Atomic Agents: Modular AI for Scalable Applications
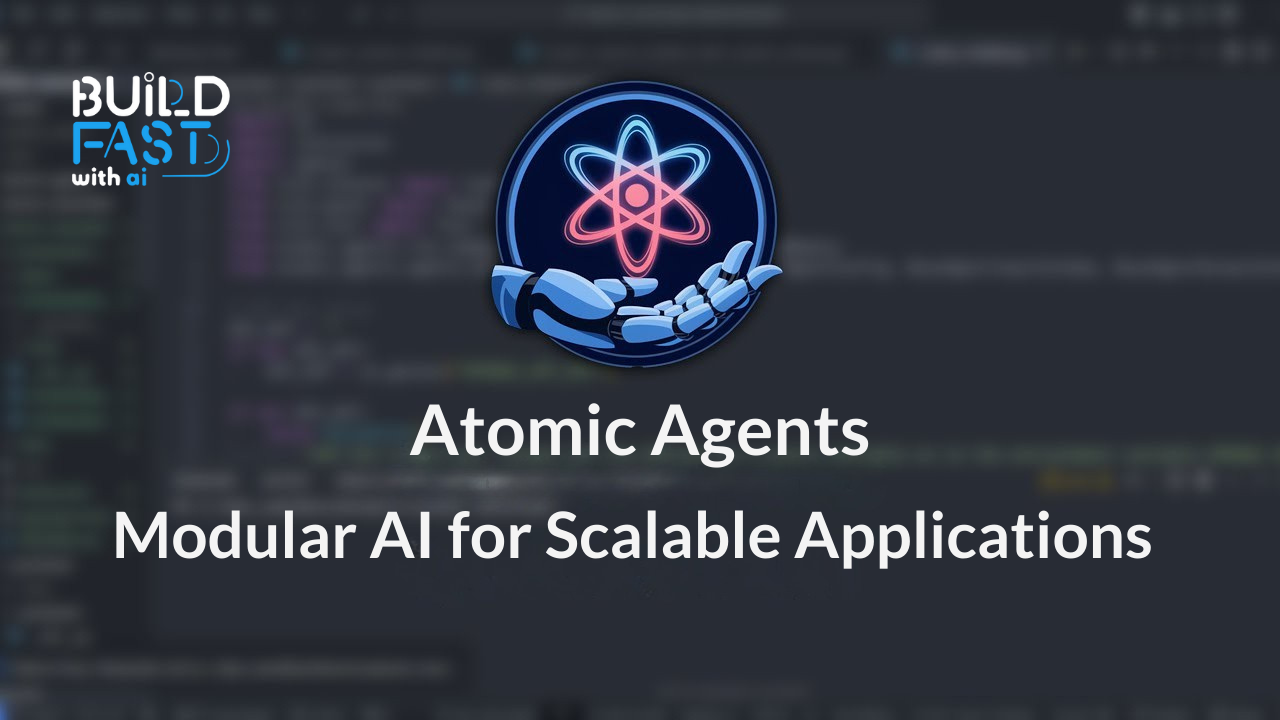
Will you regret not taking action, or be proud of what you’ve built?
Gen AI Launch Pad 2025 awaits your vision.
Introduction
Artificial Intelligence (AI) is rapidly transforming industries, but ensuring control, predictability, and extensibility in AI systems remains a challenge. Atomic Agents is a modular AI framework designed to address these concerns by enabling developers to create predictable, controllable, and reusable AI components. This blog will provide a comprehensive guide to Atomic Agents, explaining its core principles, installation process, agent configuration, and real-world applications. We will analyze the code provided in the Jupyter notebook, detailing each segment to ensure a deep understanding of the framework's functionality.
Why Choose Atomic Agents?
Atomic Agents is built to offer:
- Modularity: Develop AI applications using small, reusable components.
- Predictability: Maintain consistency in AI behavior with clear input/output schemas.
- Extensibility: Easily integrate new components without affecting existing ones.
- Control: Fine-tune each part of the system, from prompts to integrations.
Unlike traditional multi-agent frameworks that may introduce unpredictability, Atomic Agents provides structured outputs tailored for business and technical needs.
Installation and Setup
To get started with Atomic Agents, install the framework using:
pip install atomic-agents
This command installs the necessary dependencies, enabling you to create and manage AI agents efficiently.
AI Agent Initialization
The first step in using Atomic Agents is initializing an AI agent. Below is the code snippet to set up an agent with memory and OpenAI integration.
Code:
import os import instructor import openai from rich.console import Console from rich.panel import Panel from rich.text import Text from atomic_agents.lib.components.agent_memory import AgentMemory from atomic_agents.agents.base_agent import BaseAgent, BaseAgentConfig, BaseAgentInputSchema, BaseAgentOutputSchema from google.colab import userdata API_KEY = userdata.get("OPENAI_API_KEY") console = Console() memory = AgentMemory() initial_message = BaseAgentOutputSchema(chat_message="Hello! How can I assist you today?") memory.add_message("assistant", initial_message) client = instructor.from_openai(openai.OpenAI(api_key=API_KEY)) agent = BaseAgent( config=BaseAgentConfig( client=client, model="gpt-4o-mini", memory=memory, ) )
Explanation:
- Agent Memory: The
AgentMemory
object stores conversation history and contextual data. - BaseAgentConfig: Defines the agent’s configuration, including the AI model and memory.
- BaseAgent: Creates an instance of the AI assistant.
- Rich Library: Used for formatted console output.
- Google Colab's userdata: Retrieves API keys securely for authentication.
Expected Output:
Agent: Hello! How can I assist you today?
This confirms that the agent has been successfully initialized and is ready for interaction.
Interactive Chat Loop
The following code creates a loop to allow real-time user interaction with the agent.
Code:
default_system_prompt = agent.system_prompt_generator.generate_prompt() console.print(Panel(default_system_prompt, width=console.width, style="bold cyan"), style="bold cyan") console.print(Text("Agent:", style="bold green"), end=" ") console.print(Text(initial_message.chat_message, style="bold green")) while True: user_input = console.input("[bold blue]You:[/bold blue] ") if user_input.lower() in ["/exit", "/quit"]: console.print("Exiting chat...") break input_schema = BaseAgentInputSchema(chat_message=user_input) response = agent.run(input_schema) agent_message = Text(response.chat_message, style="bold green") console.print(Text("Agent:", style="bold green"), end=" ") console.print(agent_message)
Explanation:
- The chat loop continuously accepts user input.
- If the user types
/exit
or/quit
, the loop terminates. - The agent processes the input and responds accordingly.
BaseAgentInputSchema
structures user messages before being passed to the AI.- The Rich library ensures formatted output in the console.
Expected Output:
You: What is Python? Agent: Python is a high-level, interpreted programming language known for its readability and simplicity...
This interactive loop ensures that the AI can dynamically respond to user queries.
Multimodal Nutrition Label Analysis
Atomic Agents can also handle multimodal tasks, such as analyzing nutrition labels from images. The following section details how this is implemented.
Nutrition Label Data Schema
from atomic_agents.lib.base.base_io_schema import BaseIOSchema from pydantic import Field from typing import List class NutritionLabel(BaseIOSchema): calories: int = Field(..., description="Calories per serving") total_fat: float = Field(..., description="Total fat in grams") ...
Explanation:
- The
NutritionLabel
class defines the schema for extracting nutritional values. - Pydantic ensures that input values are validated and properly formatted.
- Each field represents an essential component of a nutrition label.
Expected Output:
The extracted nutritional information will be formatted in JSON structure:
{ "product_name": "Pretzels", "serving_size": "3 pretzels (28g)", "calories": 110, "total_fat": 0.5, "sodium": 400, "total_carbohydrates": 23.0, "protein": 2.0 }
This structured output makes it easy to analyze and store nutritional information programmatically.
Image Processing and Nutrition Analysis
To analyze nutrition labels, the system must first download images and process them.
def download_image(url, save_path): response = requests.get(url) with open(save_path, "wb") as file: file.write(response.content)
Explanation:
requests.get(url)
: Fetches the image from the internet.- The file is written in binary mode to maintain image integrity.
This function is used to retrieve nutrition label images before analysis.
Conclusion
Atomic Agents provides a structured, modular approach to AI development, ensuring predictability and control. Through its extensible architecture, it allows developers to create AI applications that seamlessly integrate with real-world use cases. From interactive chat assistants to complex multimodal analyses like nutrition label extraction, Atomic Agents showcases its versatility in AI-driven applications.
Resources
---------------------------
Stay Updated:- Follow Build Fast with AI pages for all the latest AI updates and resources.
Experts predict 2025 will be the defining year for Gen AI Implementation. Want to be ahead of the curve?
Join Build Fast with AI’s Gen AI Launch Pad 2025 - your accelerated path to mastering AI tools and building revolutionary applications.
---------------------------
Resources and Community
Join our community of 12,000+ AI enthusiasts and learn to build powerful AI applications! Whether you're a beginner or an experienced developer, this tutorial will help you understand and implement AI agents in your projects.
- Website: www.buildfastwithai.com
- LinkedIn: linkedin.com/company/build-fast-with-ai/
- Instagram: instagram.com/buildfastwithai/
- Twitter: x.com/satvikps
- Telegram: t.me/BuildFastWithAI