Building AI Agents with AgentLite: A Comprehensive Guide
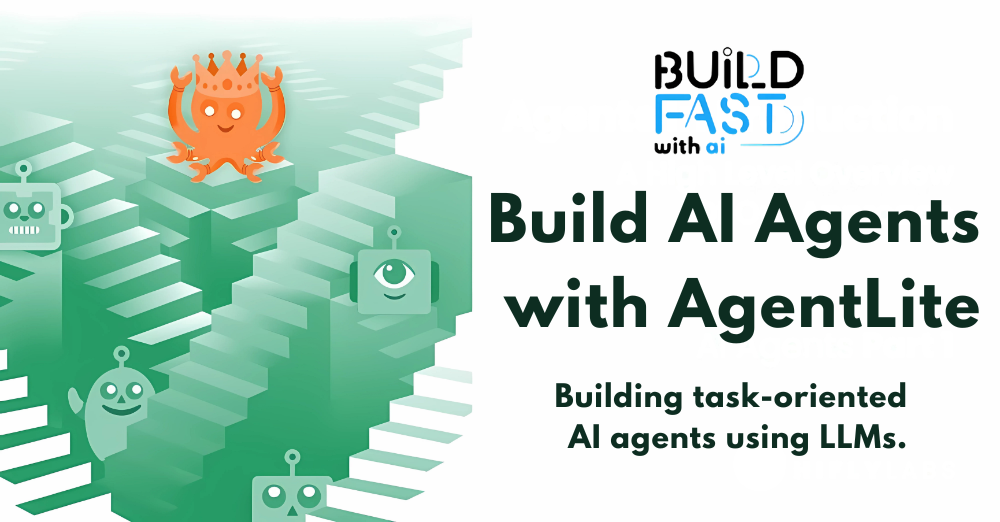
Are you waiting for change, or will you create it?
The future is calling—answer it with Gen AI Launch Pad 2025.
Introduction
AI agents are revolutionizing automation, task execution, and decision-making processes. AgentLite is an open-source framework designed to streamline the development of intelligent, task-oriented AI agents using large language models (LLMs). Whether you want to build a simple single-agent system or a sophisticated multi-agent architecture, AgentLite provides an easy-to-use and highly customizable solution.
In this guide, we will:
- Walk through AgentLite’s key features and setup process.
- Build a Hello World agent to understand the framework.
- Develop an AI-powered chess-playing agent that interacts with a chessboard using AgentLite.
- Explore the practical applications of AI agents in various domains.
By the end of this tutorial, you’ll be equipped to create your own custom AI agents for real-world applications!
🔹 Key Features of AgentLite
- ✅ Lightweight & Easy-to-Use: Simple syntax and intuitive structure make agent creation seamless.
- ✅ Supports Multi-Agent Collaboration: Build networks of AI agents that communicate and solve tasks together.
- ✅ Highly Customizable: Tailor agents for applications such as automation, gaming, and customer service.
🚀 Setup & Installation
Before we start coding, install the required dependencies:
!pip install agentlite-llm chess !pip install --upgrade langchain langchain-openai
We also need to set up an OpenAI API Key for accessing the language model:
from google.colab import userdata OPENAI_API_KEY = userdata.get("OPENAI_API_KEY")
Why Use AgentLite? AgentLite simplifies the process of developing AI agents that interact with LLMs. By integrating with frameworks like LangChain, it provides a structured approach to agent design.
Creating a "Hello World" Agent
To understand AgentLite’s core workflow, let’s create a simple agent that responds with “Hello World!” when given any instruction.
Defining the Agent
from agentlite.agents import BaseAgent from agentlite.llm.LLMConfig import LLMConfig from agentlite.llm.agent_llms import LangchainChatModel from agentlite.commons import TaskPackage class HelloWorldAgent(BaseAgent): def __init__(self, llm): super().__init__(name="HelloWorld", role="Responds with Hello World!", llm=llm) def respond(self, task, **kwargs): return "Hello World!" llm_config = LLMConfig({"llm_name": "gpt-3.5-turbo", "temperature": 0.0, "api_key": OPENAI_API_KEY}) llm = LangchainChatModel(llm_config) hello_agent = HelloWorldAgent(llm=llm)
Executing the Agent
task = TaskPackage(instruction="Any instruction here") response = hello_agent(task) print(response)
✅ Expected Output:
Hello World!
📌 Explanation:
- BaseAgent is the parent class that all AI agents inherit from.
- respond() is the agent’s function that generates a reply.
- The TaskPackage object contains the input instruction.
- The agent simply returns "Hello World!" for any given input.
Integrating Chess with AgentLite
Next, let’s take things further by building an AI agent that plays chess!
Implementing a Chess Move Action
We first define an action that allows the agent to make a move on a chessboard.
import chess import chess.svg from agentlite.actions import BaseAction from IPython.display import display, HTML BOARD = chess.Board() class BoardMove(BaseAction): def __init__(self) -> None: super().__init__( action_name="BoardMove", action_desc="Move a piece on the chessboard", params_doc={"query": "Standard Universal Chess Interface Move"}, ) def __call__(self, query): try: BOARD.push_uci(query) except ValueError as e: return f"Error: {e}. Illegal move." m = chess.Move.from_uci(query) svg_code = chess.svg.board(BOARD, arrows=[(m.from_square, m.to_square)], fill={m.from_square: "gray"}, size=200) display(HTML(svg_code)) return f"Moved: {query}."
👀 Viewing Board State
class BoardView(BaseAction): def __init__(self) -> None: super().__init__( action_name="BoardView", action_desc="Get the current board status in FEN format", params_doc={"key": "True"}, ) def __call__(self, key, **kwargs): return BOARD.fen()
✅ Expected Output:
rnbqkbnr/pppppppp/8/8/8/8/PPPPPPPP/RNBQKBNR w KQkq - 0 1
(This represents the initial chessboard state.)
🎭 Creating a Chess Player Agent
Now, we define an AI-powered chess player that interacts with the board.
from agentlite.agents import BaseAgent from agentlite.llm.agent_llms import BaseLLM from agentlite.actions import LegalMoves from agentlite.logging import AgentLogger class ChessPlayerAgent(BaseAgent): def __init__(self, llm: BaseLLM, color: str): if color not in ["white", "black"]: raise ValueError("color must be either white or black") name = f"{color}_player" role = f"You are {name}, playing against the opponent." super().__init__(name=name, role=role, llm=llm, actions=[BoardMove(), BoardView(), LegalMoves()])
🔄 Running the Chess Game
player_white = ChessPlayerAgent(llm=llm, color="white") player_black = ChessPlayerAgent(llm=llm, color="black") exp_task = TaskPackage(instruction="It is your turn") player_white(exp_task) player_black(exp_task)
Expected Chess Move Output:
Chess Moved. It's black_player's turn. Chess Moved. It's white_player's turn.
Conclusion
In this guide, we explored AgentLite, built a Hello World agent, and created an AI-powered chess player capable of interacting with a chessboard. AI agents like these can be applied to gaming, automation, and task delegation in various industries.
Further Resources
---------------------------
Stay Updated:- Follow Build Fast with AI pages for all the latest AI updates and resources.
Experts predict 2025 will be the defining year for Gen AI Implementation. Want to be ahead of the curve?
Join Build Fast with AI’s Gen AI Launch Pad 2025 - your accelerated path to mastering AI tools and building revolutionary applications.
---------------------------
Resources and Community
Join our community of 12,000+ AI enthusiasts and learn to build powerful AI applications! Whether you're a beginner or an experienced developer, this tutorial will help you understand and implement AI agents in your projects.
- Website: www.buildfastwithai.com
- LinkedIn: linkedin.com/company/build-fast-with-ai/
- Instagram: instagram.com/buildfastwithai/
- Twitter: x.com/satvikps
- Telegram: t.me/BuildFastWithAI