ChromaDB : Efficient Vector Database for Embeddings
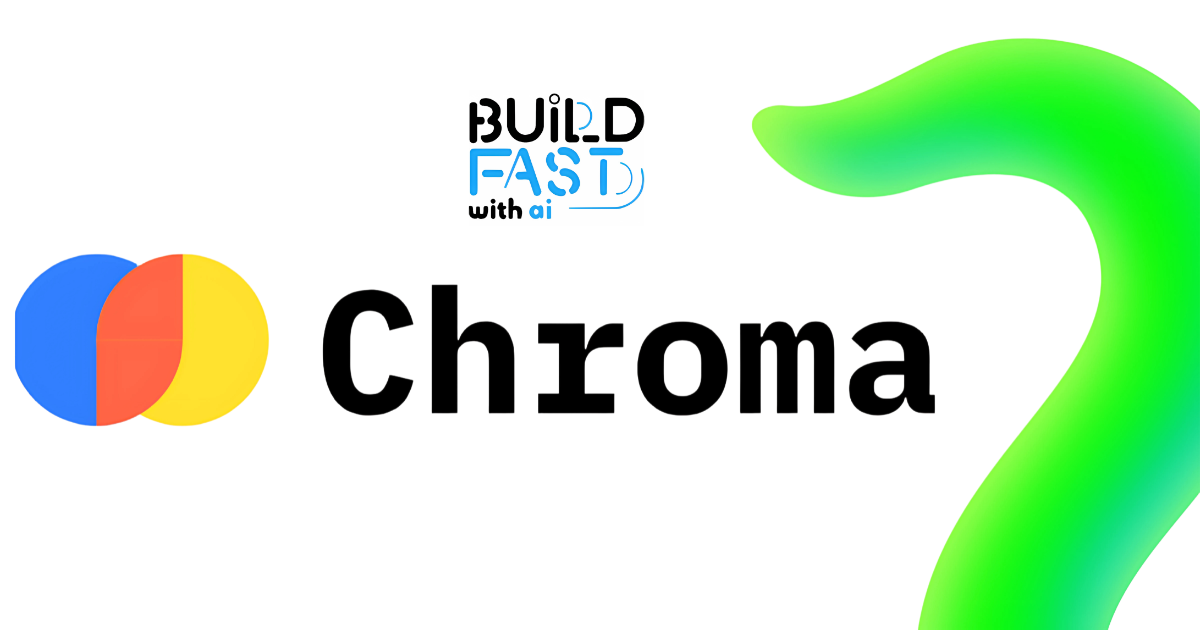
The best time to start with AI was yesterday. The second best time? Right after reading this post.
Join Build Fast with AI’s Gen AI Launch Pad 2025—a 6-week transformation program designed to accelerate your AI mastery and empower you to build revolutionary applications.
------------------------
What is ChromaDB?
Sure! Let's expand the blog post further, diving deeper into each section and explaining the process with more details, examples, and context around ChromaDB and its use cases. Here’s an extended version of the blog:
Mastering ChromaDB: A Comprehensive Guide to Efficient Vector Database for Embeddings
In the rapidly evolving field of machine learning and AI, embeddings play a pivotal role in representing complex data in a simplified and useful manner. Whether you're working with text, images, or any other form of data, embeddings help convert these entities into numerical representations that can be easily processed by machine learning models.
To manage, query, and store these embeddings efficiently, a vector database like ChromaDB is often used. ChromaDB provides the infrastructure necessary to handle large amounts of vector data, enabling rapid similarity searches, content-based retrieval, and machine learning applications. This guide will walk you through everything you need to know about ChromaDB, from installation to advanced use cases.
What is ChromaDB?
ChromaDB is an advanced vector database optimized for storing and querying embeddings. Embeddings are high-dimensional vectors that capture the essence of objects in a form that a machine can understand. For example, an image embedding is a vector of numbers that represents the content of the image in such a way that similar images will have similar vectors.
ChromaDB leverages these embeddings and stores them in a way that makes it fast and efficient to retrieve them based on similarity. It is especially useful in the following contexts:
- Visual Search: For applications that require finding similar images, such as in e-commerce platforms, social media, or content management systems.
- Recommendation Systems: ChromaDB can be used to suggest products, movies, books, or any other kind of item based on a user's preferences or past behavior.
- Machine Learning Pipelines: Storing embeddings generated from text, images, or other data types for use in tasks like classification, clustering, and semantic analysis.
How ChromaDB Works
At its core, ChromaDB stores embeddings in a vector format. These vectors can be generated from different kinds of data sources, such as images, text, audio, etc. ChromaDB allows you to insert, retrieve, and query these vectors efficiently using advanced indexing algorithms designed to minimize the time required for searching through large datasets.
Applications of ChromaDB include:
- Visual Search: Finding similar images or other multimedia content.
- Recommendation Systems: Suggesting related content or products.
- Machine Learning Pipelines: Storing embeddings for tasks such as clustering, classification, or semantic analysis.
Step 1: Setup and Installation
Before you can use ChromaDB, you need to set up the necessary libraries and tools. The notebook we’re referencing uses the following Python libraries:
- datasets – This is a popular library for accessing a variety of datasets, including those for image, text, and audio processing.
- chromadb – This is the core library for working with ChromaDB, which provides the functionality for managing and querying vector databases.
- open-clip-torch – A library for working with OpenCLIP models, which are used to generate embeddings from text and images.
You can install these libraries using the following command:
!pip install datasets chromadb open-clip-torch
This command installs everything you need to begin working with ChromaDB, including the pre-trained models required for generating embeddings. Once these libraries are installed, you can proceed to load and process your datasets.
Step 2: Load and Visualize Dataset Images 🖼️
Once the required libraries are installed, the next step is to load and visualize the dataset. In the notebook, we are dealing with image data, and the first operation typically involves loading these images for processing.
This code block loads the images, which will then be converted into embeddings and stored in the ChromaDB. The embeddings serve as the compact numerical representations of the images, allowing you to later perform similarity searches or other queries based on these embeddings.
# Example of loading dataset and visualizing from datasets import load_dataset # Load the dataset dataset = load_dataset('your_dataset_name') # Visualize the first image from the dataset image = dataset['train'][0]['image'] image.show()
In this example, we load a dataset and display the first image in the dataset. Visualization is an important step when working with image data because it lets you confirm that the images have been loaded correctly before further processing.
Step 3: Generating Embeddings for Images
After the images have been loaded, the next step is to convert them into embeddings using a pre-trained model. In this case, we use OpenCLIP, a version of CLIP (Contrastive Language–Image Pretraining) that is optimized for generating embeddings from images.
Here’s how you can generate embeddings for images using OpenCLIP:
from open_clip_torch import load_model # Load pre-trained OpenCLIP model model = load_model('ViT-B-32') # Generate embeddings for an image embedding = model.encode_image(image)
What are embeddings?
- Embeddings are dense vectors of floating-point numbers that represent the content of an image, text, or any other data.
- The model we’re using here, OpenCLIP, has been trained on massive datasets and can generate embeddings that capture semantic meaning. This means that similar images (in terms of content or style) will have similar embeddings, which is crucial for tasks like similarity search.
Once the embeddings are generated, they can be stored and queried within a vector database like ChromaDB.
Step 4: Inserting Embeddings into ChromaDB
After generating the embeddings, the next step is to store them in ChromaDB for fast access and retrieval. In this step, we initialize a ChromaDB client, create a collection to hold the embeddings, and then insert the embeddings along with any relevant metadata (such as the image ID or description).
Here’s how you can add your image embeddings to ChromaDB:
import chromadb # Initialize ChromaDB client client = chromadb.Client() # Create a collection to store the embeddings collection = client.create_collection('image_embeddings') # Insert embeddings along with their metadata (such as image ID or name) collection.add([embedding], metadatas=[{'image_id': '123'}])
In this code:
- Client: The
chromadb.Client()
initializes the connection to the ChromaDB database. - Collection: A collection in ChromaDB is a group of vectors (embeddings) that you can query against. By creating a collection called
image_embeddings
, we ensure that all image embeddings are stored together. - Add: The
add()
function inserts the embeddings into the collection, along with any metadata. Metadata can be anything that helps you identify or contextualize the embeddings, such as an image ID or a description.
Now that the embeddings are stored in ChromaDB, we can efficiently query them to find similar images.
Step 5: Querying ChromaDB
One of the main advantages of using ChromaDB is its ability to quickly retrieve embeddings that are similar to a given query embedding. For example, if you want to find images that are visually similar to a query image, you can generate its embedding and perform a similarity search.
Here’s how you can query the database for similar images:
# Query for similar images query_embedding = model.encode_image(query_image) results = collection.query(query_embedding, n_results=5) # Display the most similar images for result in results['documents']: print(result['image_id'])
In this code:
- Query Embedding: First, we generate the embedding for the query image, which is the image for which you want to find similar content.
- Query: The
query()
function in ChromaDB takes the query embedding and returns the most similar embeddings from the collection. - Results: The
results
object contains the closest matching embeddings. Here, we specifyn_results=5
, meaning we want to retrieve the 5 most similar images.
Applications of ChromaDB in Real-World Scenarios
Now that we understand how to load data, generate embeddings, and query ChromaDB, let’s explore some real-world applications of this technology:
1. Visual Search
In e-commerce, visual search allows users to upload an image of a product and find similar items. For example, if a customer uploads an image of a pair of shoes, the system can return similar shoes available for purchase on the platform.
ChromaDB makes this process seamless by storing embeddings of products and querying the most visually similar items in real-time.
2. Recommendation Systems
Recommendation systems use embeddings to suggest content that is related to what a user has interacted with in the past. By storing embeddings of items such as movies, books, or songs, ChromaDB enables recommendation systems to provide personalized suggestions based on a user's preferences.
3. Machine Learning Pipelines
In machine learning, embeddings are often used in downstream tasks such as clustering, classification, and semantic analysis. ChromaDB provides an efficient storage solution for embeddings, making it easier to build scalable machine learning pipelines.
Conclusion
ChromaDB is a powerful and efficient vector database that provides a simple yet robust way to store, retrieve, and query embeddings. Whether you're building a visual search system, recommendation engine, or integrating embeddings into a machine learning pipeline, ChromaDB offers the tools you need to manage high-dimensional data efficiently.
Through this guide, we’ve walked through the key steps of setting up, generating embeddings, and performing similarity searches with ChromaDB. Now that you have a better understanding of how to work with ChromaDB, you can start integrating it into your own projects and unlock the power of embeddings in your AI applications.
If you’re interested in leveraging ChromaDB for your own projects, make sure to explore its official documentation and experiment with different datasets and models!
Resources
---------------------------------
Stay Updated:- Follow Build Fast with AI pages for all the latest AI updates and resources.
Experts predict 2025 will be the defining year for Gen AI implementation.Want to be ahead of the curve?
Join Build Fast with AI’s Gen AI Launch Pad 2025 - your accelerated path to mastering AI tools and building revolutionary applications.