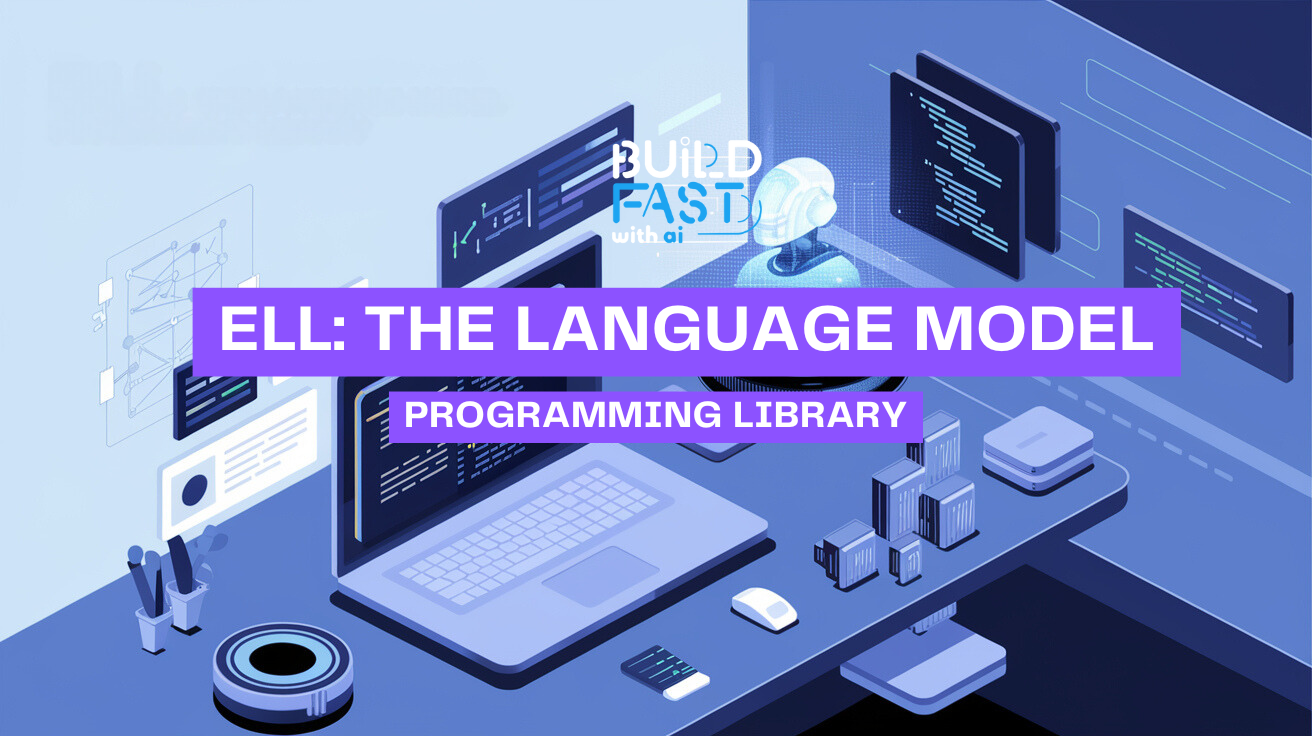
What’s the one problem AI hasn’t solved yet—could you be the one to solve it?"
Join Gen AI Launch Pad 2024 and lead the charge.
Introduction
Ell treats prompts as functions, making language model programming intuitive and developer-friendly. This abstraction allows developers to write clean, reusable, and efficient code for generating text, automating workflows, and creating AI-driven tools. This blog walks you through:
- Setting up Ell.
- Exploring basic and advanced usage.
- Utilizing Ell’s built-in tools.
- Generating structured outputs.
By the end of this blog, you’ll be equipped to leverage Ell for various real-world applications.
Setup and Installation
Install Required Libraries
To get started with Ell, install the necessary libraries using pip:
!pip install -U "ell-ai[all]" openai
This command installs Ell and its dependencies, ensuring you’re ready to harness the library’s capabilities.
Configure API Keys
Ell requires API keys to interact with models like OpenAI’s GPT. Configure your environment as shown below:
import os from google.colab import userdata os.environ['OPENAI_API_KEY'] = userdata.get('OPENAI_API_KEY')
Replace userdata.get('OPENAI_API_KEY')
with your actual OpenAI API key if not using a secure credential manager.
Real-World Application: Setting up API keys securely ensures compliance with best practices, especially for enterprise-level projects.
Basic Usage
Greeting a User
Ell simplifies interaction with language models using decorators. Let’s start with a simple example:
import ell @ell.simple(model="gpt-4o") def hello(name: str): """You are a helpful assistant.""" # System prompt return f"Say hello to {name}!" # User prompt greeting = hello("Sam Altman") print(greeting)
Explanation:
- The
@ell.simple
decorator defines a prompt as a function. - The function’s docstring acts as the system prompt, while the return statement dynamically generates the user prompt.
Expected Output:
Hello, Sam Altman! How can I assist you today?
Real-World Application: Use this pattern to create personalized user experiences in chatbots or virtual assistants.
Generating a Poem
Ell makes creative tasks straightforward. Below is an example of generating a poem:
import ell @ell.simple(model="gpt-4o") def write_poem(name: str): """You are a Poet.""" # System prompt return f"Write a poem for developer named: {name}!" # User prompt print(write_poem("Sam Altman"))
Expected Output:
Oh Sam, a coder so bright, With visions reaching endless height. Lines of code that ignite, Innovation in the dark of night. Sam, you are a guiding light.
Real-World Application: Generate creative content for blogs, marketing, or educational tools.
Advanced Usage
Prompting as Language Model Programming
Ell supports advanced prompting techniques like incorporating randomness or dynamic input generation.
import ell import random def get_random_adjective(): adjectives = ["enthusiastic", "cheerful", "warm", "friendly"] return random.choice(adjectives) @ell.simple(model="gpt-4o") def hello(name: str): """You are a helpful assistant.""" adjective = get_random_adjective() return f"Say hello to {name}, the {adjective} individual!" print(hello("Alex"))
Explanation:
- Dynamic inputs (e.g., adjectives) enhance prompt variability, creating unique outputs.
- Ell’s integration with Python functions offers flexibility for complex workflows.
Expected Output:
Hello, Alex, the cheerful individual!
Real-World Application: Create engaging, context-aware chat interfaces or automate personalized messaging.
Generating Structured Outputs
For applications requiring structured data, Ell integrates seamlessly with Pydantic:
import ell from pydantic import BaseModel, Field class MovieReview(BaseModel): title: str = Field(description="The title of the movie") rating: int = Field(description="The rating of the movie out of 10") review: str = Field(description="A brief review of the movie") @ell.simple(model="gpt-4o") def generate_review(movie_name: str): """You are a movie critic.""" return f"Write a review for the movie {movie_name}." review = generate_review("Inception") print(review)
Expected Output:
{ "title": "Inception", "rating": 9, "review": "A mind-bending thriller that redefines cinematic storytelling." }
Real-World Application: Automate review generation for e-commerce platforms or content moderation systems.
Utilizing Built-In Tools
Ell provides a framework for creating tools. Here’s an example using a custom weather retrieval tool:
@ell.tool() def get_weather(location: str = Field(description="The full name of a city and country, e.g., San Francisco, CA, USA")): """Get the current weather for a given location.""" # Simulated response return f"The weather in {location} is sunny, 25°C." print(get_weather("New York, NY, USA"))
Expected Output:
The weather in New York, NY, USA is sunny, 25°C.
Real-World Application: Integrate Ell tools into APIs for weather, stock prices, or custom data retrieval.
Conclusion
Ell simplifies the complex task of interacting with language models by turning prompts into reusable functions. From basic greetings to structured data generation and advanced prompting, it empowers developers to build smarter, more intuitive applications.
Key Takeaways:
- Treating prompts as functions streamlines development.
- Ell supports creativity, personalization, and structured outputs.
- Advanced features like tool creation make it versatile for real-world applications.
Resources
---------------------------------
Stay Updated:- Follow Build Fast with AI pages for all the latest AI updates and resources.
Experts predict 2025 will be the defining year for Gen AI implementation.Want to be ahead of the curve?
Join Build Fast with AI’s Gen AI Launch Pad 2025 - your accelerated path to mastering AI tools and building revolutionary applications.